How to Remove an Array Element in Ruby
-
Remove an Array Element Using the
reject
Method - Remove an Array Element Using Array Subtraction
-
Remove an Array Element Using the
delete
Method -
Remove an Array Element Using the
delete_if
Method -
Remove an Array Element Using the
reject!
Method - Conclusion
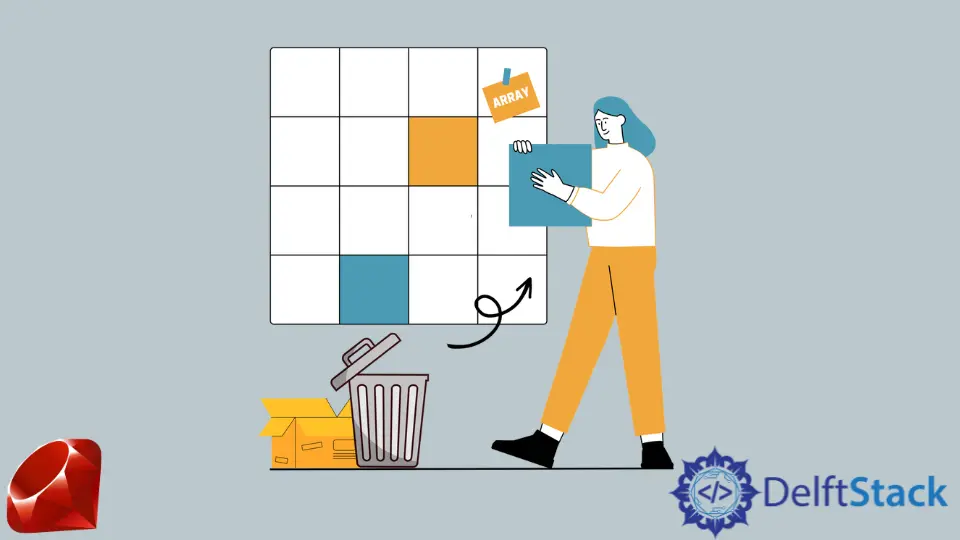
In Ruby programming, efficiently removing elements from an array is a common task. Good thing there are various methods available in Ruby that can be used to remove an element from an array.
Listed below are the different ways of removing an element from an array in Ruby. These diverse approaches cater to different scenarios, providing Ruby developers with multiple options for efficient array manipulation.
Remove an Array Element Using the reject
Method
The reject
method in Ruby is an enumerable method used to filter elements in a collection, such as an array or a hash, based on a specified condition. It returns a new collection containing only the elements for which the block evaluates to false
.
In other words, it excludes elements that satisfy the given condition. The reject
method does not modify the original collection; instead, it produces a new one with the filtered elements.
Example:
numbers = [2, 4, 6, 8, 10]
numbers_without_six = numbers.reject { |n| n == 6 }
puts numbers_without_six
Output:
2
4
8
10
In this code, we have an array called numbers
containing the elements 2, 4, 6, 8,
and 10
. The goal is to create a new array called numbers_without_six
that excludes the element with the value 6
.
We achieve this using the reject
method, which iterates through each element of the original array and rejects those that satisfy the given block condition. Here, the block checks if the element is equal to 6
, and if so, it is excluded from the new array.
The output will be the elements 2, 4, 8,
and 10
, as 6
has been successfully removed from the original array.
Remove an Array Element Using Array Subtraction
An array can be subtracted from another array using the minus -
operator in Ruby. For example, given that A
and B
are arrays, A - B
takes out all elements of B
found in A
and returns the remaining elements of A
.
We can rewrite the above example as done below to use this method.
Example:
numbers = [2, 4, 6, 8, 10]
numbers_without_six = numbers - [6]
puts numbers_without_six
Output:
2
4
8
10
In this code, we begin with an array called numbers
. Our objective is to create a new array named numbers_without_six
that excludes the element with the value 6
.
To achieve this, we use the subtraction operator (-
) along with an array containing only the element 6
. This operation effectively removes any elements present in the second array from the first.
Consequently, the new array, numbers_without_six
, is generated, and we print its contents. As a result, the output will be the elements 2, 4, 8,
and 10
, as the element 6
has been successfully subtracted from the original array.
Remove an Array Element Using the delete
Method
The delete
method in Ruby is used to remove elements from an array by specifying a particular value to be deleted. It modifies the original array by removing all occurrences of the specified value.
The method takes one argument, representing the value to be deleted, and returns the modified array.
Example:
numbers = [2, 4, 6, 8, 10]
numbers.delete(6)
puts numbers
Output:
2
4
8
10
In this code, we start with an array named numbers
. Our goal is to remove the element with the value 6
from this array.
To accomplish this, we use the delete
method, specifying the value 6
as the argument. The delete
method searches for and removes all occurrences of the specified value in the array.
Subsequently, we print the modified array. The output will be the elements 2, 4, 8,
and 10
, with the element 6
successfully deleted from the original array.
Remove an Array Element Using the delete_if
Method
There is also a delete_if
method that deletes an element that matches a condition from an array.
The delete_if
method in Ruby is an enumerable method applied to arrays. It removes elements from the array that satisfy a specified condition provided in a block.
The method iterates through the array, deleting elements for which the block evaluates to true
.
Example:
numbers = [2, 4, 6, 8, 10]
numbers.delete_if { |n| n == 6 }
puts numbers
Output:
2
4
8
10
In the code above, we initialize an array named numbers
. Our objective is to remove all occurrences of the value 6
from this array.
To achieve this, we employ the delete_if
method along with a block. The block iterates through each element of the array, and we use a condition to check if the element is equal to 6
and elements satisfying this condition are deleted from the array.
Subsequently, we print the modified array. As a result, the output will be the elements 2, 4, 8,
and 10
, with all instances of the value 6
successfully removed from the original array.
Remove an Array Element Using the reject!
Method
The reject!
method in Ruby is a destructive enumerable method applied to arrays. It removes elements from the array based on a specified condition provided in a block.
Unlike reject
, reject!
modifies the original array in place, removing elements that satisfy the given condition. If no elements are removed, it returns nil
.
Example:
numbers = [2, 4, 6, 8, 10]
numbers.reject! { |n| n == 6 }
puts numbers
Output:
2
4
8
10
In this code, we use the reject!
method along with a block. The block iterates through each element of the array, checking if the element is equal to 6
.
Elements meeting this condition are rejected, meaning they are removed from the array. Following the modification, we print the updated array.
As a result, the output will be the elements 2, 4, 8,
and 10
, with the element 6
successfully rejected from the original array.
Conclusion
This article presented several methods for efficiently removing elements from an array in Ruby. The reject
method filters elements based on a condition, array subtraction uses the -
operator to exclude specified elements, and the delete
method removes a specific value, modifying the original array.
Additionally, both the delete_if
and reject!
methods delete elements based on a condition, with the latter modifying the array in place. These diverse approaches cater to various scenarios, offering flexibility and options for developers in handling array modifications in Ruby.