How to Add Integers to an Array in Java
- Use Another Array to Add Integers to an Array in Java
-
Use the
add()
Function to Add Integers to an Array in Java -
Use the
Arrays.fill()
Method to Add Integers to an Array in Java -
Use the
System.arraycopy()
Method to Add Integers to an Array in Java - Conclusion
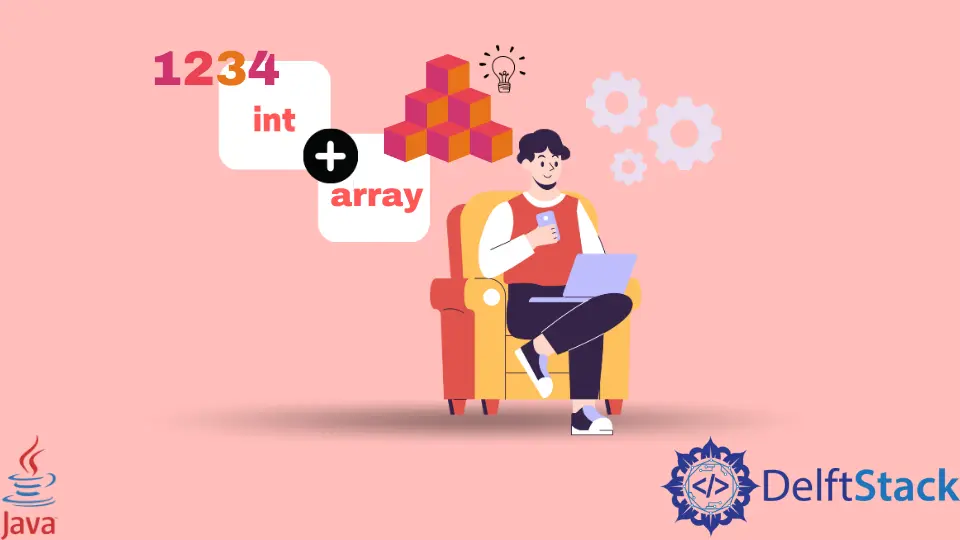
In Java, arrays provide a convenient way to store and manipulate collections of elements. If you’re working on a project that involves handling integers, you’ll likely need to add integers to an array at some point.
This article will guide you through the process of adding integers to an array in Java.
Use Another Array to Add Integers to an Array in Java
Adding integers to an array in Java can be accomplished by creating another array of greater size to accommodate additional elements. Although this method is not the most memory-efficient, it is straightforward and effective.
The key idea behind using another array is to create a new array with a larger size, copy the elements from the original array to the new one, and then add the desired integers.
Here’s a code example to better illustrate this method:
public class AddIntegersUsingAnotherArray {
public static void main(String[] args) {
int[] originalArray = {1, 2, 3, 4, 5};
int newSize = originalArray.length + 2;
int[] newArray = new int[newSize];
for (int i = 0; i < originalArray.length; i++) {
newArray[i] = originalArray[i];
}
newArray[newArray.length - 2] = 10;
newArray[newArray.length - 1] = 12;
for (int value : newArray) {
System.out.print(value + " ");
}
}
}
In our Java program, we begin by declaring an original array named originalArray
containing some initial elements. Our goal is to extend this array by adding two more integers.
To achieve this, we calculate the size of the new array (newSize
) by adding the desired number of integers to be added (in this case, 2
) to the length of the original array. We then create a new array (newArray
) with this calculated size.
Next, we use the following for
loop to iterate through the original array, copying each element to the corresponding position in the new array. This ensures that the new array contains all the elements from the original array.
for (int i = 0; i < originalArray.length; i++) {
newArray[i] = originalArray[i];
}
Following the element copying process, we proceed to add the desired integers to the new array. Specifically, we assign the value 10
to the second-to-last position (newArray.length - 2
) and 12
to the last position (newArray.length - 1
).
To show the result, we utilize another for
loop to print the elements of the new array.
The output of the provided code will be:
1 2 3 4 5 10 12
Use the add()
Function to Add Integers to an Array in Java
An alternative and more efficient method for adding integers to an array in Java is by utilizing the add()
function. This function is part of the ArrayList
class, which is a dynamic array implementation in Java.
Syntax of the add()
Function:
boolean add(E element)
The add()
function takes an element of type E
(generic type) as its parameter and returns a boolean value. It returns true
if the element is successfully added to the array and false
otherwise.
When the add()
function is called, it appends the specified element to the end of the array. However, if an index is specified, the element is inserted at that index, and the elements at and after that index are shifted to accommodate the new element.
