How to Check if Value Exists in Array in Ruby
-
Use the
include?
Method to Check if Value Exists in Ruby Array -
Use the
any?
Method to Check if Value Exists in Ruby Array -
Use the
index
Method to Check if Value Exists in Ruby Array -
Use the
member?
Method to Check if Value Exists in Ruby Array -
Use the
find
Method to Check if Value Exists in Ruby Array - Conclusion
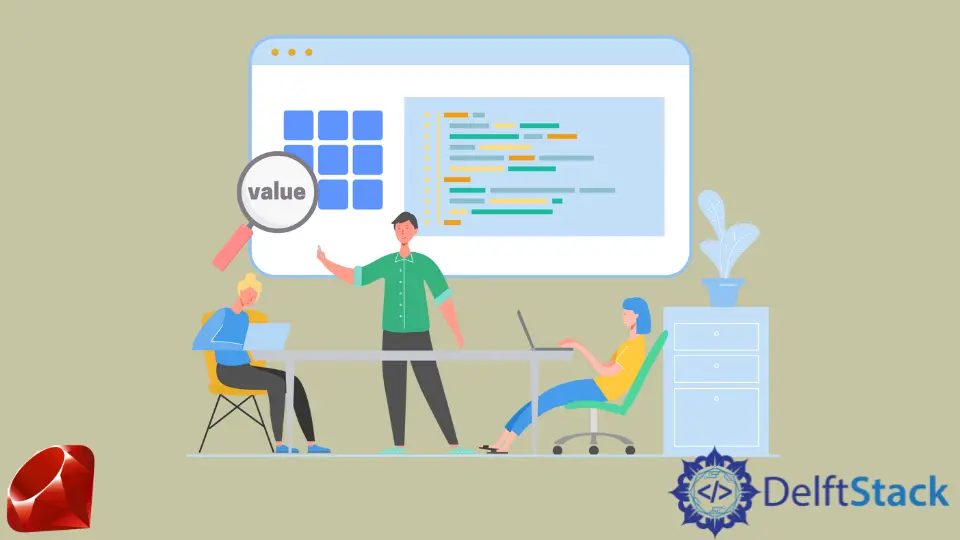
In Ruby, an array is a common data type. It contains elements of various data types, including number, string, Boolean, and even another array.
We may need to check if a value exists in a given array in some circumstances. This article shows how to do so using the array’s built-in methods.
Use the include?
Method to Check if Value Exists in Ruby Array
The include?
seems to be the most straightforward way to check if a value resides within an array. If an element is in the array, include?
returns true
; otherwise, false
.
Example:
print(%w[ruby sapphire amber].include?('ruby'))
print("\n")
print(%w[ruby sapphire amber].include?('moonstone'))
Output:
true
false
In the code, we are using the print
method along with the include?
method to check if specific values exist in a Ruby array. The array, %w[ruby sapphire amber]
, contains three elements.
In the first line, we check if the array includes the value 'ruby'
using the include?('ruby')
method. Since 'ruby'
is indeed present in the array, the first print
statement outputs true
.
In the second line, we check for the existence of 'moonstone'
using include?('moonstone')
. As 'moonstone'
is not part of the array, the second print
statement outputs false
.
Use the any?
Method to Check if Value Exists in Ruby Array
We could also use any?
instead of include?
to see if an array contains a value. Because any?
accepts a block, it’s ideal for complex checking logic.
It returns true
if the block returns a value other than false
or nil
.
Example:
print(%w[ruby sapphire amber].any? { |gem| gem == 'moonstone' })
print("\n")
print(%w[ruby sapphire amber].any? { |gem| gem == 'ruby' })
Output:
false
true
In the code example above, we use the any?
method in Ruby to determine the presence of specific values within an array. The array %w[ruby sapphire amber]
consists of three elements.
In the first line, we employ any?
with a block to check if any element in the array is equal to 'moonstone'
. Since 'moonstone'
is not present in the array, the first print
statement outputs false
.
In the second line, we check if any element matches the value 'ruby'
within the array. As 'ruby'
is indeed an element of the array, the second print
statement outputs true
.
Moving on, we will have another example using the any?
method.
The code below will use any?
to see if the array contains any gems with a size greater than four.
Example:
print(%w[ruby sapphire amber].any? { |gem| gem.size > 4 })
Output:
true
In this code, we check if any element within the array %w[ruby sapphire amber]
has a size greater than 4
. Using a block with the condition gem.size > 4
, we iterate through each element in the array to evaluate the size.
The any?
method returns true
if at least one element satisfies the condition. In this case, both 'sapphire'
and 'amber'
have sizes greater than 4
, so the print
statement outputs true
.
Use the index
Method to Check if Value Exists in Ruby Array
Another way to check if a value exists in a Ruby array is by using the index
method.
This method either takes a single value, as include?
does, or a block. The index
method returns the matching value’s index; otherwise returns nil
, unlike include?
and any?
, which return Boolean.
Example:
print(%w[ruby sapphire amber].index { |gem| gem == 'ruby' })
print("\n")
print(%w[ruby sapphire amber].index { |gem| gem.size > 10 })
Output:
0
In this code, we use the index
method in Ruby to find the index of specific values within the array %w[ruby sapphire amber]
.
In the first line, we use index
with a block to check if any element in the array is equal to 'ruby'
. Since 'ruby'
is the first element in the array, the print
statement outputs 0
.
In the second line, we’ll find the index of the first element with a size greater than 10
. None of the elements in the array satisfy this condition, so the print
statement outputs nothing.
Use the member?
Method to Check if Value Exists in Ruby Array
The member?
method is similar to include?
and can also be used to check if a value exists in an array. Both methods are used to check whether a particular element is present in an array.
Note: The
member?
method is a member of theEnumerable
module, which means it can be used not only with arrays but also with other enumerable objects.
Example:
array = %w[ruby sapphire amber]
value_to_check = 'ruby'
puts array.member?(value_to_check) ? 'Value exists' : 'Value does not exist'
Output:
Value exists
In this code, we have an array %w[ruby sapphire amber]
and a value to check, which is 'ruby'
. Using the member?
method in Ruby, we check if the specified value, 'ruby'
, exists in the array.
The member?
method returns true
if the value is present and false
otherwise. In this case, as 'ruby'
is an element in the array, the ternary operator is evaluated to true
, and the puts
statement outputs Value exists
.
Use the find
Method to Check if Value Exists in Ruby Array
In Ruby, the find
method is not typically used for checking the existence of a value in an array. Instead, it is primarily employed for searching and returning the first element in an array that satisfies a given condition.
While this is the case, you can still adapt it to check if a specific value exists in an array. However, it’s important to note that using find
for this purpose might not be the most idiomatic or efficient approach, and other methods like include?
or member?
are generally more appropriate.
Example:
array = %w[ruby sapphire amber]
puts array.find { |gem| gem.size > 10 } ? 'Value exists' : 'Value does not exist'
Output:
Value does not exist
In this code, we have an array %w[ruby sapphire amber]
. We use the find
method in Ruby along with a block to search for an element with a size greater than 10
within the array.
The find
method returns the first element that meets the given condition. In this case, none of the gems in the array have a size greater than 10
, so the find
method returns nil
.
As a result, the ternary operator evaluates to false
, and the puts
statement outputs Value does not exist
.
Conclusion
This article has explored various methods in Ruby for checking if a value exists in an array. Whether using the straightforward include?
method, the versatile any?
method for complex logic, or the index
, member?
, and find
methods, Ruby offers multiple approaches to suit different scenarios.
Choose the method that best fits your specific use case for efficient value checking in Ruby arrays.