How to Populate an Array in Java
-
Use
{ }
to Populate an Array in Java -
Use the
for
Loop to Populate an Array in Java -
Use the
Arrays.copyOf()
Method to Fill Element in a Java Array -
Use the
Arrays.fill()
Method to Fill Elements in a Java Array
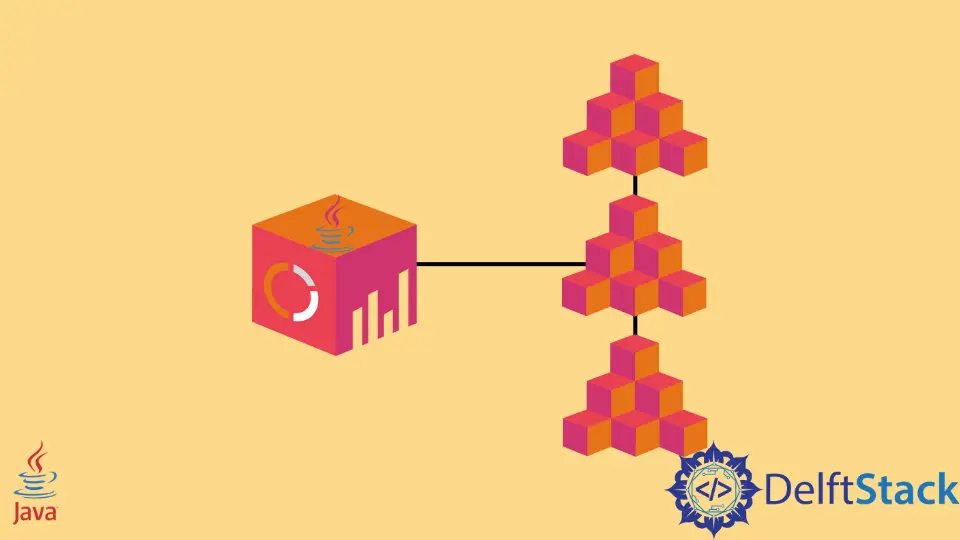
Based on the user definition, the array will be primitive, or the object (or non-primitive) references of a class.
In a primitive data type array, the elements are stored in a contiguous memory location. In contrast, in a non-primitive data type, the elements are stored in dynamic memory (Heap segment).
In this tutorial, we populate an array in Java. Populate here means filling the array with some values.
Use { }
to Populate an Array in Java
It is the basic and one of the simplest methods to populate an array. Curly Braces {} are used you define the elements of an array.
For example,
import java.util.*;
public class Num {
public static void main(String args[]) {
int arr[] = {1, 3, 5, 7, 11}; // Declaration of elements using { }
for (int j = 0; j < arr.length; j++) {
System.out.print(array[j] + " ");
}
}
}
Output:
1 3 5 7 11
Use the for
Loop to Populate an Array in Java
The Scanner
class is used to scan the array elements from the user. We run a loop until the user’s length & using the object of the Scanner
class elements are entered in each iteration.
See the following code.
import java.util.Scanner;
public class ArrayInputUsingLoop {
public static void main(String[] args) {
int number;
Scanner obj = new Scanner(System.in);
System.out.print("Total number of elements: ");
number = obj.nextInt();
int[] array = new int[20];
System.out.println("Enter the elements of the array: ");
for (int i = 0; i < number; i++) {
array[i] = obj.nextInt(); // reads elements from the user
}
System.out.println("Array elements you entered are: ");
for (int i = 0; i < number; i++) {
System.out.println(array[i]);
}
}
}
Output:
Total number of elements: 5
Enter the elements of the array:
5
4
3
2
1
Array elements you entered are:
5
4
3
2
1
Use the Arrays.copyOf()
Method to Fill Element in a Java Array
The Array.copyOf()
method belongs to java.util.Arrays
class. This function copies the particular array and truncates it with zeros or null values if needed to maintain the copy array’s given length.
There will be identical values for all the valid indices in the original and the copied array.
For example,
import java.util.Arrays;
public class Main {
public static void main(String[] args) {
int[] array1 = new int[] {8, 9, 10, 11, 12};
System.out.println("First array is:");
for (int i = 0; i < array1.length; i++) {
System.out.println(array1[i]);
}
int[] array2 = Arrays.copyOf(array1, 7);
array2[5] = 6;
array2[6] = 7;
System.out.println("New array after copying elements is:");
for (int i = 0; i < array2.length; i++) {
System.out.println(array2[i]);
}
}
}
Output:
First array is:
8
9
10
11
12
New array after copying elements is:
8
9
10
11
12
6
7
If the length exceeds the original array, then the extra elements are compensated with 0 value.
For example,
import java.util.Arrays;
public class ArrayCopy {
public static void main(String args[]) {
int[] originalarray = new int[] {7, 8, 9};
System.out.println("The Original Array is : \n");
for (int i = 0; i < originalarray.length; i++) System.out.print(originalarray[i] + " ");
int[] copyarray = Arrays.copyOf(originalarray, 5);
System.out.print("\nNew Array copy of greater length is:\n");
for (int i = 0; i < copyarray.length; i++) System.out.print(copyarray[i] + " ");
}
}
Output:
The Original Array is :
7 8 9
New Array copy of greater length is:
7 8 9 0 0
Use the Arrays.fill()
Method to Fill Elements in a Java Array
The Arrays.fill()
method belongs to the java.util.Arrays
class.
Using this method, we can replace all the elements in a given array with the newly entered element. All the positions of the array will be replaced or filled by the value specified element.
For example,
import java.util.Arrays;
public class ArrayFill {
public static void main(String[] args) {
int array[] = {6, 7, 8, 9, 10};
Arrays.fill(array, 5);
System.out.println(Arrays.toString(array));
}
}
Output:
[5, 5, 5, 5, 5]
The original array can be filled partly by the new elements by specifying the index.
For example,
import java.util.Arrays;
public class ArrayFill2 {
public static void main(String[] args) {
int array[] = {7, 8, 9, 10, 11};
Arrays.fill(array, 2, 5, 0); // Replace elements from index 2 to index 4 by 0
System.out.println(Arrays.toString(array));
}
}
Output:
[7, 8, 0, 0, 0]