How to Sum an Array of Numbers in Ruby
-
Use
Array#sum
to Sum an Array of Numbers in Ruby -
Use
Array#inject
to Sum an Array of Numbers in Ruby -
Use
Array#reduce
to Sum an Array of Numbers in Ruby -
Use the
+
Operator WithArray#reduce
to Sum an Array of Numbers in Ruby -
Use
Array#each
to Sum an Array of Numbers in Ruby - Conclusion
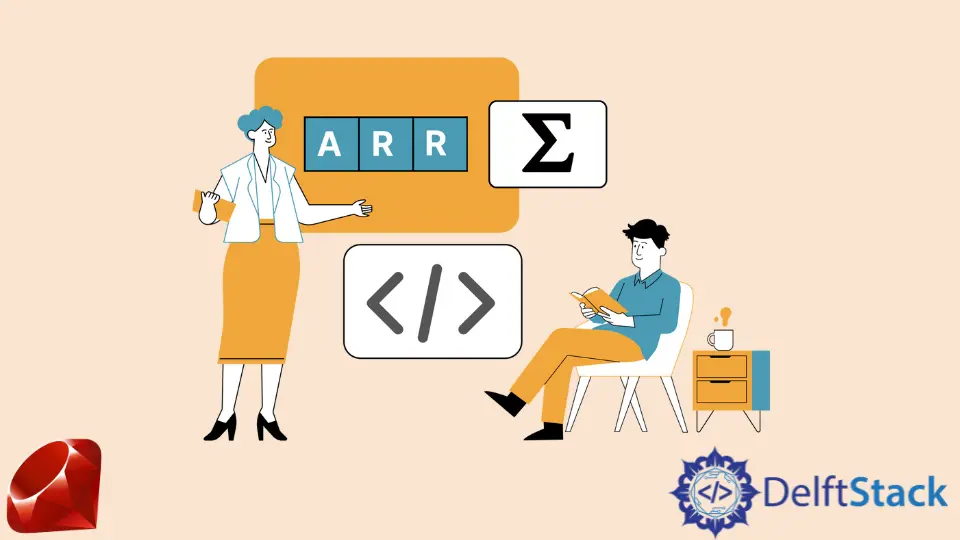
Ruby, a dynamically typed and object-oriented programming language, offers several elegant and concise ways to perform common operations on arrays. Summing an array of numbers is a fundamental task, and Ruby provides developers with multiple methods to achieve this.
In this article, we’ll explore various approaches to sum an array of numbers in Ruby.
Use Array#sum
to Sum an Array of Numbers in Ruby
Since version 2.4, Ruby has provided a concise and elegant solution for summing an array of numbers: the Array#sum
method. This method simplifies the process of calculating the sum of elements in an array.
The syntax for using the Array#sum
method is quite straightforward:
array.sum
Simply call the sum
method on your array, and Ruby will handle the rest. This method works seamlessly with arrays containing numerical elements, making it an ideal choice for summing numeric data.
Consider the following example, where we have an array of numbers:
numbers = [1, 2, 3, 4, 5]
sum = numbers.sum
puts sum
In this example, we have an array named numbers
containing the values [1, 2, 3, 4, 5]
. The magic happens with the sum
method, which is called on the array.
This single method is designed to iterate through the array, adding up all the numeric elements and providing the sum as the result.
The sum
method eliminates the need for explicit iteration or complex block logic. It takes care of the summation process internally, making the code clean and expressive.
Output:
15
Use Array#inject
to Sum an Array of Numbers in Ruby
While the Array#sum
method offers a clean and concise solution for summing numeric arrays, Ruby’s versatility shines through another powerful tool: the Enumerable#inject
method. This method provides a more generalized approach to accumulation, allowing for a wide range of operations beyond simple addition.
The inject
method is part of the Enumerable
module and can be used with arrays. Its syntax involves specifying an initial value and providing a block that defines the accumulation operation:
enumerable.inject(initial) { |_result, _element| block }
Here, enumerable
is the array we’re working with, initial
is the starting value for the accumulation, and the block defines how each element contributes to the result.
Let’s use the inject
method to sum an array of numbers:
numbers = [1, 2, 3, 4, 5]
sum = numbers.inject(0) { |result, element| result + element }
puts sum
Here, we initialize the variable sum
to zero, which serves as the starting point for our accumulation. The inject
method iterates through each element of the array (numbers
) and applies the block.
The block takes two parameters: result
(the current accumulated value) and element
(the current element from the array). In our case, the block adds the current element to the accumulated result.
