The continue Keyword in Ruby
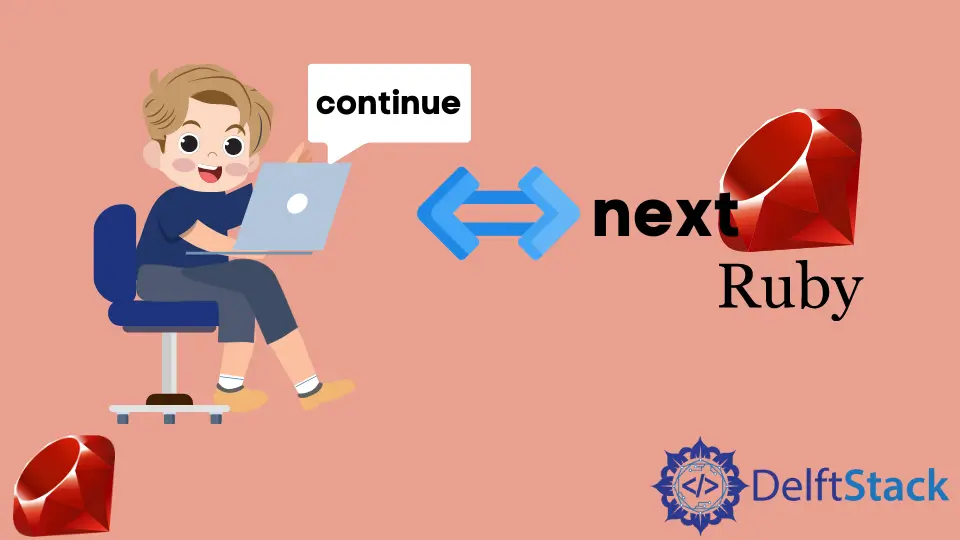
When diving into the world of programming, understanding control flow is essential. In many languages, the continue
keyword allows you to skip the current iteration of a loop and proceed to the next one. While Ruby doesn’t have a direct continue
keyword like C or Python, it offers elegant alternatives to achieve the same functionality.
In this article, we will explore these alternatives in Ruby, showcasing how you can effectively manage loop iterations. Whether you’re a seasoned Ruby developer or just starting out, understanding these concepts will enhance your coding skills and improve your code’s readability. Let’s uncover the Ruby way of handling loop control!
Using the Next Keyword
In Ruby, the closest equivalent to the continue
keyword is the next
keyword. This keyword can be used within loops to skip the current iteration and move to the next one. It’s straightforward and integrates seamlessly into your loop logic.
Here’s a simple example using the next
keyword in a for
loop:
for i in 1..5
next if i == 3
puts i
end
Output:
1
2
4
5
In this code, we loop through the numbers 1 to 5. When i
equals 3, the next
keyword is invoked, causing the loop to skip the puts i
statement for that iteration. As a result, the number 3 is not printed. This method is particularly useful when you want to filter out specific values without complicating your loop logic. The next
keyword helps keep your code clean and readable, allowing you to focus on the core functionality without unnecessary complexity.
Using an If Statement
Another way to achieve similar functionality in Ruby is by using an if
statement to control the flow of your loop. While this method may not be as concise as using next
, it provides clear logic that many developers may find easier to understand.
Here’s an example demonstrating this approach:
for i in 1..5
if i == 3
next
end
puts i
end
Output:
1
2
4
5
In this example, we again loop through the numbers 1 to 5. The if
statement checks if i
is equal to 3. If it is, the loop skips the puts i
line, effectively achieving the same result as the previous example. This method can be beneficial when you have multiple conditions to check, as it allows you to embed more complex logic within the loop. However, it can lead to slightly more verbose code compared to using next
, so it’s essential to consider the readability and maintainability of your code when choosing this approach.
Using the Until Keyword
Ruby also offers the until
keyword, which can be used to create loops that continue until a specified condition becomes true. While not a direct substitute for continue
, it can be structured to skip iterations based on conditions.
Here’s how you can use until
to achieve similar results:
i = 0
until i == 5
i += 1
next if i == 3
puts i
end
Output:
1
2
4
5
In this example, we initialize i
to 0 and increment it until it reaches 5. Within the loop, we use next
to skip the iteration when i
equals 3. This method showcases how you can control loop execution using different constructs in Ruby. The until
keyword can be especially useful when the looping condition is more naturally expressed in terms of “until” rather than “while.” This flexibility in Ruby allows developers to choose the most readable and intuitive approach for their specific use case.
Conclusion
Understanding how to control loop iterations is crucial for writing efficient and effective Ruby code. While Ruby doesn’t have a continue
keyword like some other programming languages, it provides several powerful alternatives, such as next
, if
statements, and the until
loop. Each of these methods allows you to manage your loop flow in a way that keeps your code clean and readable. By mastering these techniques, you’ll enhance your programming toolkit and be better equipped to tackle a wide range of coding challenges. Happy coding!
FAQ
-
What is the equivalent of the continue keyword in Ruby?
The equivalent of the continue keyword in Ruby is the next keyword, which allows you to skip the current iteration of a loop. -
Can I use an if statement to achieve similar functionality?
Yes, you can use an if statement to control the flow of your loop and skip iterations based on specific conditions. -
What is the purpose of the until keyword in Ruby?
The until keyword creates loops that continue until a specified condition becomes true, providing another way to control loop execution. -
How does using next improve code readability?
Using next helps keep your code concise and focused, eliminating unnecessary complexity and making it easier to understand the loop’s intent. -
Are there any performance considerations when using these methods?
Generally, using next or if statements has minimal performance impact. However, always choose the method that enhances code clarity and maintainability.