How to Add Character to String in JavaScript
- Introduction to String Manipulation in JavaScript
- Adding Characters at the Start and End of a String
- Inserting Characters at a Specific Position
- Working With Arrays and Strings
- Conclusion
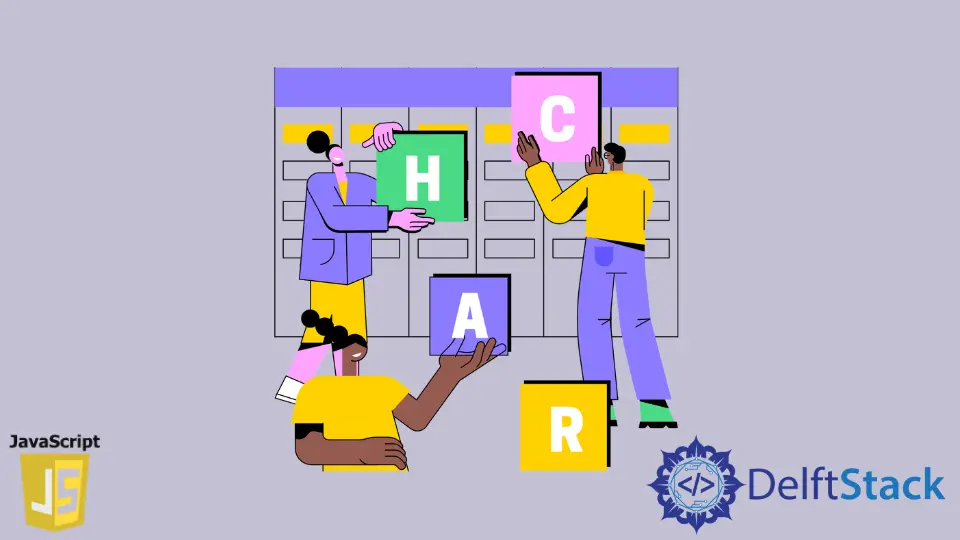
String manipulation, a fundamental aspect of JavaScript, enhances code readability, facilitates dynamic HTML generation, and enables efficient handling of textual data. With various techniques available, developers can manipulate strings in several ways, responding to the diverse demands of web development and programming tasks.
Introduction to String Manipulation in JavaScript
In JavaScript, mastering string manipulation is crucial for dynamic content creation, website development, and data processing. Whether adding variables to an existing string using concat
, merging strings with template literals, or join method array elements, these methods empower developers to construct and modify content seamlessly.
Adding Characters at the Start and End of a String
Using Concatenation
The +
operator, with its straightforward and intuitive use for string concatenation, provides a clear and efficient solution for adding character to the start of a string in JavaScript. Whether for simple modifications or more complex string concatenation scenarios, the +
operator remains a reliable and commonly used tool in a JavaScript developer’s toolkit.
Adding at the Start With +
Operator
The +
operator is a straightforward and commonly used for string concatenation. In the example above, we add H
to the start of the string ello
.
// Original string
let existingString = "ello";
// Character to add
let charToAdd = "H";
// Using the + operator to add the character at the start
let newString = charToAdd + existingString;
// Logging the result
console.log(newString);
Output:
Adding at the End With +
Operator
Similarly, the +
operator is employed to add a character to the end of a string. In this example, we add the character o
to the string Hell
.
// Original string
let existingString = "Hell";
// Character to add
let charToAdd = "o";
// Using the + operator to add the character at the end
let newString = existingString + charToAdd;
// Logging the result
console.log(newString);
Output:
The +
operator, with its clear and intuitive use for string concatenation, provides an efficient solution for adding characters to both the start and end of a string in JavaScript. This is concise, widely adopted, and suitable for various concatenation scenarios in JavaScript development.
String Interpolation
One elegant technique for adding characters to the start of a string is through string interpolation, a feature provided by template literals. This approach not only simplifies the syntax but also enhances readability.
Using literals inside the backticks, we write ${charToAdd}${existingString}
, utilizing string interpolation. This syntax allows us to embed the value of the charToAdd
variable directly at the beginning of the existingString
.
Adding at the Start With String Interpolation
A sleek way to add characters at the beginning of a string is using string interpolation with template literals.
// Original string
let existingString = "ello";
// Character to add
let charToAdd = "H";
// Using string interpolation to add the character at the start
let newString = `${charToAdd}${existingString}`;
// Logging the result
console.log(newString);
We start with the string ello
and aim to add the character H
at the beginning. Using literals, we create a new string, newString
, by embedding ${charToAdd}${existingString}
.
Output:
String interpolation allows us to embed expressions directly within a string, showcasing its power for concise and readable string manipulation in JavaScript.
Adding at the End With String Interpolation
Similarly, string interpolation proves effective for adding characters to the end of a string. In this example, we add the character o
to the string Hell
.
// Original string
let existingString = "Hell";
// Character to add
let charToAdd = "o";
// Using string interpolation to add the character at the end
let newString = `${existingString}${charToAdd}`;
// Logging the result
console.log(newString);
String interpolation in JavaScript is achieved by enclosing expressions inside ${}
within a string literal. This method is concise and effective for combining values and creating a modified string.
