How to Dynamically Apply className Values in React
-
Set Dynamic
className
Values in React -
Use Native JavaScript to Set Dynamic
className
Values in React -
Use String Literals (ES6) to Set Dynamic
className
Values in React
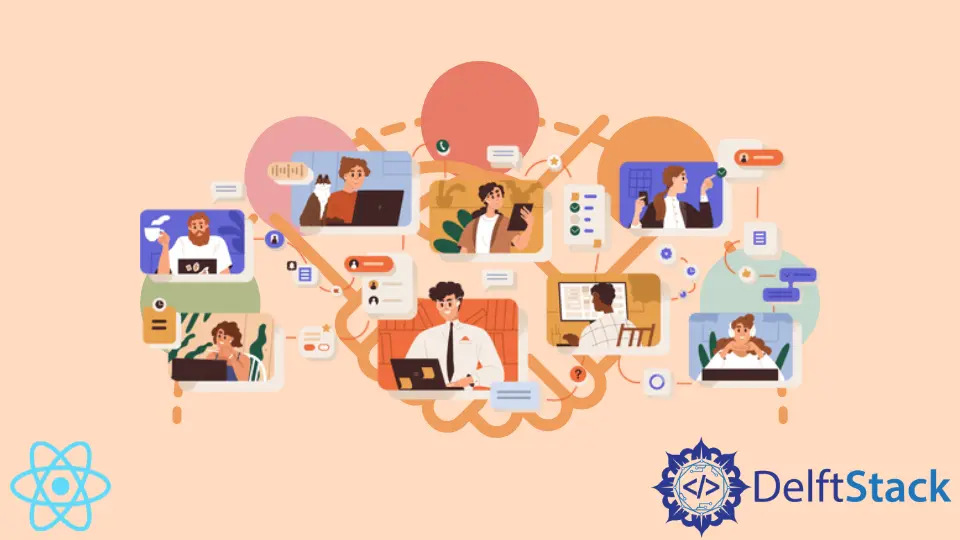
Modern users set high expectations when it comes to customer experience. They want features like dark mode and intuitive interfaces that respond to their actions.
Web developers need to find a way to dynamically update classes and styles depending on user actions to implement these features.
The className
attribute will contain multiple values for advanced applications and containers. If you want to set multiple values to a className
but only one of the className
values is dynamic, you can do that in various ways.
Set Dynamic className
Values in React
In React, we use the className
attribute to add classes to our elements. The className
attribute will contain multiple values when building complex web applications.
When building React applications, we use the templating language JSX, which allows JavaScript expressions within the return
statement. All you have to do is wrap JavaScript expressions with curly brackets {}
, and everything between these brackets will be evaluated.
You can set multiple values to the className
attribute. Some of them will be constant, while others will be applied dynamically.
Let’s explore specific ways to do that in React.
Use Native JavaScript to Set Dynamic className
Values in React
The simplest way to add dynamic className
values is to combine curly braces and set the className
attributes equal to the state variables. This is a fairly simple feature available in all versions of JavaScript.
Example:
export default function App() {
const [border, setBorder] = useState("blackBorder");
return (
<div className={"App " + border}>
<h1>Hello CodeSandbox</h1>
<input type="text" onChange={(e) => setBorder(e.target.value)}></input>
</div>
);
}
In this example, we used the useState()
hook to set the default value of the border
state variable to blackBorder
. In CSS, we defined the blackBorder
class rules, which will apply a 2-pixel black border to any container.
Within the return
statement, we used the {}
curly braces syntax to have the two className
values. The App
class will be constant, whereas the second className
value will depend on the value of the border
state variable.
We can implement a text input to let users type in any className
, and it will update the value of the border
valuable. Check out the demo yourself and type in one of the following values: greenBorder
, blackBorder
, redBorder
and see what happens.
Use String Literals (ES6) to Set Dynamic className
Values in React
Alternatively, you can use backticks, also known as string literals, to define dynamic values for the className
attribute.
Example:
export default function App() {
const [border, setBorder] = useState("blackBorder");
return (
<div className={`App ${border}`}>
<h1>Hello CodeSandbox</h1>
<input type="text" onChange={(e) => setBorder(e.target.value)}></input>
</div>
);
}
This syntax is much more readable. Pay attention to the double use of curly braces.
The outside pair is necessary so that JSX understands that there is a JavaScript expression between curly braces.
When using backticks, the dollar sign denotes a reference to a variable. For instance, if you wrote ${someVariable}
, JavaScript would know to look up the current value of the variable.
Otherwise, everything is the same as before.
Irakli is a writer who loves computers and helping people solve their technical problems. He lives in Georgia and enjoys spending time with animals.
LinkedIn