How to Get Maximum and Minimum Number in a Ruby Array
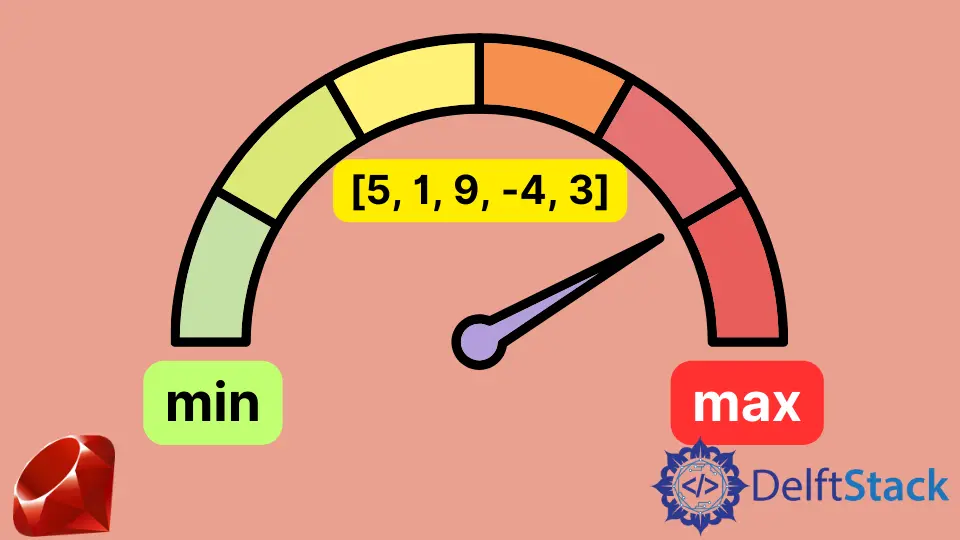
When working with arrays in Ruby, finding the maximum and minimum numbers is a common task that developers encounter frequently. Whether you’re analyzing data, performing calculations, or simply looking to extract specific values, understanding how to efficiently retrieve these numbers can streamline your coding process. Ruby provides several elegant methods to achieve this, making it a powerful tool for developers of all skill levels.
In this article, we’ll explore different techniques to find the maximum and minimum values in an array, complete with code examples and detailed explanations. Let’s dive in!
Using the max and min Methods
Ruby offers built-in methods that make finding the maximum and minimum values in an array incredibly straightforward. The max
and min
methods are specifically designed for this purpose.
Here’s how you can use them:
numbers = [3, 5, 1, 8, 2]
max_value = numbers.max
min_value = numbers.min
puts "Maximum value: #{max_value}"
puts "Minimum value: #{min_value}"
Output:
Maximum value: 8
Minimum value: 1
In this example, we start by defining an array called numbers
. We then call the max
method on this array to retrieve the highest value, which is stored in the variable max_value
. Similarly, we use the min
method to find the lowest value, storing it in min_value
. Finally, we print both values to the console.
Using these built-in methods is highly efficient and requires minimal code. They are particularly useful when you need quick access to the maximum or minimum values without additional complexity. This approach is not only easy to read but also leverages Ruby’s internal optimizations for performance.
Using the sort Method
Another way to find the maximum and minimum values in a Ruby array is by using the sort
method. This method sorts the entire array, allowing you to easily access the first and last elements.
Here’s how it works:
numbers = [3, 5, 1, 8, 2]
sorted_numbers = numbers.sort
max_value = sorted_numbers[-1]
min_value = sorted_numbers[0]
puts "Maximum value: #{max_value}"
puts "Minimum value: #{min_value}"
Output:
Maximum value: 8
Minimum value: 1
In this case, we first sort the numbers
array using the sort
method. This rearranges the elements in ascending order. The maximum value can then be found at the last index of the sorted array, while the minimum value is at the first index. We store these values in max_value
and min_value
, respectively, and print them.
While this method is straightforward, it’s worth noting that sorting the array can be less efficient than using the max
and min
methods, particularly for larger datasets. Sorting involves additional computational overhead, so it’s best used when you need the sorted array for other purposes as well.
Using Enumerable Module
Ruby’s Enumerable module provides additional methods that can be utilized to find the maximum and minimum values in an array. Specifically, the max_by
and min_by
methods allow for more complex comparisons, which is particularly useful for arrays of objects.
Here’s an example:
numbers = [3, 5, 1, 8, 2]
max_value = numbers.max_by { |num| num }
min_value = numbers.min_by { |num| num }
puts "Maximum value: #{max_value}"
puts "Minimum value: #{min_value}"
Output:
Maximum value: 8
Minimum value: 1
In this example, we use the max_by
and min_by
methods. These methods require a block, which allows you to specify the criteria for determining the maximum or minimum. In this case, the block simply returns the number itself, which is sufficient for our integer array.
This method is particularly powerful when dealing with more complex data structures, such as arrays of hashes or objects. You can customize the block to extract specific attributes for comparison, making it a versatile tool in your Ruby programming toolkit.
Conclusion
Finding the maximum and minimum numbers in a Ruby array is a fundamental skill that can enhance your coding efficiency. Whether you choose to use the built-in max
and min
methods, the sort
method, or the Enumerable
module, Ruby provides you with several elegant solutions. Each method has its own strengths and ideal use cases, so understanding these options allows you to choose the best approach for your specific needs. With these techniques under your belt, you’ll be well-equipped to handle array manipulations in Ruby with confidence.
FAQ
-
How do I find the maximum value in a Ruby array?
You can use themax
method on the array to find the maximum value easily. -
Is there a way to find both maximum and minimum values in one go?
No, Ruby does not provide a built-in method to find both values simultaneously, but you can callmax
andmin
in succession. -
Can I find the maximum and minimum values in an array of strings?
Yes, themax
andmin
methods can be used on arrays of strings, and they will compare the strings based on their lexicographical order. -
What is the performance difference between using
sort
andmax/min
?
Usingmax
andmin
is generally more efficient thansort
, especially for large arrays, as sorting involves additional computational overhead. -
Can I use max_by and min_by for complex data structures?
Yes,max_by
andmin_by
can be used with arrays of objects or hashes, allowing you to specify custom criteria for comparison.