Pandas DataFrame.astype() Function
-
Syntax of
pandas.DataFrame.astype()
: -
Example Codes:
DataFrame.astype()
Method to Change Data Type of One Column -
Example Codes:
DataFrame.astype()
Method to Change the Data Type of All Columns of Data Frame -
Example Codes:
DataFrame.astype()
Method to Change the Data Type With Exception
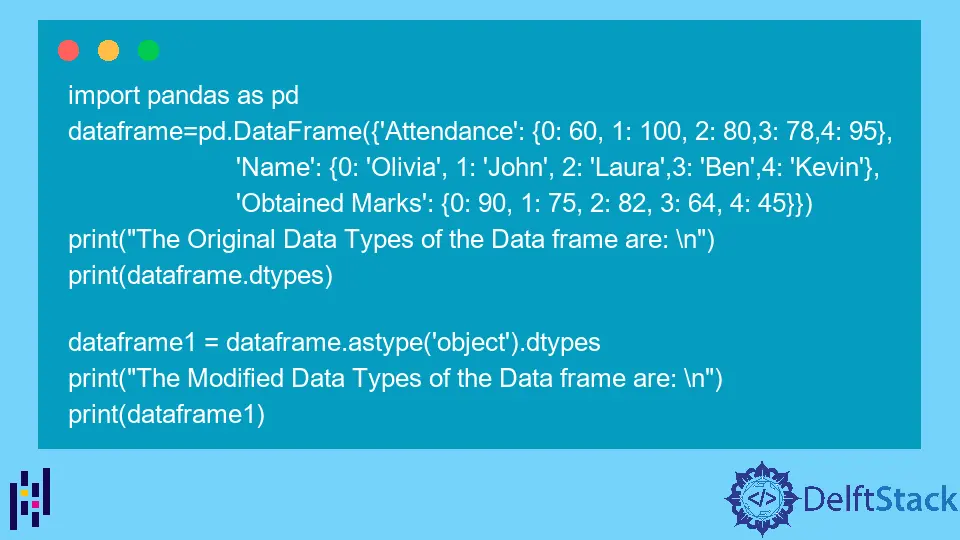
Python Pandas DataFrame.astype()
function changes the data type of the objects to a specified data type.
Syntax of pandas.DataFrame.astype()
:
DataFrame.astype(dtype, copy=True, errors="raise")
Parameters
dtype |
Data type that we want to assign to our object. |
copy |
A Boolean parameter. It returns a copy when True . |
errors |
It controls the raising of exceptions on invalid data for the provided data type. It has two options. raise : allows exceptions to be raised. ignore : suppresses exceptions. If an error exists, then it returns the original object. |
Return
It returns the DataFrame with the casted data types.
Example Codes: DataFrame.astype()
Method to Change Data Type of One Column
import pandas as pd
dataframe=pd.DataFrame({'Attendance': {0: 60, 1: 100, 2: 80,3: 78,4: 95},
'Name': {0: 'Olivia', 1: 'John', 2: 'Laura',3: 'Ben',4: 'Kevin'},
'Obtained Marks': {0: 90, 1: 75, 2: 82, 3: 64, 4: 45}})
print("The Original Data Types of the Data frame are: \n")
print(dataframe.dtypes)
dataframe1 = dataframe.astype({'Attendance': 'int32'}).dtypes
print("The Modified Data Types of the Data frame are: \n")
print(dataframe1)
Output:
The Original Data Types of the Data frame are:
Attendance int64
Name object
Obtained Marks int64
dtype: object
The Modified Data Types of the Data frame are:
Attendance int32
Name object
Obtained Marks int64
dtype: object
The function has returned the casted data type. We have used dtypes()
function to show the data types of the columns of the DataFrame.
Example Codes: DataFrame.astype()
Method to Change the Data Type of All Columns of Data Frame
We will try to change the data type of the given DataFrame.
import pandas as pd
dataframe=pd.DataFrame({'Attendance': {0: 60, 1: 100, 2: 80,3: 78,4: 95},
'Name': {0: 'Olivia', 1: 'John', 2: 'Laura',3: 'Ben',4: 'Kevin'},
'Obtained Marks': {0: 90, 1: 75, 2: 82, 3: 64, 4: 45}})
print("The Original Data Types of the Data frame are: \n")
print(dataframe.dtypes)
dataframe1 = dataframe.astype('object').dtypes
print("The Modified Data Types of the Data frame are: \n")
print(dataframe1)
Output:
The Original Data Types of the Data frame are:
Attendance int64
Name object
Obtained Marks int64
dtype: object
The Modified Data Types of the Data frame are:
Attendance object
Name object
Obtained Marks object
dtype: object
The function has returned the modified DataFrame. It has changed the data type of all columns to object
.
Example Codes: DataFrame.astype()
Method to Change the Data Type With Exception
Now we will set the data type object
to int32
. The function will ignore the exception as we will pass the parameter errors= 'ignore'
.
import pandas as pd
dataframe=pd.DataFrame({'Attendance': {0: 60, 1: 100, 2: 80,3: 78,4: 95},
'Name': {0: 'Olivia', 1: 'John', 2: 'Laura',3: 'Ben',4: 'Kevin'},
'Obtained Marks': {0: 90, 1: 75, 2: 82, 3: 64, 4: 45}})
print("The Original Data Types of the Data frame are: \n")
print(dataframe.dtypes)
dataframe1 = dataframe.astype('int32', errors='ignore').dtypes
print("The Modified Data Types of the Data frame are: \n")
print(dataframe1)
Output:
The Original Data Types of the Data frame are:
Attendance int64
Name object
Obtained Marks int64
dtype: object
The Modified Data Types of the Data frame are:
Attendance int32
Name object
Obtained Marks int32
dtype: object
Note that the function has not raised any exceptions. It has ignored the error as we were casting the object
to int32
. It merely has not changed the data type of the Name
column.