How to Use Multiple Class Names in React
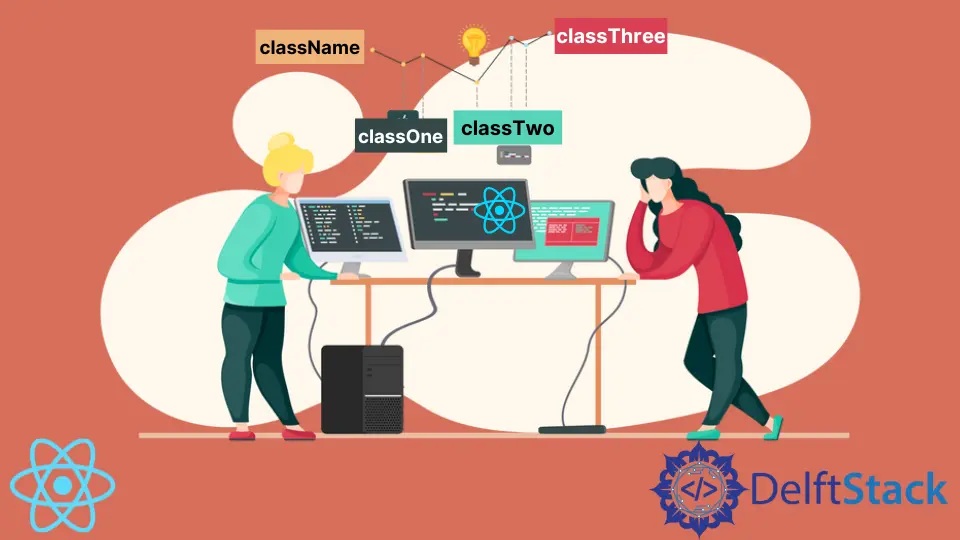
When developing complex applications, it is not unusual to have HTML elements with multiple classes. If you’re familiar with HTML and CSS, you’re probably familiar with the syntax to set multiple classes.
In this article, we’ll look at multiple different ways to do this in React.
Multiple Class Names in React
Most React developers use JSX, which is a special syntax for writing simple component definitions. Its syntax is very similar to HTML, which is why it is preferred by most React developers. There are a few essential differences that concern setting classes.
In HTML, you are probably used to setting classes using the class
attribute. Since React components can be defined as an instance of a class, Class is a reserved word. So, developers must use the className
attribute instead.
In JSX, className
attributes must be set equal to strings. A simple definition with multiple classes would look like this:
<h1 className="classOne classTwo">Hi! Try edit me</h1>
Alternatively, the className
attribute can be set equal to a JavaScript expression that returns a string. To ensure that the expressions are correctly interpreted as JavaScript code, you must place them between curly braces. Let’s take a look at an example:
const color = "white"
<h1 className={"classOne " + color }>Hi! Try edit me</h1>;
In this example, we add a regular string and a string value contained within a variable. Once the expression is evaluated, our className
attribute will equal the 'classOne white'
string.
We can make the expression more readable by replacing the +
operator with a .concat()
method, which combines two strings into one.
const color="white"
h1 className={"classOne ".concat(color)}>Hi! Try edit me</h1>
React .join()
Method
This standard JavaScript method is useful for transforming an array of string values into multiple className
values. Since it is a JavaScript method, we need to place an entire expression between curly braces in JSX.
By default, the combined string values will be separated by a comma. However, to produce a valid list of className
values, we need to separate them by a single space. Fortunately, we can specify the separator by passing a single space string ' '
as the argument to the method. Here’s an example:
class App extends Component {
render() {
const arr = ["bold", "primary", "lead"]
return (<div><p className={arr.join(" ")}>Hi! Try edit me</p></div>)
}
}
In our styles.css
file on playcode, we defined the three classes:
.lead {
font-size: 40px;
}
.bold {
font-weight: bold;
}
.primary {
color: white
}
Once the code within the curly braces is executed, you can see that all three classes are applied.
You can also look into the source code to verify that the classes are applied to the <p>
element.
<div id="app">
<div class="container black">
<p class="bold primary lead">Hi! Try edit me</p>
</div>
</div>
Custom Function in React
In ES6, we can use array destructuring syntax to create an elegant custom function that returns a formatted string that we can use as a className
value.
Let’s look at the code:
class App extends Component {
render() {
const classNameGenerator = (...classes)=>{
return classes.join(" ")
}
return (<div className={classNameGenerator("container")}></div>)
}
}
This function treats its arguments as array items and then uses the .join()
method to return a formatted list of className
values.
When using the function, you can pass as many string values as you’d like.
Conditional className
in React
Applying the className
values conditionally can be useful for changing an element’s appearance based on user input. For instance, if your application has dark and light themes, its background should also toggle from light to darker shades. Here’s a code example:
const lightBackground = !this.state.darkTheme ? "white" : "black"
<div className={classNameGenerator("container") + " " + lightBackground }></div>
The lightBackground
variable gets assigned one of the two strings based on the darkTheme
state property value. In addition to calling classNameGenerator("")
function, we also use the +
operator to include the conditional value of the variable as well.
For better demonstration, our playcode application includes a button for toggling the boolean
value of the darkTheme
property.
Irakli is a writer who loves computers and helping people solve their technical problems. He lives in Georgia and enjoys spending time with animals.
LinkedIn