How to Convert String Into Number in TypeScript
-
Convert String Into Number Using the Unary Plus (
+
) Operator in TypeScript -
Convert String Into Number Using the
parseInt()
Function in TypeScript -
Convert String Into Number Using the
parseFloat()
Function in TypeScript -
Convert String Into Number Using the Global
Number()
Function in TypeScript -
Check for
NaN
After Converting String Into Number in TypeScript
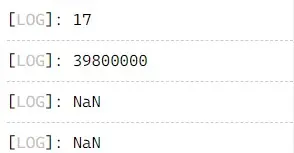
This article will discuss how to convert a string into a number using different methods in TypeScript with examples. In TypeScript, we can convert a string into a number in a few ways.
The unary plus (+
) operator and global Number()
function are very helpful in TypeScript. We can also utilize the parseInt()
and parseFloat()
functions.
We can show you some examples of how to utilize these functions.
Convert String Into Number Using the Unary Plus (+
) Operator in TypeScript
The unary plus (+
) operator changes all string representations of numbers, boolean values (true and false), and null to numbers. The unary plus operator will be NaN
if the operand cannot be changed into a number.
Let’s go through some examples, as shown below.
Example code:
# TypeScript
console.log(+"111")
console.log(+"112.5175")
console.log(+"10AA0.5165")
console.log(+"")
console.log(+" ")
console.log(+null)
console.log(+undefined)
console.log(+Infinity)
console.log(+true)
console.log(+false)
console.log(+"0x44")
console.log(+"0066")
console.log(+"0o21")
console.log(+"3.98e7")
console.log(+"71 35")
console.log(+"BA 35")
Output:
We can convert any string to a number; it converts an empty string or Null
to 0
. As we can see, true
became 1
and false
turned into 0
.
We can also convert Octal/Hexa numbers into a decimal; we have to work accurately on scientific notation numbers. If it fails to convert into a number, it returns NaN
.
Convert String Into Number Using the parseInt()
Function in TypeScript
The parseInt()
function analyzes a string and changes it into a number. The following is the syntax of the parseInt()
function.
If we don’t give the radix, it supposes a Hexadecimal number.
Syntax:
# Typescript
parseInt(string, radix)
As shown below, let us go through an example and use different methods.
Example code:
# TypeScript
console.log(parseInt("120"))
console.log(parseInt("100.5225"))
console.log(parseInt("10AA0.3375"))
console.log(parseInt(""))
console.log(parseInt(null))
console.log(parseInt(Infinity)
console.log(parseInt(true))
console.log(parseInt(false))
console.log(parseInt("0x12"))
console.log(parseInt("0042"))
console.log(parseInt("0o56"))
console.log(parseInt("3.285e7"))
console.log(parseInt("95 35"))
console.log(parseInt("CA 35"))
Output:
As we can see, we can convert any string into a number. We can convert empty strings, null
, infinity
, true
, false
into NaN
.
If the string starts with 0x
, it is a Hexadecimal number. It is important to know that parseInt()
does not recognize the octal number that begins with 0o
; old browsers start with 0
as octal.
It ignores the major and trailing spaces. If the start of the string is a number, then it turns into a number and avoids the rest.
We can make use of the radix. The radix starts from 2 to 36:
- 2 is used for binary
- 8 is used for octal
- 10 is used for decimal
- 16 is used for the Hexadecimal number
Example code:
# TypeScript
console.log(parseInt("22"));
console.log(parseInt("56",8));
console.log(parseInt("54",19));
console.log(parseInt("021"));
console.log(parseInt("061",9));
console.log(parseInt("011",11));
console.log(parseInt("0x21"));
console.log(parseInt("0x41",3));
console.log(parseInt("0x11",19));
console.log(parseInt("0o21"));
console.log(parseInt("0o41",3));
console.log(parseInt("0o11",26));
Output:
Convert String Into Number Using the parseFloat()
Function in TypeScript
We can discuss one more function for this topic. parseFloat()
is a function that converts a string into a number.
Example code:
# TypeScript
console.log(parseFloat("200"))
console.log(parseFloat("223.5535"))
console.log(parseFloat("11AA0.5225"))
console.log(parseFloat(""))
console.log(parseFloat(null))
console.log(parseFloat(Infinity))
console.log(parseFloat(true))
console.log(parseFloat(false))
console.log(parseFloat("0x77"))
console.log(parseFloat("0042"))
console.log(parseFloat("0o11"))
console.log(parseFloat("3.228e7"))
console.log(parseFloat("45 85"))
console.log(parseFloat("FA 95"))
Output:
We can convert a string into a decimal or a number. We have converted the empty strings, null
, true
, and false
into a NaN
.
This function returns infinity
as it is. If the string starts with 0x
or 0o
, it changes into 0
. Hexadecimal or octal numbers are not recognized.
It ignores the major and trailing spaces. If the string’s starting character is a number, it turns into a number and avoids the rest.
Convert String Into Number Using the Global Number()
Function in TypeScript
We can use the global Number()
function. It is like a unary plus (+
) operator.
Example code:
# TypeScript
console.log(Number("320"))
console.log(Number("120.5225"))
console.log(Number("15AA0.5245"))
console.log(Number(""))
console.log(Number(" "))
console.log(Number(null))
console.log(Number(undefined))
console.log(Number(Infinity))
console.log(Number(true))
console.log(Number(false))
console.log(Number("0x43"))
console.log(Number("0052"))
console.log(Number("0o11"))
console.log(Number("3.435e7"))
console.log(Number("15 99"))
console.log(Number("DE 25"))
Output:
We can see in the output that we have successfully converted a string into a number. It converted null
to 0
.
The true
boolean value is converted to 1
, and the false
becomes 0
. We can also convert Octal/Hexa numbers into decimals.
We can work correctly on scientific notations numbers. If it fails to convert, then it will return NaN
.
Check for NaN
After Converting String Into Number in TypeScript
NaN
stands for Not a Number
. It is the output of numerical operations, but it is not a number.
After the conversion, we can check if the value is NaN
using the isNaN()
method.
Example code:
# TypeScript
strToNum("AB 35")
strToNum("35")
function strToNum(Valnum) {
if (isNaN(+Valnum)) {
console.log("This is not a number")
} else {
console.log(+Valnum)
}
}
Output:
Rana is a computer science graduate passionate about helping people to build and diagnose scalable web application problems and problems developers face across the full-stack.
LinkedIn