How to Format Number With Commas in Python
-
Use
format()
Function to Format Number With Commas in Python -
Use the
str.format()
Method to Format Number With Commas in Python - Use F-Strings to Format Number With Commas in Python
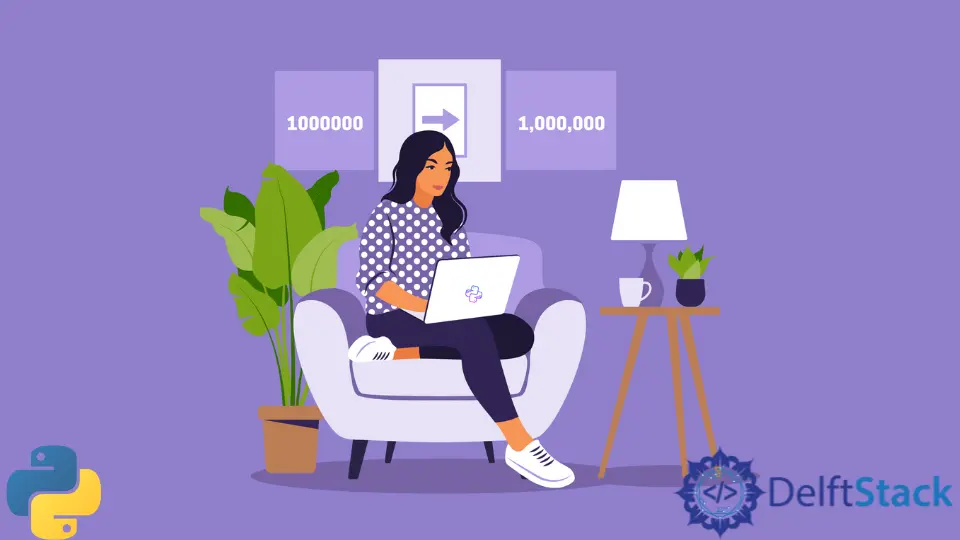
Whenever there are a lot of numbers in one program, inserting commas in between each number as a thousand separators always helps in reading the number correctly without looking at each number carefully. Generally, this task is done when we deal with big numbers with decimal points, currencies, etc.
This tutorial will demonstrate different ways of formatting a number with commas in Python.
Use format()
Function to Format Number With Commas in Python
The format()
is a built-in function that generally helps in string handling. This function also helps in changing complex variables and handles value formatting.
Example:
initial_num = 1000000000
thousand_sep = format(initial_num, ",d")
print("Number before inserting commas : " + str(initial_num))
print("Number after inserting commas: " + str(thousand_sep))
Output:
Number before inserting commas : 1000000000
Number after inserting commas: 1,000,000,000
In this method, we first store a number in which we have to insert commas in a variable. Then we use the format()
function by first mentioning the variable name and then the format specifier as the function argument. Here, the format specifier is ,d
, representing that a decimal value is stored as an initial value. Finally, the str()
function returns the initial and final value as a string.
Use the str.format()
Method to Format Number With Commas in Python
This method works based on the string formatter. String formatters are represented by curly braces {}
that work by mentioning the replacement parameters and the place of those parameters.
Example:
def thousand_sep(num):
return "{:,}".format(num)
print(thousand_sep(1000000000))
Output:
1,000,000,000
In this method, we first define a function called thousand_sep
with its argument as the number in which commas are inserted. After that, we call the str.format()
with the string as the string formatter. In the string formatter, we mention the replacement parameter i.e ,
. Finally, we print the defined function.
Use F-Strings to Format Number With Commas in Python
F-strings is again a string formatting technique in Python. This method is the simplest way to add expressions inside python strings. It is used by prefixing the whole string with the letter f
.
Example:
initial_num = 1000000000
f"{initial_num:,}"
Output:
'1,000,000,000'
In this method, the expression to be added and the string to be formatted are stored after prefixing the letter f
. Also, note that the output is returned as a string.
Lakshay Kapoor is a final year B.Tech Computer Science student at Amity University Noida. He is familiar with programming languages and their real-world applications (Python/R/C++). Deeply interested in the area of Data Sciences and Machine Learning.
LinkedIn