JavaScript Unique Array
-
Get the Unique Values From an Array Using the
Set()
Function in JavaScript -
Get the Unique Values From an Array Using the
indexOf()
Function in JavaScript -
Get the Unique Values From an Array Using the
filter()
Function in JavaScript
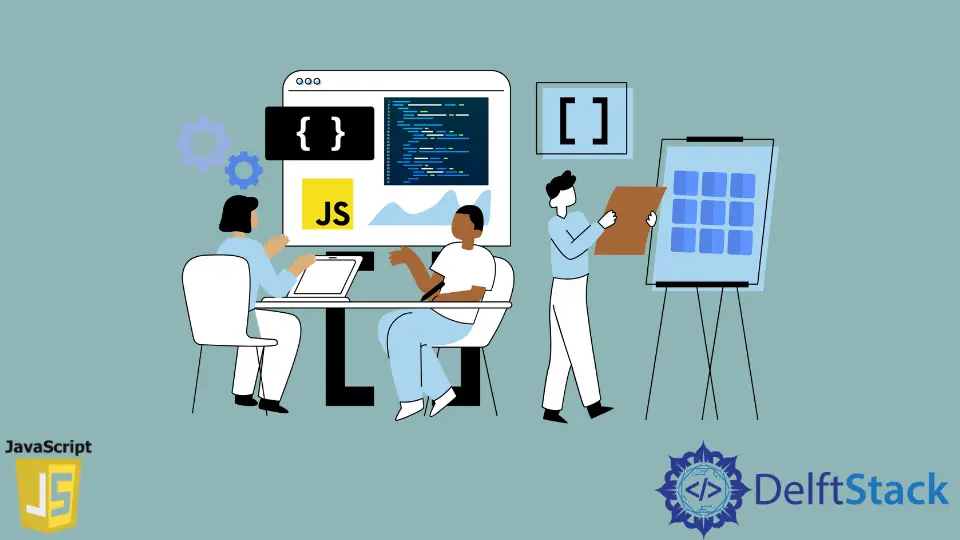
This tutorial will discuss how to get the unique values from an array using the Set()
, indexOf()
and filter()
functions in JavaScript.
Get the Unique Values From an Array Using the Set()
Function in JavaScript
To get unique values from an array, we can use the Set()
function, which creates a new array with unique values from the existing array. For example, let’s create an array with duplicate values, create a new one with unique values using the Set()
function, and show the result on the console using the console.log()
function in JavaScript. See the code below.
var myArray = ['c', 'b', 'c', 2, 'b'];
var uniqueArray = [...new Set(myArray)]
console.log('Original Array = ', myArray);
console.log('Array with unique values = ', uniqueArray);
Output:
Original Array = (5) ["c", "b", "c", 2, "b"]
Array with unique values = (3) ["c", "b", 2]
In the output, the new array does not contain any duplicate values.
Get the Unique Values From an Array Using the indexOf()
Function in JavaScript
To get unique values from an array, we can create our own function using the indexOf()
function and a loop, which will create a new array with unique values from the existing array. We will use the array to move the elements to the new array and the indexOf()
function to check if an element is already present in the new array or not.
If the element is not in the new array, it will be moved into the new array; otherwise, it will remain. For example, let’s create an array with duplicate values, then create a new array with unique values using our function, and show the result on the console using the console.log()
function in JavaScript. See the code below.
function uArray(array) {
var out = [];
for (var i = 0, len = array.length; i < len; i++)
if (out.indexOf(array[i]) === -1) out.push(array[i]);
return out;
}
var myArray = ['c', 'b', 'c', 2, 'b'];
var uniqueArray = uArray(myArray);
console.log('Original Array = ', myArray);
console.log('Array with unique values = ', uniqueArray);
Output:
Original Array = (5) ["c", "b", "c", 2, "b"]
Array with unique values = (3) ["c", "b", 2]
Here, the new array does not contain any duplicate values.
Get the Unique Values From an Array Using the filter()
Function in JavaScript
To get unique values from an array, we can use the filter()
function, which creates a new array by filtering the values from the existing array depending on a particular condition. The filter()
function will check each value present in the original array. If a value is a duplicate, the function will remove it; otherwise, the value will be added to the new array.
For example, let’s create an array with duplicate values, create a new array with unique values using the filter()
function, and show the result on the console using the console.log()
function in JavaScript. See the code below.
var myArray = ['c', 'b', 'c', 2, 'b'];
var uniqueArray = myArray.filter((val, ind, arr) => arr.indexOf(val) === ind);
console.log('Original Array = ', myArray);
console.log('Array with unique values = ', uniqueArray);
Output:
Original Array = (5) ["c", "b", "c", 2, "b"]
Array with unique values = (3) ["c", "b", 2]
As you can see here, the new array does not contain any duplicate values.
Related Article - JavaScript Array
- How to Check if Array Contains Value in JavaScript
- How to Create Array of Specific Length in JavaScript
- How to Convert Array to String in JavaScript
- How to Remove First Element From an Array in JavaScript
- How to Search Objects From an Array in JavaScript
- How to Convert Arguments to an Array in JavaScript