How to Add Class Active in React
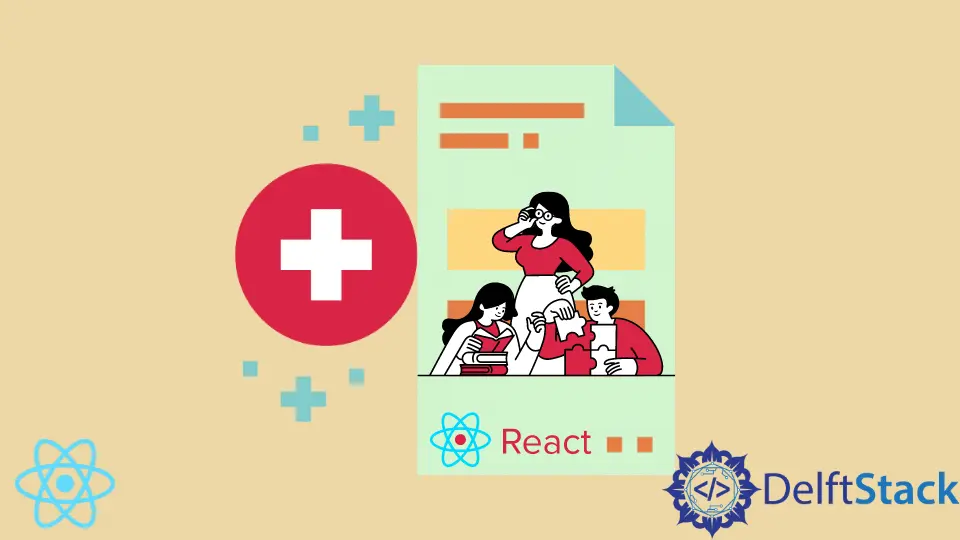
To cause only one classNames
to be active when building apps with the React framework. Once we attempt to make a classNames
active, the other classNames
become active.
We will now discuss in this article how to solve this situation.
the classNames
Attributes in React
We use class
in HTML as a reference when we want to style the webpage with CSS, but we cannot use a class
in React because the word is reserved for a different function. This is where classNames
become useful as a replacement for classes in React.
classNames
are useful when we want to create a specific function for a particular component, as we will see with the button classNames
example.
Let us picture a group of buttons with the color green. When none of these buttons are clicked, they remain green, but it turns red when one button is clicked, meaning it is active.
We’ll discuss in this tutorial how to make the clicked button turn blue without the rest of the buttons turning blue by adding an active class to a button.
Add Active to a Button classNames
in React
Start by creating a new web project, npx create-react-app examplefour
. Then move on to the app.js
to lay up the following codes.
Code - App.js
:
import React, { useState } from "react";
import styled from "styled-components";
const theme = {
blue: {
default: "#3f51b5",
hover: "#283593"
},
pink: {
default: "#e91e63",
hover: "#ad1457"
}
};
const Button = styled.button`
background-color: ${(props) => theme[props.theme].default};
color: white;
padding: 5px 15px;
border-radius: 5px;
outline: 0;
text-transform: uppercase;
margin: 10px 0px;
cursor: pointer;
box-shadow: 0px 2px 2px lightgray;
transition: ease background-color 250ms;
&:hover {
background-color: ${(props) => theme[props.theme].hover};
}
&:disabled {
cursor: default;
opacity: 0.7;
}
`;
Button.defaultProps = {
theme: "blue"
};
function clickMe() {
alert("You clicked me!");
}
const ButtonToggle = styled(Button)`
opacity: 0.7;
${({ active }) =>
active &&
`
opacity: 1;
`}
`;
const Tab = styled.button`
padding: 10px 30px;
cursor: pointer;
opacity: 0.6;
background: white;
border: 0;
outline: 0;
border-bottom: 2px solid transparent;
transition: ease border-bottom 250ms;
${({ active }) =>
active &&
`
border-bottom: 2px solid black;
opacity: 1;
`}
`;
const types = ["Cash", "Credit Card", "Bitcoin"];
function ToggleGroup() {
const [active, setActive] = useState(types[0]);
return (
<div>
{types.map((type) => (
<ButtonToggle active={active === type} onClick={() => setActive(type)}>
{type}
</ButtonToggle>
))}
</div>
);
}
export default function App() {
return (
<>
<ToggleGroup />
</>
);
We use React hooks to track which button is active when clicked, called the ToggleGroup
and ButtonToggle
functions. Then, with the onClick
event listener function being set to active, once one of the buttons is clicked, only the classNames
attached to that button becomes active.
Then we added some styling to differentiate the clicked button from the inactive ones. Next, we do a bit of coding in the index.js
like in the following snippet.
Code - index.js
:
import React from "react";
import ReactDOM from "react-dom";
import App from "./App";
const rootElement = document.getElementById("root");
ReactDOM.render(<App />, rootElement);
Output:
Conclusion
An attempt by a user to make a single button active from the rest of the buttons can prove a very stubborn task. Applying the ToggleGroup
function alongside the onClick
event listener is one smooth way to get past the situation.
Fisayo is a tech expert and enthusiast who loves to solve problems, seek new challenges and aim to spread the knowledge of what she has learned across the globe.
LinkedIn