How to Get Type of Object in Java
-
Get Object Type Using
getClass()
in Java -
Get Object Type Using
instanceOf
in Java - Get Type of a Manually Created Class Object in Java
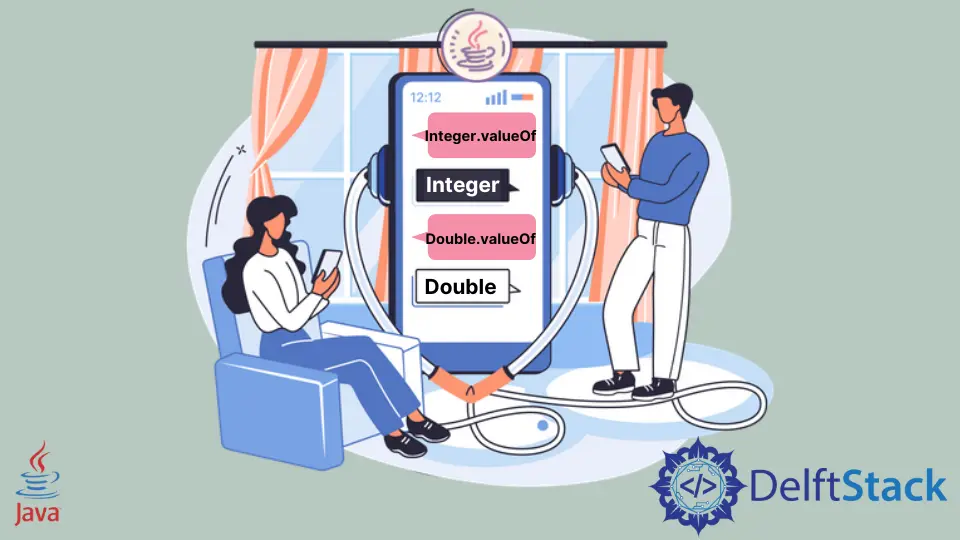
In this article, we’ll learn how to get the type of object in Java. It’s helpful to check the object type when the object comes from a source. It’s a place where we can’t verify the type of objects, such as from an API or a private class that we don’t have access to.
Get Object Type Using getClass()
in Java
In the first method, we check the type of Object of wrapper classes like Integer
and String
. We have two objects, var1
and var2
, to check the type. We’ll use the getClass()
method of the Object
class, the parent class of all objects in Java.
We check the class using the if
condition. As the wrapper classes also contain a field class that returns the type, we can check whose type matches with var1
and var2
. Below, we check both the objects with three types.
public class ObjectType {
public static void main(String[] args) {
Object var1 = Integer.valueOf("15");
Object var2 = String.valueOf(var1);
if (var1.getClass() == Integer.class) {
System.out.println("var1 is an Integer");
} else if (var1.getClass() == String.class) {
System.out.println("var1 is a String");
} else if (var1.getClass() == Double.class) {
System.out.println("var1 is a Double");
}
if (var2.getClass() == Integer.class) {
System.out.println("var2 is an Integer");
} else if (var2.getClass() == String.class) {
System.out.println("var2 is a String");
} else if (var2.getClass() == Double.class) {
System.out.println("var2 is a Double");
}
}
}
Output:
var1 is an Integer
var2 is a String
Get Object Type Using instanceOf
in Java
Another method to get the type of object in Java is by using the instanceOf
function; it returns if the object’s instance matches with the given class. In this example, we have the objects var1
and var2
that are checked with these three types: Integer
, String
, and Double
; if any of the conditions meet, we can execute a different code.
Because var1
is of type Integer
, the condition var1 instanceOf Integer
will become true, and var2
is Double
so, var2 instanceOf Double
will become true.
public class ObjectType {
public static void main(String[] args) {
Object var1 = Integer.valueOf("15");
Object var2 = Double.valueOf("10");
if (var1 instanceof Integer) {
System.out.println("var1 is an Integer");
} else if (var1 instanceof String) {
System.out.println("var1 is a String");
} else if (var1 instanceof Double) {
System.out.println("var1 is a Double");
}
if (var2 instanceof Integer) {
System.out.println("var2 is an Integer");
} else if (var2 instanceof String) {
System.out.println("var2 is a String");
} else if (var2 instanceof Double) {
System.out.println("var2 is a Double");
}
}
}
Output:
var1 is an Integer
var2 is a Double
Get Type of a Manually Created Class Object in Java
We checked the types of wrapper classes, but in this example, we get the type of three objects of three manually-created classes. We create three classes:ObjectType2
, ObjectType3
and ObjectType4
.
ObjectType3
inherits ObjectType4
, and ObjectType2
inherits ObjectType3
. Now we want to know the type of objects of all these classes. We have three objects, obj
, obj2
, and obj3
; we use both the methods that we discussed in the above examples that are getClass()
and instanceOf
.
However, there differences between the type of obj2
. The obj2
variable returned the type ObjectType4
while its class is ObjectType3
. It happens because we inherit the ObjectType4
class in the ObjectType3
and the instanceOf
checks all the classes and subclasses. The obj
returned ObjectType3
because the getClass()
function checks only the direct class.
public class ObjectType {
public static void main(String[] args) {
Object obj = new ObjectType2();
Object obj2 = new ObjectType3();
Object obj3 = new ObjectType4();
if (obj.getClass() == ObjectType4.class) {
System.out.println("obj is of type ObjectType4");
} else if (obj.getClass() == ObjectType3.class) {
System.out.println("obj is of type ObjectType3");
} else if (obj.getClass() == ObjectType2.class) {
System.out.println("obj is of type ObjectType2");
}
if (obj2 instanceof ObjectType4) {
System.out.println("obj2 is an instance of ObjectType4");
} else if (obj2 instanceof ObjectType3) {
System.out.println("obj2 is an instance of ObjectType3");
} else if (obj2 instanceof ObjectType2) {
System.out.println("obj2 is an instance of ObjectType2");
}
if (obj3 instanceof ObjectType4) {
System.out.println("obj3 is an instance of ObjectType4");
} else if (obj3 instanceof ObjectType3) {
System.out.println("obj3 is an instance of ObjectType3");
} else if (obj3 instanceof ObjectType2) {
System.out.println("obj3 is an instance of ObjectType2");
}
}
}
class ObjectType2 extends ObjectType3 {
int getAValue3() {
System.out.println(getAValue2());
a = 300;
return a;
}
}
class ObjectType3 extends ObjectType4 {
int getAValue2() {
System.out.println(getAValue1());
a = 200;
return a;
}
}
class ObjectType4 {
int a = 50;
int getAValue1() {
a = 100;
return a;
}
}
Output:
obj is of type ObjectType2
obj2 is an instance of ObjectType4
obj3 is an instance of ObjectType4
Rupam Saini is an android developer, who also works sometimes as a web developer., He likes to read books and write about various things.
LinkedIn