Here’s an example to see how the add()
function works:
import java.util.ArrayList;
public class AddIntegersToArray {
public static void main(String[] args) {
ArrayList<Integer> integerArray = new ArrayList<>();
integerArray.add(10);
integerArray.add(20);
integerArray.add(30);
integerArray.add(40);
System.out.println("Array Elements: " + integerArray);
}
}
Output:
Array Elements: [10, 20, 30, 40]
In this example, we start by importing the ArrayList
class from the java.util
package. We then create an instance of ArrayList
called integerArray
to store integers.
Here, the add()
function is used to add integers (10
, 20
, 30
, and 40
in this case) to the array. Finally, we print the array elements using System.out.println()
.
The add()
function appends each integer to the end of the array. In this way, we build our array dynamically without the need to specify its size beforehand.
This is an efficient way to manage arrays in Java, especially when the size of the array is not known in advance.
Use the Arrays.fill()
Method to Add Integers to an Array in Java
While the add()
function is powerful for dynamic array management, there are scenarios where you might want to initialize an array with a specific value. Java provides the Arrays.fill()
method for precisely this purpose.
This method efficiently populates an array with a specified value, allowing for quick and concise initialization.
Syntax of Arrays.fill()
:
void fill(int[] array, int value)
The fill()
method takes two parameters: the array to be filled (int[] array
) and the value with which to fill the array (int value
). This method does not return a value; instead, it modifies the input array directly.
The fill()
method traverses the given array and sets each element to the specified value. This is particularly useful when initializing an array with a default value or resetting its content.
Complete code example:
import java.util.Arrays;
public class FillIntArray {
public static void main(String[] args) {
int[] integerArray = new int[5];
Arrays.fill(integerArray, 50);
System.out.println("Array Elements: " + Arrays.toString(integerArray));
}
}
Output:
Array Elements: [50, 50, 50, 50, 50]
In this example, we declare an integer array called integerArray
with a size of 5
. The Arrays.fill()
method is employed to fill every element in the array with the value 50
.
As you can see, the result is an array where each element is initialized to the specified value.
The Arrays.fill()
method provides a concise way to initialize or reset array elements to a specific value. This is particularly useful when working with arrays that need a predetermined starting point, ensuring consistency in array initialization.
Use the System.arraycopy()
Method to Add Integers to an Array in Java
In Java, another method for adding integers to an array is through the use of System.arraycopy()
. This method provides a means to efficiently copy elements from one array to another, allowing for the insertion of new elements at specific positions.
Syntax of System.arraycopy()
:
static void arraycopy(Object src, int srcPos, Object dest, int destPos, int length)
The parameters include:
Object src
: The source array.int srcPos
: The starting position in the source array.Object dest
: The destination array.int destPos
: The starting position in the destination array.int length
: The number of elements to be copied.
The arraycopy()
method copies a specified range of elements from the source array (src
) to a specified position in the destination array (dest
).
Let’s see a working code example to better understand how this works:
public class ArrayCopyExample {
public static void main(String[] args) {
int[] sourceArray = {10, 20, 30, 40, 50};
int[] destinationArray = new int[sourceArray.length + 3];
System.arraycopy(sourceArray, 0, destinationArray, 0, sourceArray.length);
destinationArray[sourceArray.length] = 60;
destinationArray[sourceArray.length + 1] = 70;
destinationArray[sourceArray.length + 2] = 80;
System.out.println("Array Elements: " + java.util.Arrays.toString(destinationArray));
}
}
Output:
Array Elements: [10, 20, 30, 40, 50, 60, 70, 80]
In this example, we have an initial array called sourceArray
with values 10
, 20
, 30
, 40
, and 50
. We then create a larger array, destinationArray
, with enough space to accommodate the existing elements and additional integers.
The System.arraycopy()
method is employed to copy the elements from sourceArray
to destinationArray
. The parameters specify the source array (sourceArray
), the starting position in the source array (0
), the destination array (destinationArray
), the starting position in the destination array (0
), and the number of elements to copy (sourceArray.length
).
Following the array copy operation, new elements (60
, 70
, and 80
) are added to destinationArray
at positions corresponding to the length of sourceArray
.
The System.arraycopy()
method is particularly useful when working with arrays of varying sizes and when precise control over element placement is required.
Conclusion
In conclusion, adding integers to an array in Java offers various approaches, each catering to specific requirements.
Whether you prefer a straightforward method with another array, the dynamic nature of ArrayList
, the efficiency of Arrays.fill()
, or the precision of System.arraycopy()
, Java provides the flexibility to choose the approach that best suits your programming needs.