How to Change the Order of Pandas DataFrame Columns
Asad Riaz
Feb 02, 2024
Pandas
Pandas DataFrame
Pandas DataFrame Column
- List Columns in the Newly Desired Order in Pandas
-
insert
New Column With the Specific Location in Pandas -
reindex
Column for Given Order in Pandas
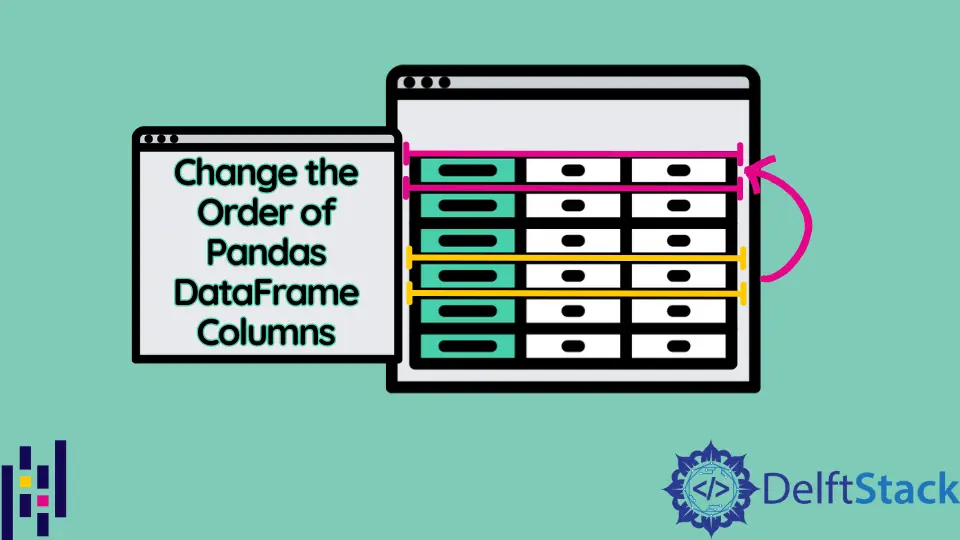
We will introduce how to change the order of DataFrame
columns, with different methods like assigning the column names in the order we want, by using insert
and reindex
.
List Columns in the Newly Desired Order in Pandas
The simplest way is to reassign the DataFrame
with a list of the columns
, or by just assign the column names in the order we want them:
# python 3.x
import pandas as pd
df = pd.DataFrame(
{
"a": ["1", "2", "3", "4"],
"b": [16, 7, 6, 16],
"c": [61, 57, 16, 36],
"d": ["12", "22", "13", "44"],
"e": ["Green", "Red", "Blue", "Yellow"],
"f": [1, 11, 23, 66],
}
)
print(df)
df = df[["e", "c", "b", "f", "d", "a"]]
print("Rearranging ..................")
print("..............................")
print(df)
Output:
a b c d e f
0 1 16 61 12 Green 1
1 2 7 57 22 Red 11
2 3 6 16 13 Blue 23
3 4 16 36 44 Yellow 66
Rearranging ..................
..............................
e c b f d a
0 Green 61 16 1 12 1
1 Red 57 7 11 22 2
2 Blue 16 6 23 13 3
3 Yellow 36 16 66 44 4
insert
New Column With the Specific Location in Pandas
If we are creating a new column we can insert it any location we want:
# python 3.x
import pandas as pd
df = pd.DataFrame(
{
"a": ["1", "2", "3", "4"],
"b": [16, 7, 6, 16],
"c": [61, 57, 16, 36],
"d": ["12", "22", "13", "44"],
"e": ["Green", "Red", "Blue", "Yellow"],
"f": [1, 11, 23, 66],
}
)
print(df)
print("Inserting ..................")
print("..............................")
df.insert(0, "newColMean", df.mean(1))
print(df)
Output:
newColMean a b c d e f
0 26.000000 1 16 61 12 Green 1
1 25.000000 2 7 57 22 Red 11
2 15.000000 3 6 16 13 Blue 23
3 39.333333 4 16 36 44 Yellow 66
reindex
Column for Given Order in Pandas
reindex
is arguably the most efficient way to rearrange the column:
# python 3.x
import pandas as pd
df = pd.DataFrame(
{
"a": ["1", "2", "3", "4"],
"b": [16, 7, 6, 16],
"c": [61, 57, 16, 36],
"d": ["12", "22", "13", "44"],
"e": ["Green", "Red", "Blue", "Yellow"],
"f": [1, 11, 23, 66],
}
)
print(df)
print("Rearranging ..................")
print("..............................")
df = df.reindex(columns=["a", "f", "d", "b", "c", "e"])
print(df)
Output:
a b c d e f
0 1 16 61 12 Green 1
1 2 7 57 22 Red 11
2 3 6 16 13 Blue 23
3 4 16 36 44 Yellow 66
Rearranging ..................
..............................
a f d b c e
0 1 1 12 16 61 Green
1 2 11 22 7 57 Red
2 3 23 13 6 16 Blue
3 4 66 44 16 36 Yellow
Enjoying our tutorials? Subscribe to DelftStack on YouTube to support us in creating more high-quality video guides. Subscribe
Related Article - Pandas DataFrame
- How to Get Pandas DataFrame Column Headers as a List
- How to Delete Pandas DataFrame Column
- How to Convert Pandas Column to Datetime
- How to Convert a Float to an Integer in Pandas DataFrame
- How to Sort Pandas DataFrame by One Column's Values
- How to Get the Aggregate of Pandas Group-By and Sum