How to Use CLSX to Conditionally Apply Classes in React
- What is CLSX?
- Setting Up CLSX in Your React Project
- Basic Usage of CLSX
- Advanced Usage with Arrays and Nested Objects
- Performance Considerations
- Conclusion
- FAQ
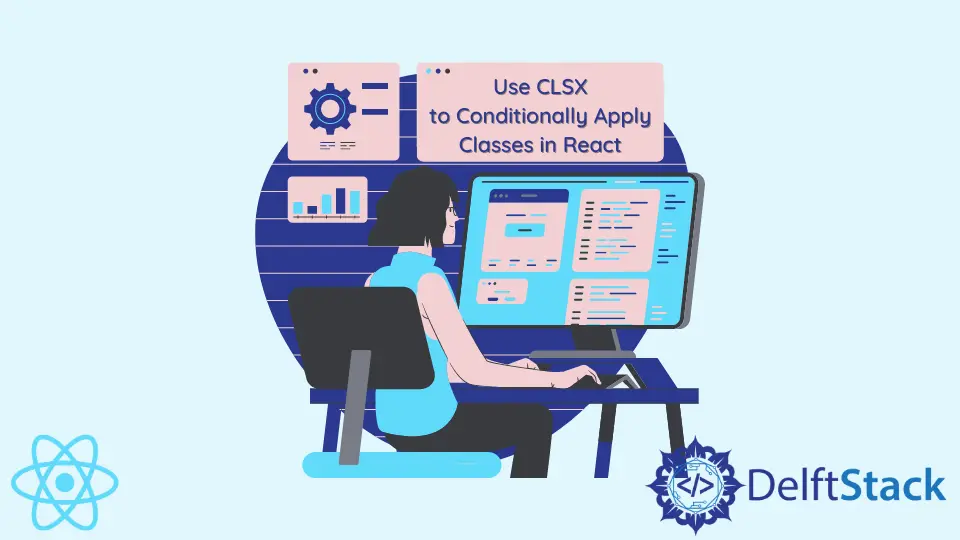
In the world of React, managing class names dynamically can sometimes feel like a daunting task. If you’ve ever found yourself tangled in a web of conditional statements just to apply classes based on certain conditions, you’re not alone. Enter CLSX, a lightweight utility that simplifies this process. The clsx()
function allows you to conditionally apply classes to your React components with remarkable ease and clarity.
In this article, we’ll explore how to effectively use CLSX to enhance your React applications, making your code cleaner and more maintainable. Whether you’re a beginner or an experienced developer, you’ll find practical examples and insights that will elevate your React development skills.
What is CLSX?
CLSX is a tiny utility for constructing className strings conditionally. Instead of using complex string concatenation or multiple ternary operators, CLSX provides a straightforward API that allows you to pass in various arguments, such as strings, objects, and arrays, to generate a single className string. This not only makes your code more readable but also helps to avoid potential bugs that can arise from complex conditional logic.
Setting Up CLSX in Your React Project
Before diving into examples, you need to install CLSX in your React project. You can easily do this using npm or yarn. Here’s how:
npm install clsx
or
yarn add clsx
Once installed, you can import CLSX into your components and start using it right away. The simplicity of integrating CLSX into your project is one of its many advantages, allowing you to focus on building your application without unnecessary complexity.
Basic Usage of CLSX
The most straightforward way to use CLSX is by passing strings directly to the clsx()
function. Here’s a basic example:
import clsx from 'clsx';
function Button({ primary, disabled }) {
const buttonClass = clsx('btn', {
'btn-primary': primary,
'btn-disabled': disabled,
});
return <button className={buttonClass}>Click Me</button>;
}
In this example, the Button
component accepts two props: primary
and disabled
. Depending on these props, CLSX constructs the appropriate class string. If primary
is true, the button will have the class btn-primary
. If disabled
is true, it will include btn-disabled
. This approach keeps your code clean and easy to read.
Output:
<button class="btn btn-primary">Click Me</button>
Using CLSX in this way not only simplifies class management but also enhances the readability of your components. You can easily see which classes will be applied based on the props, making it easier to understand the behavior of your components at a glance.
Advanced Usage with Arrays and Nested Objects
CLSX shines when dealing with more complex scenarios involving arrays and nested objects. Here’s an example that demonstrates this capability:
import clsx from 'clsx';
function Card({ isFeatured, isHighlighted }) {
const cardClass = clsx('card', {
'card-featured': isFeatured,
'card-highlighted': isHighlighted,
}, ['additional-class', { 'extra-class': isFeatured }]);
return <div className={cardClass}>This is a card</div>;
}
In this instance, the Card
component can accept multiple conditions and even additional classes through an array. If isFeatured
or isHighlighted
is true, the respective classes will be added. Additionally, an array containing additional-class
is always included, and extra-class
is conditionally added based on isFeatured
. This flexibility allows for highly customizable class management.
Output:
<div class="card card-featured additional-class extra-class">This is a card</div>
This advanced usage showcases the power of CLSX in managing dynamic class names effectively. You can easily combine static and dynamic classes, making your components more versatile and adaptable to different states.
Performance Considerations
When working with conditional classes in React, performance is an essential consideration. CLSX is designed to be efficient and lightweight, making it a great choice for performance-sensitive applications. The utility is optimized to handle class name construction without unnecessary overhead, ensuring your components render quickly.
Moreover, using CLSX can reduce the complexity of your components, leading to fewer re-renders and improved performance. By keeping your class management logic simple and clear, you can focus on building features rather than debugging class name issues.
Conclusion
Using CLSX to conditionally apply classes in React can significantly streamline your development process. Its straightforward API allows you to manage class names with ease, keeping your code clean and maintainable. By incorporating CLSX into your React projects, you can enhance your components’ readability and performance. Whether you’re building a simple button or a complex card component, CLSX is a powerful tool that every React developer should consider.
FAQ
-
What is CLSX used for?
CLSX is a utility for conditionally constructing className strings in React components. -
How do I install CLSX in my React project?
You can install CLSX using npm with the commandnpm install clsx
or with yarn usingyarn add clsx
. -
Can I use CLSX with arrays and objects?
Yes, CLSX allows you to pass arrays and objects to conditionally apply multiple classes based on various conditions. -
Is CLSX performance-friendly?
Yes, CLSX is designed to be lightweight and efficient, making it suitable for performance-sensitive applications. -
How does CLSX improve code readability?
By simplifying the logic for applying class names, CLSX makes your code easier to read and maintain compared to complex string concatenation.
Irakli is a writer who loves computers and helping people solve their technical problems. He lives in Georgia and enjoys spending time with animals.
LinkedIn