How to Toggle Classes in ReactJS
- Understanding Class Toggling in ReactJS
- Using Conditional Rendering for Class Toggling
- Leveraging CSS Modules for Class Toggling
- Conclusion
- FAQ
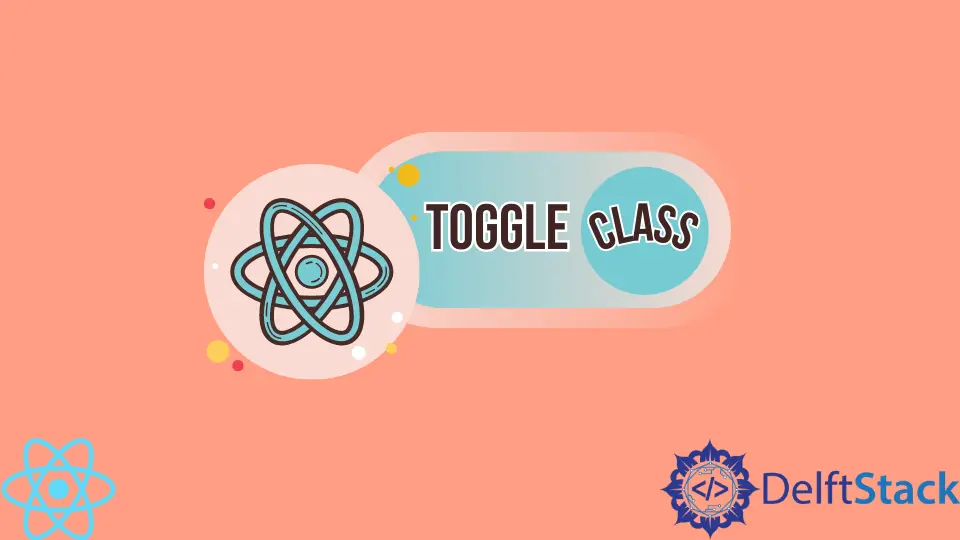
Toggling classes in ReactJS is a fundamental skill that can significantly enhance the interactivity of your web applications. Whether you’re creating a simple toggle button or implementing a more complex UI component, understanding how to manage and manipulate CSS classes dynamically is crucial.
In this article, we’ll explore different methods to toggle classes in ReactJS through practical examples. By the end, you’ll be equipped with the knowledge to implement class toggling effectively in your own projects. Let’s dive in and discover how to make your React components more engaging and user-friendly!
Understanding Class Toggling in ReactJS
In React, managing the state of your components is the key to toggling classes effectively. The primary approach involves using the built-in useState
hook to track whether a particular class should be applied. This allows you to conditionally render classes based on the component’s state.
Here’s a simple example that demonstrates the concept. We’ll create a button that toggles a class on a div element when clicked.
Example Code
import React, { useState } from 'react';
const ToggleClassExample = () => {
const [isActive, setIsActive] = useState(false);
const toggleClass = () => {
setIsActive(!isActive);
};
return (
<div className={isActive ? 'active' : 'inactive'}>
<p>This is a toggleable class example.</p>
<button onClick={toggleClass}>Toggle Class</button>
</div>
);
};
export default ToggleClassExample;
Output:
This is a toggleable class example.
In this example, we define a functional component called ToggleClassExample
. We use the useState
hook to create a state variable, isActive
, which determines whether the class active
or inactive
is applied to the div. The toggleClass
function updates this state when the button is clicked, thus toggling the class.
Using Conditional Rendering for Class Toggling
Another effective method for toggling classes in React is through conditional rendering. This approach can be particularly useful when you have multiple classes that need to be toggled based on various conditions.
Let’s look at an example where we toggle between multiple classes based on different states.
Example Code
import React, { useState } from 'react';
const MultiClassToggle = () => {
const [status, setStatus] = useState('inactive');
const toggleStatus = () => {
setStatus(prevStatus => (prevStatus === 'inactive' ? 'active' : 'inactive'));
};
return (
<div className={status}>
<p>The current status is: {status}</p>
<button onClick={toggleStatus}>Toggle Status</button>
</div>
);
};
export default MultiClassToggle;
Output:
The current status is: inactive
In this example, we define a state variable status
that can either be active
or inactive
. The toggleStatus
function switches the value of status
between these two states. The class applied to the div changes dynamically based on the current value of status
, showcasing how conditional rendering can be leveraged for class toggling in React.
Leveraging CSS Modules for Class Toggling
CSS Modules provide a scoped way to manage CSS classes in React applications. When using CSS Modules, class names are automatically scoped locally, which helps prevent conflicts. You can still toggle classes using the same state management techniques but with the added benefit of modular CSS.
Here’s how you can implement class toggling with CSS Modules.
Example Code
import React, { useState } from 'react';
import styles from './Toggle.module.css';
const CssModuleToggle = () => {
const [isActive, setIsActive] = useState(false);
const toggleClass = () => {
setIsActive(!isActive);
};
return (
<div className={isActive ? styles.active : styles.inactive}>
<p>This is a CSS Modules toggle example.</p>
<button onClick={toggleClass}>Toggle Class</button>
</div>
);
};
export default CssModuleToggle;
Output:
This is a CSS Modules toggle example.
In this example, we import styles from a CSS module file named Toggle.module.css
. The active
and inactive
classes are applied based on the isActive
state. This method not only keeps your styles organized but also prevents class name collisions, making your React applications more maintainable.
Conclusion
Toggling classes in ReactJS is an essential technique for creating dynamic, interactive user interfaces. By leveraging state management and conditional rendering, you can easily apply and switch classes based on user interactions. Whether you choose to use standard CSS, CSS Modules, or other styling solutions, mastering class toggling will undoubtedly enhance your React development skills. As you continue to build more complex applications, remember that effective class management is key to delivering a seamless user experience.
FAQ
- How do I toggle multiple classes in React?
You can toggle multiple classes using an array and thejoin
method to combine them based on your component’s state.
-
Can I use inline styles instead of classes in React?
Yes, you can use inline styles directly in your components, but toggling classes is often more manageable for larger applications. -
What are CSS Modules in React?
CSS Modules are a way to style React components with locally scoped class names, preventing conflicts with other styles in your application. -
How can I style a component based on its state?
You can use theuseState
hook to manage the component’s state and conditionally apply classes or styles based on that state. -
Is it possible to toggle classes without using state?
While it is possible to manipulate classes directly using refs or DOM methods, using state is the recommended approach in React for better reactivity and performance.
Shraddha is a JavaScript nerd that utilises it for everything from experimenting to assisting individuals and businesses with day-to-day operations and business growth. She is a writer, chef, and computer programmer. As a senior MEAN/MERN stack developer and project manager with more than 4 years of experience in this sector, she now handles multiple projects. She has been producing technical writing for at least a year and a half. She enjoys coming up with fresh, innovative ideas.
LinkedIn