How to Convert a Float to an Integer in Pandas DataFrame
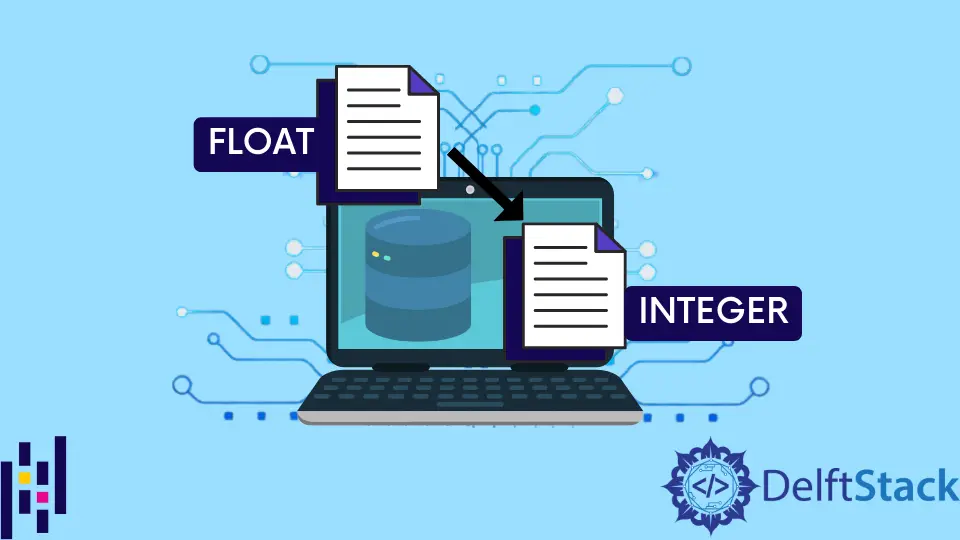
We will demonstrate methods to convert a float to an integer in a Pandas DataFrame
- astype(int)
and to_numeric()
methods.
First, we create a random array using the NumPy
library and then convert it into DataFrame
.
import pandas as pd
import numpy as np
df = pd.DataFrame(np.random.rand(5, 5) * 5)
print(df)
If you run this code, you will get the output as following which has values of float
type.
0 1 2 3 4
00.3024483.5519583.8786602.3803524.741592
14.0541870.9409520.4590584.3148010.524993
22.8917334.9268854.9557732.6263734.144166
31.1276393.1968234.1440201.3506320.401138
41.4235372.0194553.0389450.4366573.823888
astype(int)
to Convert float
to int
in Pandas
To convert float
into int
we could use the Pandas DataFrame.astype(int)
method. The code is,
import pandas as pd
import numpy as np
df = pd.DataFrame(np.random.rand(5, 5) * 5)
print("*********** Random Float DataFrame ************")
print(df)
print("***********************************************")
print("***********************************************")
print("*********** Dataframe Converted into INT ************")
print(df.astype(int))
print("***********************************************")
print("***********************************************")
After running the above codes, we will get the following output.
*********** Random Float DataFrame ************
0 1 2 3 4
0 3.629665 2.552326 3.995622 3.155777 4.715785
1 1.597920 2.090324 0.511498 1.936061 1.286486
2 1.051634 3.550839 2.468125 4.213684 2.329477
3 3.443836 2.749433 1.560226 3.037708 2.966934
4 4.686530 2.096314 4.028526 4.253299 1.175233
***********************************************
***********************************************
*********** Dataframe Converted into INT ************
0 1 2 3 4
0 3 2 3 3 4
1 1 2 0 1 1
2 1 3 2 4 2
3 3 2 1 3 2
4 4 2 4 4 1
***********************************************
***********************************************
It converts all the Pandas DataFrame
columns to int
.
Convert Pandas DataFrame Column to int
With Rounding Off
We can round off the float
value to int
by using df.round(0).astype(int)
.
import pandas as pd
import numpy as np
df = pd.DataFrame(np.random.rand(5, 5) * 5)
print("*********** Random Float DataFrame ************")
print(df)
print("***********************************************")
print("***********************************************")
print("*********** Dataframe Converted into INT ************")
print(df.astype(int))
print("***********************************************")
print("***********************************************")
print("*********** Rounding Float value to INT ************")
print(df.round(0).astype(int))
print("***********************************************")
print("***********************************************")
After running the codes, we will get the following output.
*********** Random Float DataFrame ************
0 1 2 3 4
0 4.888469 3.815177 3.993451 0.901108 1.958223
1 1.009212 1.532287 2.689159 1.008482 1.394623
2 4.152496 0.265261 4.667446 4.412533 4.797394
3 0.039770 1.526753 3.778224 0.073072 0.509409
4 1.292580 3.581438 0.759043 3.872206 2.591886
***********************************************
***********************************************
*********** Dataframe Converted into INT ************
0 1 2 3 4
0 4 3 3 0 1
1 1 1 2 1 1
2 4 0 4 4 4
3 0 1 3 0 0
4 1 3 0 3 2
***********************************************
***********************************************
*********** Rounding Float value to INT ************
0 1 2 3 4
0 5 4 4 1 2
1 1 2 3 1 1
2 4 0 5 4 5
3 0 2 4 0 1
4 1 4 1 4 3
***********************************************
***********************************************
The df.astype(int)
converts Pandas float
to int
by negelecting all the floating point digits.
df.round(0).astype(int)
rounds the Pandas float
number closer to zero.
to_numeric()
Method to Convert float
to int
in Pandas
This method provides functionality to safely convert non-numeric types (e.g. strings) to a suitable numeric type.
s = pd.Series(["1.0", "2", -3])
print(pd.to_numeric(s, downcast="integer"))
After running the codes, we will get the following output.
01
12
2 -3
dtype: int8