Pandas DataFrame DataFrame.assign()関数
Suraj Joshi
2023年1月30日
Pandas
Pandas DataFrame
-
pandas.DataFrame.assign()
の構文: -
コード例:単一の列を割り当てる
DataFrame.assign()
メソッド -
コード例:複数の列を割り当てるための
DataFrame.assign()
メソッド
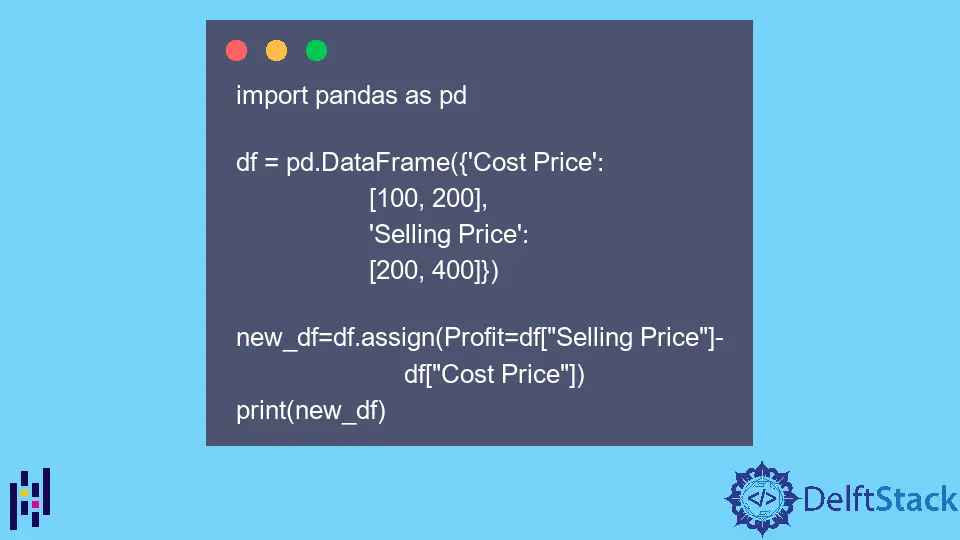
Python Pandas DataFrame.assign()
関数は、新しい列を DataFrame
に割り当てます。
pandas.DataFrame.assign()
の構文:
DataFrame.assign(**kwargs)
パラメーター
**kwargs |
assign() 関数のキーワード引数。DataFrame に割り当てられる列名は、キーワード引数として渡されます。 |
戻り値
既存の列とともに新しい列が割り当てられた DataFrame
オブジェクトを返します。
コード例:単一の列を割り当てる DataFrame.assign()
メソッド
import pandas as pd
df = pd.DataFrame({'Cost Price':
[100, 200],
'Selling Price':
[200, 400]})
new_df=df.assign(Profit=df["Selling Price"]-
df["Cost Price"])
print(new_df)
呼び出し元の DataFrame
は
Cost Price Selling Price
0 100 200
1 200 400
出力:
Cost Price Selling Price Profit
0 100 200 100
1 200 400 200
新しい列 Profit
を Dataframe
に割り当てます。これは Selling Price
列と Cost Price
列の違いに対応しています。
また、呼び出し可能なオブジェクトに対して lambda
関数を使用して、df
に新しい列を割り当てることもできます。
import pandas as pd
df = pd.DataFrame({'Cost_Price':
[100, 200],
'Selling_Price':
[200, 400]})
new_df=df.assign(Profit=lambda x:
x.Selling_Price-
x.Cost_Price)
print(new_df)
呼び出し元の DataFrame
は
Cost Price Selling Price
0 100 200
1 200 400
出力:
Cost_Price Selling_Price Profit
0 100 200 100
1 200 400 200
コード例:複数の列を割り当てるための DataFrame.assign()
メソッド
import pandas as pd
df = pd.DataFrame({'Cost_Price':
[100, 200],
'Selling_Price':
[200, 400]})
new_df=df.assign(Cost_Price_Euro =
df['Cost_Price']*1.11,
Selling_Price_Euro =
df['Selling_Price']*1.11)
print(new_df)
呼び出し元の DataFrame
は
Cost Price Selling Price
0 100 200
1 200 400
出力:
Cost_Price Selling_Price Cost_Price_Euro Selling_Price_Euro
0 100 200 111.0 222.0
1 200 400 222.0 444.0
2つの新しい列 Cost_Price_Euro
と Selling_Price_Euro
を df
に割り当てます。これらは既存の列 Cost_Price
と Selling_Price
からそれぞれ派生します。
呼び出し可能なオブジェクトに対して lambda
関数を使用して df
に複数の列を割り当てることもできます。
import pandas as pd
df = pd.DataFrame({'Cost_Price':
[100, 200],
'Selling_Price':
[200, 400]})
new_df=df.assign(Cost_Price_Euro =
lambda x: x.Cost_Price*1.11,
Selling_Price_Euro =
lambda x: x.Selling_Price*1.11)
print(new_df)
呼び出し元の DataFrame
は
Cost Price Selling Price
0 100 200
1 200 400
出力:
Cost_Price Selling_Price Cost_Price_Euro Selling_Price_Euro
0 100 200 111.0 222.0
1 200 400 222.0 444.0
チュートリアルを楽しんでいますか? <a href="https://www.youtube.com/@delftstack/?sub_confirmation=1" style="color: #a94442; font-weight: bold; text-decoration: underline;">DelftStackをチャンネル登録</a> して、高品質な動画ガイドをさらに制作するためのサポートをお願いします。 Subscribe
著者: Suraj Joshi
Suraj Joshi is a backend software engineer at Matrice.ai.
LinkedIn