The inject
method iterates through the array, updating the result
at each step based on the block’s logic. After processing all elements, the final sum is stored in the variable sum
.
Output:
15
The inject
method is particularly useful when you need more flexibility in defining the accumulation logic. It allows for a wide range of operations beyond simple addition.
Use Array#reduce
to Sum an Array of Numbers in Ruby
Ruby provides yet another versatile tool: the Array#reduce
method. Similar to inject
, reduce
offers flexibility in defining accumulation logic, making it a powerful choice for summing an array of numbers.
The reduce
method shares the same syntax as inject
and is often considered an alias for it. It requires an initial value and a block that specifies how elements should be combined:
enumerable.reduce(initial) { |_result, _element| block }
Here, enumerable
is the array we’re working with, initial
is the starting value for accumulation, and the block defines how each element contributes to the result.
Let’s use the reduce
method to sum an array of numbers:
numbers = [1, 2, 3, 4, 5]
sum = numbers.reduce(0) { |result, element| result + element }
puts sum
In this example, we set the initial value of sum
to zero, which serves as the starting point for our accumulation. The reduce
method iterates through each element of the array (numbers
), applying the block logic.
The block takes two parameters: result
(the current accumulated value) and element
(the current element from the array). The block, in our case, adds the current element to the accumulated result.
Similar to inject
, the reduce
method iterates through the array, updating the result
at each step based on the block’s logic. After processing all elements, the final sum is stored in the variable sum
.
Output:
15
While reduce
and inject
are often used interchangeably, the former is preferred by some developers for its clarity and readability. The choice between reduce
and inject
ultimately depends on personal preference and coding style.
Use the +
Operator With Array#reduce
to Sum an Array of Numbers in Ruby
Ruby’s expressive syntax often allows for elegant solutions to common tasks. When it comes to summing an array of numbers, a concise approach is to leverage the +
operator with the Array#reduce
method.
This method takes advantage of Ruby’s ability to use symbols as shorthand for certain operations.
The syntax for using the +
operator with reduce
involves passing the :+
symbol to the method:
enumerable.reduce(:+)
Here, enumerable
is the array we’re working with, and :+
is a symbol shorthand for the addition operation.
Let’s use the +
operator with the reduce
method to sum an array of numbers:
numbers = [1, 2, 3, 4, 5]
sum = numbers.reduce(:+)
puts sum
In this example, we take advantage of Ruby’s succinct syntax by applying the +
operator directly within the reduce
method. The reduce
method iterates through each element of the array (numbers
), using the :+
symbol as a shorthand for addition.
The block, in this case, is replaced by the symbol :+
, which tells Ruby to add the current element to the accumulated result. This results in a clear and concise representation of the summation process.
Output:
15
This approach offers a balance of brevity and clarity, making the code easy to read and understand. It’s a testament to Ruby’s flexibility and the power of symbols as concise representations of operations within the language.
Use Array#each
to Sum an Array of Numbers in Ruby
While Ruby offers concise methods like Array#sum
and Array#reduce
for array summation, sometimes a more explicit approach is preferred. Enter the Array#each
method, a traditional iteration technique that provides explicit control over the loop.
The syntax for using Array#each
involves iterating through each element of the array and applying a block:
array.each { |_element| block }
Here, array
is the array we’re working with, and the block defines the operation to be performed on each element.
Let’s use the each
method to sum an array of numbers:
numbers = [1, 2, 3, 4, 5]
sum = 0
numbers.each { |number| sum += number }
puts sum
In this code, we initialize the variable sum
to zero, serving as the starting point for our accumulation. We then use the each
method on the numbers
array, iterating through each element.
The block, represented by { |number| sum += number }
, adds each element to the accumulating sum
.
The explicit nature of this approach provides a clear view of the iteration process. For each element in the array, the block is executed, updating the sum
variable accordingly.
Output:
15
While not as concise as some other methods, Array#each
offers a straightforward and explicit way to iterate through an array. This approach is beneficial when you desire fine-grained control over the iteration process or need to perform more complex operations within the block.
Conclusion
Summing an array of numbers in Ruby can be accomplished using various methods, each with its advantages. The choice of method depends on factors such as readability, conciseness, and personal preference.
With the array of options provided in this guide, developers can select the approach that best fits their coding style and the requirements of their specific project. Whether you favor the simplicity of Array#sum
, the traditional Array#each
method, or the flexibility of Array#inject
and Array#reduce
, Ruby empowers you to write clean and expressive code for array summation.