JavaScript String Encryption and Decryption
- Understanding String Encryption and Decryption
- Using the CryptoJS Library
- Using Web Crypto API
- Conclusion
- FAQ
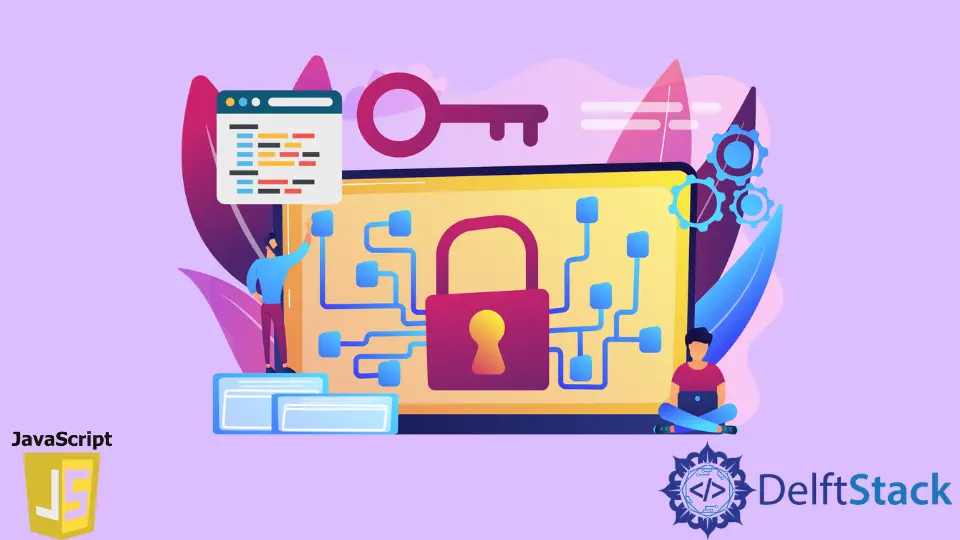
In today’s digital landscape, data security is paramount. One effective way to protect sensitive information is through encryption. If you’re a JavaScript developer, understanding how to encrypt and decrypt strings can be invaluable.
This tutorial introduces you to the essential concepts and methods for encrypting and decrypting strings in JavaScript. We’ll explore various techniques, including the use of libraries and built-in functions, to ensure your data remains safe from prying eyes. Whether you’re building a web application or handling user data, mastering string encryption and decryption will enhance your coding skill set and bolster your application’s security.
Understanding String Encryption and Decryption
Before diving into the implementation, it’s important to grasp what string encryption and decryption mean. Encryption is the process of converting plain text into a coded format, making it unreadable to unauthorized users. Decryption, on the other hand, reverses this process, converting the encrypted data back into its original form. In JavaScript, there are several methods to achieve this, ranging from simple algorithms to using libraries that handle the complexity for you.
Using the CryptoJS Library
One of the most popular libraries for JavaScript encryption is CryptoJS. This library provides a straightforward way to encrypt and decrypt strings using various algorithms, such as AES. To get started, you need to include the CryptoJS library in your project.
Installation
You can install CryptoJS via npm:
npm install crypto-js
Encryption Example
Here’s a simple example of encrypting a string using AES:
const CryptoJS = require("crypto-js");
const secretKey = "mySecretKey";
const originalText = "Hello, World!";
const encryptedText = CryptoJS.AES.encrypt(originalText, secretKey).toString();
console.log("Encrypted Text: ", encryptedText);
Output:
Encrypted Text: U2FsdGVkX1+...
In this example, we first import the CryptoJS library and define a secret key. The AES.encrypt
method is then used to encrypt the original string, and the result is converted to a string format for easy storage or transmission.
Decryption Example
To decrypt the text, you can use the following code:
const decryptedBytes = CryptoJS.AES.decrypt(encryptedText, secretKey);
const decryptedText = decryptedBytes.toString(CryptoJS.enc.Utf8);
console.log("Decrypted Text: ", decryptedText);
Output:
Decrypted Text: Hello, World!
In this decryption example, we use AES.decrypt
to reverse the encryption process. The decrypted bytes are then converted back into a readable string format using toString(CryptoJS.enc.Utf8)
. This method ensures that the original text is retrieved accurately.
