How to Sort Array of Objects Alphabetically in JavaScript
-
Sort Array of Objects Alphabetically Using the
if
Condition andsort()
Function in JavaScript -
Sort Array of Objects Alphabetically Using
localeCompare()
andsort()
Function in JavaScript -
Sort Array of Objects Alphabetically Using
Collator()
andsort()
Function in JavaScript
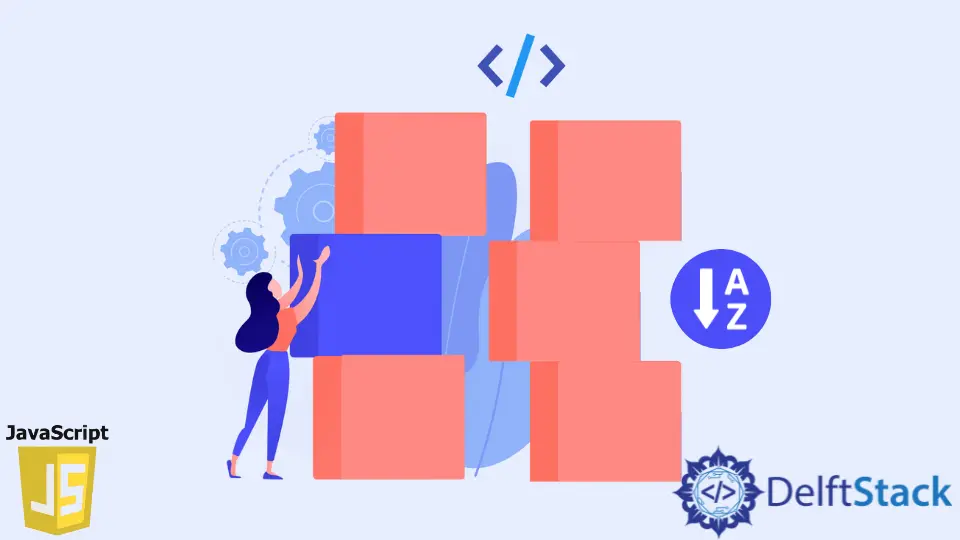
This tutorial will discuss sorting an array of objects alphabetically using the sort()
function in JavaScript.
Sort Array of Objects Alphabetically Using the if
Condition and sort()
Function in JavaScript
If we have an array of strings or integers, we can easily sort them using the sort()
function in JavaScript. For example, let’s sort an array of strings alphabetically using the sort()
function. See the code below.
var a = ['banana', 'apple', 'orange'];
var m = a.sort();
console.log(m);
Output:
Array(3)
0: "apple"
1: "banana"
2: "orange"
length: 3
As you can see, the array is sorted alphabetically, and the result is saved in variable m
. If we have an array of objects, we have to use some conditions before using the sort()
function to sort the array. For example, if we have an array of objects containing the first and last name of some people and we want to sort the array according to the people’s last name. We have to pass a function inside the sort()
function, which will compare the last name of each person, and if the last name of the first person is less than the last name of the second person, the function will return a negative value, and if it is greater, the function will return positive value; and if both are equal, the function will return zero. See the code below.
var a = [
{FirsName: 'Ellie', LastName: 'Williams'},
{FirstName: 'Lara', LastName: 'Croft'}
];
function SortArray(x, y) {
if (x.LastName < y.LastName) {
return -1;
}
if (x.LastName > y.LastName) {
return 1;
}
return 0;
}
var s = a.sort(SortArray);
console.log(s);
Output:
(2) [{…}, {…}]
0: {FirstName: "Lara", LastName: "Croft"}
1: {FirsName: "Ellie", LastName: "Williams"}
length: 2
As you can see, the array is sorted according to the last name. You can also increase the number of objects inside the array. You can also sort the array according to the first name.
Sort Array of Objects Alphabetically Using localeCompare()
and sort()
Function in JavaScript
Instead of using the if
condition, you can also use the localeCompare()
function to compare the strings. It provides many other options for comparison that you can set inside the function. For example, let’s compare the above array of objects using the localeCompare()
function. See the code below.
var a = [
{FirsName: 'Ellie', LastName: 'Williams'},
{FirstName: 'Lara', LastName: 'Croft'}
];
function SortArray(x, y) {
return x.LastName.localeCompare(y.LastName);
}
var s = a.sort(SortArray);
console.log(s);
Output:
(2) [{…}, {…}]
0: {FirstName: "Lara", LastName: "Croft"}
1: {FirsName: "Ellie", LastName: "Williams"}
length: 2
The output is the same as in the above method. You can also set the function to ignore any punctuations and special characters during comparison. For example, if we have punctuation before the last name of a person, the function will not sort the array. In this case, we can use the localeCompare()
function and set it to ignore the punctuations during comparison. See the code below.
var a = [
{FirsName: 'Ellie', LastName: ',Williams'},
{FirstName: 'Lara', LastName: 'Croft'}
];
function SortArray(x, y) {
return x.LastName.localeCompare(y.LastName, 'fr', {ignorePunctuation: true});
}
var s = a.sort(SortArray);
console.log(s);
Output:
(2) [{…}, {…}]
0: {FirstName: "Lara", LastName: "Croft"}
1: {FirsName: "Ellie", LastName: ",Williams"}
length: 2
The array is sorted according to the last name even if there is a punctuation present. You can also ignore special characters if they are present in the string by setting the sensitivity of the localeCompare()
function to base, as shown below.
x.LastName.localeCompare(y.LastName, 'en', {sensitivity: 'base'});
Check this link for more details about the localeCompare()
function.
Sort Array of Objects Alphabetically Using Collator()
and sort()
Function in JavaScript
Instead of using the if
condition, you can also use the Collator()
function to compare the strings. For example, let’s compare the above array of objects using the Collator()
function. See the code below.
const collator = new Intl.Collator('en');
var a = [
{FirsName: 'Ellie', LastName: 'Williams'},
{FirstName: 'Lara', LastName: 'Croft'}
];
function SortArray(x, y) {
return collator.compare(x.LastName, y.LastName);
}
var s = a.sort(SortArray);
console.log(s);
Output:
(2) [{…}, {…}]
0: {FirstName: "Lara", LastName: "Croft"}
1: {FirsName: "Ellie", LastName: "Williams"}
length: 2
The output is the same as in the above methods. You can also change the sorting order to descending by changing the place of the two arguments in the collator.compare()
function. You can also use the Collator()
function to compare strings of a different language; you just need to initialize the collator object with that language. For example, in the above code, we used en
for the English language. Check this link for more details about the Collator()
function.
Related Article - JavaScript Array
- How to Check if Array Contains Value in JavaScript
- How to Create Array of Specific Length in JavaScript
- How to Convert Array to String in JavaScript
- How to Remove First Element From an Array in JavaScript
- How to Search Objects From an Array in JavaScript
- How to Convert Arguments to an Array in JavaScript