How to Filter an Array in Ruby
Nurudeen Ibrahim
Feb 02, 2024
-
Filter an Array Using the
select
Method in Ruby -
Filter an Array Using the
reject
Method in Ruby -
Filter an Array Using the
inject
Method in Ruby
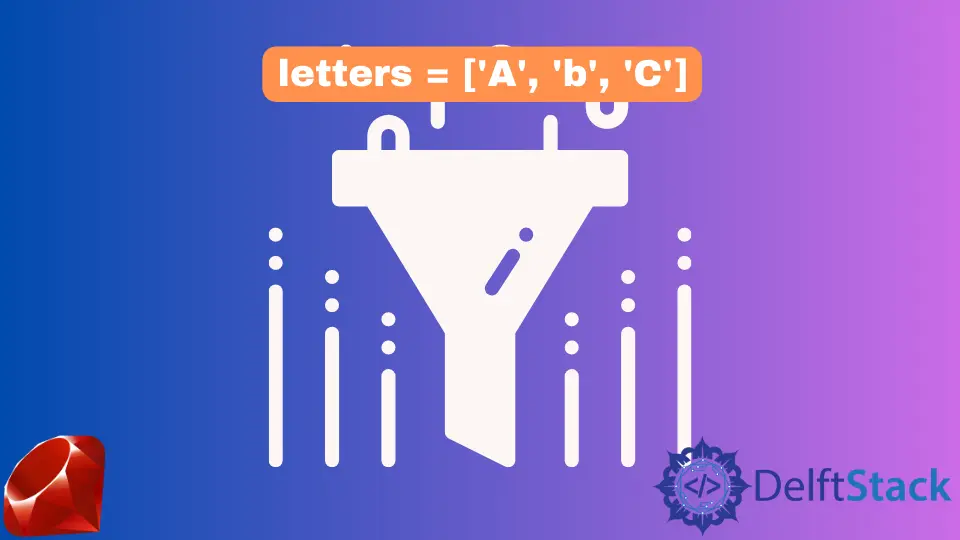
The following topic is the methods used to filter an array in Ruby.
Filter an Array Using the select
Method in Ruby
This is a very common method of filtering in Ruby. It’s useful when selecting a few elements from the array.
letters = %w[A k L B n O P c r]
small_letters = letters.select { |l| l == l.downcase }
puts small_letters
Output:
k
n
c
r
Filter an Array Using the reject
Method in Ruby
This is the opposite of select
. The ’ reject ’ method is preferred when many elements are taken from an array.
letters = %w[A k L B n O P C r]
small_letters = letters.reject { |l| l != l.downcase }
puts small_letters
Output:
k
n
r
Filter an Array Using the inject
Method in Ruby
The inject
method is usually used to accumulate all the elements of an array but can filter by setting the initial accumulator to an empty array.
letters = %w[A k L B n O P c r]
small_letters = letters.each_with_object([]) do |l, result|
result << l if l == l.downcase
end
puts small_letters
Output:
k
n
q
r