如何更改 Panas DataFrame 列的顺序
Asad Riaz
2023年1月30日
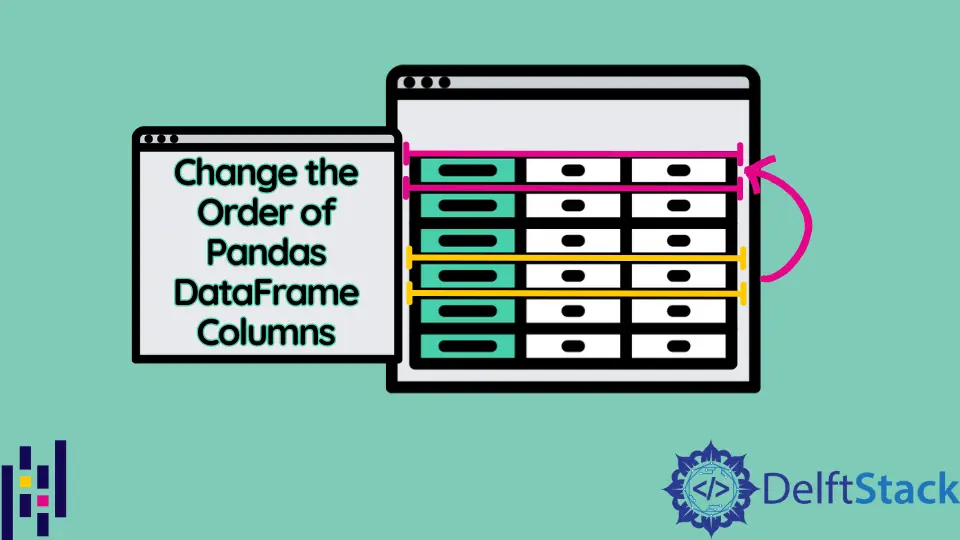
我们将介绍如何使用 insert
和 reindex
以不同的方法更改 pandas.DataFrame
列的顺序,例如以所需的顺序分配列名。
以新顺序在对 pandas.DataFrame
列排序
最简单的方法是用 columns
的列表重新分配 DataFrame
,或者只是按照我们想要的顺序分配列名:
# python 3.x
import pandas as pd
df = pd.DataFrame(
{
"a": ["1", "2", "3", "4"],
"b": [16, 7, 6, 16],
"c": [61, 57, 16, 36],
"d": ["12", "22", "13", "44"],
"e": ["Green", "Red", "Blue", "Yellow"],
"f": [1, 11, 23, 66],
}
)
print(df)
df = df[["e", "c", "b", "f", "d", "a"]]
print("Rearranging ..................")
print("..............................")
print(df)
输出:
a b c d e f
0 1 16 61 12 Green 1
1 2 7 57 22 Red 11
2 3 6 16 13 Blue 23
3 4 16 36 44 Yellow 66
Rearranging ..................
..............................
e c b f d a
0 Green 61 16 1 12 1
1 Red 57 7 11 22 2
2 Blue 16 6 23 13 3
3 Yellow 36 16 66 44 4
在 Pandas 中用 insert
添加新列和位置
如果我们要创建新列,则可以将其插入到我们想要的任何位置:
# python 3.x
import pandas as pd
df = pd.DataFrame(
{
"a": ["1", "2", "3", "4"],
"b": [16, 7, 6, 16],
"c": [61, 57, 16, 36],
"d": ["12", "22", "13", "44"],
"e": ["Green", "Red", "Blue", "Yellow"],
"f": [1, 11, 23, 66],
}
)
print(df)
print("Inserting ..................")
print("..............................")
df.insert(0, "newColMean", df.mean(1))
print(df)
输出:
newColMean a b c d e f
0 26.000000 1 16 61 12 Green 1
1 25.000000 2 7 57 22 Red 11
2 15.000000 3 6 16 13 Blue 23
3 39.333333 4 16 36 44 Yellow 66
Pandas 中给定顺序的 reindex
列
reindex
可以说是重新排列列的最有效方法:
# python 3.x
import pandas as pd
df = pd.DataFrame(
{
"a": ["1", "2", "3", "4"],
"b": [16, 7, 6, 16],
"c": [61, 57, 16, 36],
"d": ["12", "22", "13", "44"],
"e": ["Green", "Red", "Blue", "Yellow"],
"f": [1, 11, 23, 66],
}
)
print(df)
print("Rearranging ..................")
print("..............................")
df = df.reindex(columns=["a", "f", "d", "b", "c", "e"])
print(df)
输出:
a b c d e f
0 1 16 61 12 Green 1
1 2 7 57 22 Red 11
2 3 6 16 13 Blue 23
3 4 16 36 44 Yellow 66
Rearranging ..................
..............................
a f d b c e
0 1 1 12 16 61 Green
1 2 11 22 7 57 Red
2 3 23 13 6 16 Blue
3 4 66 44 16 36 Yellow
相关文章 - Pandas DataFrame
- 如何将 Pandas DataFrame 列标题获取为列表
- 如何删除 Pandas DataFrame 列
- 如何在 Pandas 中将 DataFrame 列转换为日期时间
- 如何在 Pandas DataFrame 中将浮点数转换为整数
- 如何按一列的值对 Pandas DataFrame 进行排序
- 如何用 group-by 和 sum 获得 Pandas 总和