Using Web Crypto API
The Web Crypto API is a built-in JavaScript API that provides cryptographic operations in web applications. It allows for secure encryption and decryption without the need for external libraries. Here’s how to use the Web Crypto API for string encryption and decryption.
Encryption Example
async function encryptString(plainText, password) {
const enc = new TextEncoder();
const key = await window.crypto.subtle.importKey(
"raw",
enc.encode(password),
{ name: "PBKDF2" },
false,
["deriveBits", "deriveKey"]
);
const iv = window.crypto.getRandomValues(new Uint8Array(12));
const keyMaterial = await window.crypto.subtle.deriveKey(
{
name: "PBKDF2",
salt: iv,
iterations: 100000,
hash: "SHA-256"
},
key,
{ name: "AES-GCM", length: 256 },
false,
["encrypt"]
);
const cipherText = await window.crypto.subtle.encrypt(
{ name: "AES-GCM", iv: iv },
keyMaterial,
enc.encode(plainText)
);
return { iv: Array.from(iv), cipherText: Array.from(new Uint8Array(cipherText)) };
}
encryptString("Hello, World!", "mySecretPassword").then(result => {
console.log("Encrypted Result: ", result);
});
Output:
Encrypted Result: { iv: [...], cipherText: [...] }
In this example, we define an asynchronous function encryptString
that takes a plain text string and a password. The function uses the PBKDF2 algorithm to derive a key from the password and then encrypts the string using AES-GCM. The result includes both the initialization vector (IV) and the encrypted text.
Decryption Example
To decrypt the encrypted string, use the following function:
async function decryptString(encryptedData, password) {
const enc = new TextEncoder();
const key = await window.crypto.subtle.importKey(
"raw",
enc.encode(password),
{ name: "PBKDF2" },
false,
["deriveBits", "deriveKey"]
);
const iv = new Uint8Array(encryptedData.iv);
const cipherText = new Uint8Array(encryptedData.cipherText);
const keyMaterial = await window.crypto.subtle.deriveKey(
{
name: "PBKDF2",
salt: iv,
iterations: 100000,
hash: "SHA-256"
},
key,
{ name: "AES-GCM", length: 256 },
false,
["decrypt"]
);
const decryptedData = await window.crypto.subtle.decrypt(
{ name: "AES-GCM", iv: iv },
keyMaterial,
cipherText
);
return new TextDecoder().decode(decryptedData);
}
decryptString(encryptedResult, "mySecretPassword").then(decryptedText => {
console.log("Decrypted Text: ", decryptedText);
});
Output:
Decrypted Text: Hello, World!
In this decryption example, we recreate the key using the same password and IV. The decryptString
function reverses the encryption process, allowing us to retrieve the original text accurately. This method is secure and efficient, leveraging built-in browser capabilities.
Conclusion
Understanding JavaScript string encryption and decryption is crucial for any developer focused on data security. By utilizing libraries like CryptoJS or the built-in Web Crypto API, you can easily implement encryption in your applications. With the right techniques, you can ensure that sensitive information remains protected from unauthorized access. Whether you’re developing a web application or handling user data, mastering these encryption methods will significantly enhance your coding skills and improve your application’s security.
FAQ
-
What is string encryption in JavaScript?
String encryption is the process of converting readable text into a coded format to protect sensitive information from unauthorized access. -
Why should I encrypt strings in my applications?
Encrypting strings helps secure sensitive data, ensuring that even if it is intercepted, it cannot be read without the proper decryption key. -
What libraries can I use for string encryption in JavaScript?
Popular libraries include CryptoJS and the built-in Web Crypto API, which provide various encryption algorithms and easy-to-use functions. -
Is the Web Crypto API supported in all browsers?
Most modern browsers support the Web Crypto API, but it’s always a good practice to check compatibility if your application targets older browsers. -
How do I securely store encryption keys?
Encryption keys should be stored securely, ideally in a secure environment, such as a server-side application or a secure key management system.
Harshit Jindal has done his Bachelors in Computer Science Engineering(2021) from DTU. He has always been a problem solver and now turned that into his profession. Currently working at M365 Cloud Security team(Torus) on Cloud Security Services and Datacenter Buildout Automation.
LinkedIn