Difference Between Each_with_index and each.with_index in Ruby
- Understanding each_with_index
- Exploring each.with_index
- Key Differences Between each_with_index and each.with_index
- Conclusion
- FAQ
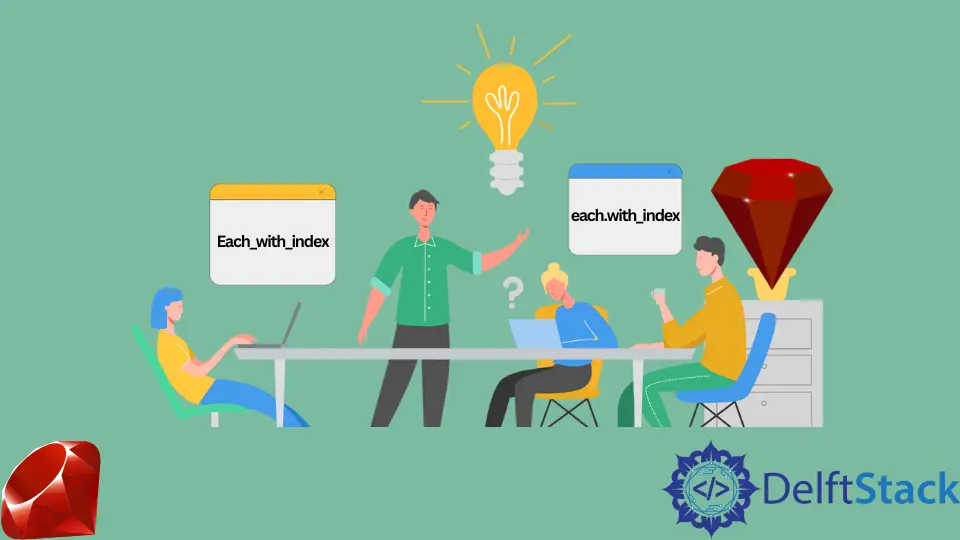
Ruby is a versatile programming language that offers various ways to iterate over collections. Among these methods, each_with_index
and each.with_index
are two popular options that can be confusing for newcomers. Both serve the purpose of iterating through an array while providing the index of each element. However, they have distinct syntaxes and use cases that can affect your code’s readability and performance.
In this tutorial, we will explore the differences between each_with_index
and each.with_index
, providing clear examples to illustrate their usage. By understanding these methods, you can write more efficient Ruby code and enhance your programming skills.
Understanding each_with_index
The each_with_index
method is a built-in Ruby method that allows you to iterate through an array while keeping track of the current index. This method is particularly useful when you need both the value and its position in the array for processing. Here’s how it works:
fruits = ["apple", "banana", "cherry"]
fruits.each_with_index do |fruit, index|
puts "#{index}: #{fruit}"
end
Output:
0: apple
1: banana
2: cherry
In this example, the each_with_index
method is called on the fruits
array. The block takes two parameters: fruit
, which represents the current element, and index
, which represents the current index. As the loop iterates through the array, it prints both the index and the fruit name. This method is straightforward and easy to read, making it ideal for cases where you need to access both the element and its index simultaneously.
The each_with_index
method is also flexible. You can even provide a starting index if you want to begin counting from a number other than zero. For instance, if you wanted to start counting from one, you could do so by adding a second argument:
fruits.each_with_index(1) do |fruit, index|
puts "#{index}: #{fruit}"
end
Output:
1: apple
2: banana
3: cherry
This flexibility makes each_with_index
a powerful tool in your Ruby programming toolkit.
Exploring each.with_index
On the other hand, each.with_index
is a method chain that combines the each
method with the with_index
method. This approach is a bit more functional and can be seen as more idiomatic in Ruby. Here’s how you can use it:
fruits = ["apple", "banana", "cherry"]
fruits.each.with_index do |fruit, index|
puts "#{index}: #{fruit}"
end
Output:
0: apple
1: banana
2: cherry
In this example, each
is called on the fruits
array, and then with_index
is chained to it. The block still takes two parameters: fruit
and index
, just like in the each_with_index
method. The output remains the same, providing the index alongside the value for each element in the array.
One of the advantages of using each.with_index
is that it can be more readable in certain contexts, especially when you’re chaining multiple methods together. This method allows for a clean and expressive style, particularly when combined with other Enumerable methods.
Additionally, each.with_index
can also accept a starting index just like each_with_index
. For example:
fruits.each.with_index(1) do |fruit, index|
puts "#{index}: #{fruit}"
end
Output:
1: apple
2: banana
3: cherry
This capability provides the same flexibility as each_with_index
, allowing you to start indexing from any number you choose.
Key Differences Between each_with_index and each.with_index
While both each_with_index
and each.with_index
achieve similar results, there are some key differences worth noting:
-
Syntax:
each_with_index
is a single method, whileeach.with_index
is a combination of two methods. This difference in syntax may influence your choice depending on your coding style or the complexity of your code. -
Readability: In scenarios where you are chaining multiple methods,
each.with_index
can provide better readability. It allows you to see the flow of data more clearly, making your code easier to understand at a glance. -
Performance: In most cases, the performance difference between the two methods is negligible. However, if you are working with large datasets, it’s always a good idea to benchmark your code to find the most efficient solution for your specific use case.
-
Flexibility: Both methods allow you to specify a starting index, but the chaining nature of
each.with_index
can make it easier to integrate into more complex method chains.
By understanding these differences, you can make an informed decision about which method to use based on your specific needs and coding style.
Conclusion
In conclusion, both each_with_index
and each.with_index
are valuable tools for iterating over arrays in Ruby. While they serve a similar purpose, their differences in syntax, readability, and flexibility can influence your choice depending on the context. By mastering these methods, you can enhance your Ruby programming skills and write more efficient, readable code. Whether you prefer the straightforward approach of each_with_index
or the functional style of each.with_index
, both methods will serve you well in your coding endeavors.
FAQ
-
What is the main purpose of each_with_index in Ruby?
each_with_index allows you to iterate over an array while keeping track of the index of each element. -
Can I specify a starting index when using each.with_index?
Yes, you can specify a starting index by passing an argument to the with_index method. -
Which method is better for chaining multiple methods together?
each.with_index is often considered better for chaining because it provides a more functional style. -
Is there any performance difference between the two methods?
Generally, the performance difference is negligible, but it’s best to benchmark for large datasets. -
Can I use each_with_index with other enumerable methods?
Yes, you can use each_with_index in conjunction with other enumerable methods, but it may not be as clear as using each.with_index in complex chains.