Output:
String interpolation provides a clean and modern approach to string concatenation, enhancing readability and offering an expressive technique for string manipulation in JavaScript. Whether at the start or end of a string, this technique stands as a versatile and efficient tool in a JavaScript developer’s toolkit.
the concat()
Method
The concat()
is a versatile tool for string manipulation, providing a straightforward and readable approach to concatenate strings.
Adding at the Start
The concat()
method is a flexible tool for string manipulation, offering a clear and easy way to combine JavaScript strings. In this example, we use concat()
to add the character H
to the start of the string ello
.
// Original string
let existingString = "ello";
// Character to add
let charToAdd = "H";
// Using concat method to add the character at the start
let newString = charToAdd.concat(existingString);
// Logging the result
console.log(newString);
In this case, we invoke charToAdd.concat(existingString)
, effectively adding the character H
to the start of the existing string.
The result is stored in newString
, which now holds the modified string.
Output:
This simplicity makes it a clear and concise solution for adding character at the start of a string.
Adding at the End
Similarly, the concat()
method is a versatile tool for adding character to the end of a string. In this example, we use concat()
to add the character o
to the end of the string Hell
.
// Original string
let existingString = "Hell";
// Character to add
let charToAdd = "o";
// Using concat method to add the character at the end
let newString = existingString.concat(charToAdd);
// Logging the result
console.log(newString);
Using the concat()
method, we concatenate o
with the original string, resulting in the modified string Hello
, stored in the variable newString
.
Output:
The concat()
method provides a concise and effective solution for adding characters to the end of a string, showcasing its versatility in various string manipulation scenarios. This fundamental technique remains a reliable choice for developers working with strings in JavaScript.
Inserting Characters at a Specific Position
Using String slicing()
There are instances where adding characters to a specific position in a string becomes crucial. One effective method to achieve this is through string slicing.
Example:
// Original string
let originalString = "Helo";
// Character to add
let charToAdd = "l";
// Position to insert the character
let position = 2;
// Using string slicing to add the character at a specific position
let newString = originalString.slice(0, position) + charToAdd + originalString.slice(position);
// Logging the result
console.log(newString);
We start with the string Helo
and want to insert the character l
at index 2. Using string slicing, we create newString
by combining the substring before the position, the character to add, and the rest of the original string.
This efficiently inserts the character, resulting in the modified string.
Output:
The use of string slicing provides a clear and efficient solution for inserting characters into strings at specific positions, showcasing the versatility of this fundamental string manipulation technique in JavaScript.
Using substring()
In this section, we’ll explore the concept of achieving this task using the substring()
function. This function provides an elegant and efficient way to manipulate strings by extracting and modifying substrings.
Let’s examine how this can be achieved using the substring()
function:
// Original string
let originalString = "Helo";
// Character to add
let charToAdd = "l";
// Position to insert the character
let position = 2;
// Using substring() to add the character at a specific position
let newString = originalString.substring(0, position) + charToAdd + originalString.substring(position);
// Logging the result
console.log(newString);
We start with the string Helo
and want to insert the character l
at position 2.
Using substring()
, we create newString
by combining the substring before the position, the character to add, and the rest of the original string. This efficiently inserts the character, resulting in the modified string, which is then logged to the console.
Output:
The substring()
function provides a concise and readable solution to add character into strings at specific positions, showcasing its effectiveness as a valuable tool in JavaScript string manipulation.
Working With Arrays and Strings
Array.join()
and String.split()
When it comes on how to add character to a string while working with arrays and strings, a combination of array.join()
and string.split()
provides an efficient and flexible solution. This approach leverages the capabilities to achieve the desired string manipulation.
Example:
// Original string
let existingString = "Helo";
// Character to add
let charToAdd = "l";
// Position to insert the character
let position = 3;
// Convert the string to an array of characters
let stringArray = existingString.split('');
// Insert the character at the specified position
stringArray.splice(position, 0, charToAdd);
// Join the array back into a string
let newString = stringArray.join('');
// Logging the result
console.log(newString);
We begin with the string Helo
and aim to add character l
at position 3. By converting the string into an array using existingString.split('')
, we create stringArray
.
Then, using splice()
on the array, we insert charToAdd
at position 3 without removing elements. After manipulating, we join it back into a string with stringArray.join('')
, resulting in the modified string, newString
.
The output in the console will be:
The combination of array.join()
and string.split()
proves to be a versatile and effective approach for string manipulation, providing developers with a powerful tool for working with arrays and strings in JavaScript.
Conclusion
In JavaScript, manipulating strings involves various methods like concatenation
, string interpolation
, concat()
, string slicing()
, substring()
, array.join()
, and string.split()
. Whether using the +
operator or specific methods, developers can efficiently add character to create a new string.
These accept two strings or two arguments, offering flexibility for string manipulation. Developers can employ these techniques in functions, loops, or objects, and the resulting strings can be logged to the console.