How to Include Dynamic Image in React Native
- Understanding Dynamic Images in React Native
- Method 1: Using Local Images
- Method 2: Fetching Images from the Internet
- Method 3: Using Image Caching for Performance
- Conclusion
- FAQ
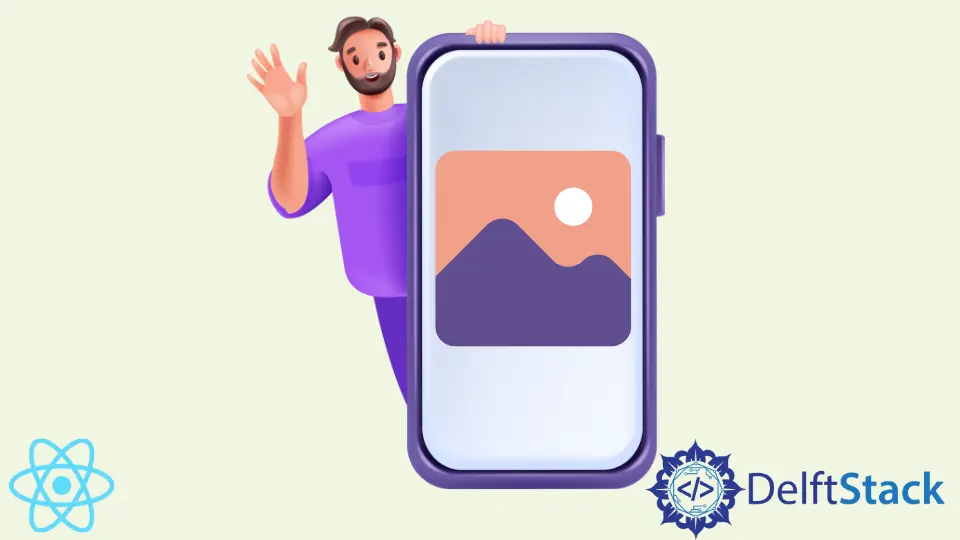
In the world of mobile app development, React Native stands out for its ability to create dynamic and engaging user experiences. One common requirement for many apps is to display images that can change based on user interactions or data fetched from APIs. Including dynamic images in React Native can elevate your application and make it more interactive.
In this tutorial, we will walk through the steps to include dynamic images in your React Native project. Whether you’re a beginner or an experienced developer, this guide will provide you with the insights you need to enhance your app’s visual appeal.
Understanding Dynamic Images in React Native
Dynamic images refer to images that are not static; they can change based on user input, data from APIs, or other conditions. In React Native, handling dynamic images is straightforward, thanks to its flexible components and state management. The key is to use state to manage the image source dynamically.
To get started, ensure you have a React Native environment set up. You can create a new project using the following command:
npx react-native init DynamicImageApp
This command will set up a new React Native project called DynamicImageApp
. Once your project is created, you can navigate to the project directory and start implementing dynamic images.
Method 1: Using Local Images
One of the simplest ways to include dynamic images in React Native is by using local images. This method is particularly useful when you have a set of images stored locally within your project.
Here’s how you can implement it:
- First, create a folder named
assets
in your project directory and place your images inside it. - Use the
Image
component from React Native and manage the image source using state.
Here’s a sample code snippet demonstrating how to achieve this:
import React, { useState } from 'react';
import { View, Image, Button } from 'react-native';
const App = () => {
const [imageSource, setImageSource] = useState(require('./assets/image1.jpg'));
const changeImage = () => {
setImageSource(require('./assets/image2.jpg'));
};
return (
<View>
<Image source={imageSource} style={{ width: 200, height: 200 }} />
<Button title="Change Image" onPress={changeImage} />
</View>
);
};
export default App;
In this code, we import necessary components and create a functional component called App
. We initialize the state with the first image using useState
. The changeImage
function updates the state to the second image when the button is pressed. The Image
component dynamically renders the current image based on the state.
This method is straightforward and ideal for scenarios where your images are not fetched from external sources. By managing the image source through state, you can easily switch between different local images.
Method 2: Fetching Images from the Internet
If your application requires displaying images from the web, you can dynamically fetch images using their URLs. This method is beneficial when you want to display user-uploaded images or images from a third-party API.
Here’s how to implement this:
- Use the
Image
component with a URL as the source. - Manage the URL through state to change the displayed image dynamically.
Here’s a sample code snippet to illustrate this:
import React, { useState } from 'react';
import { View, Image, Button } from 'react-native';
const App = () => {
const [imageURL, setImageURL] = useState('https://example.com/image1.jpg');
const changeImage = () => {
setImageURL('https://example.com/image2.jpg');
};
return (
<View>
<Image source={{ uri: imageURL }} style={{ width: 200, height: 200 }} />
<Button title="Change Image" onPress={changeImage} />
</View>
);
};
export default App;
In this example, we use a URL for the image source. The changeImage
function updates the URL to a different image when the button is clicked. The Image
component will automatically re-render with the new image source.
This method is particularly useful for applications that require real-time updates or user-generated content. By using URLs, you can easily integrate images from various sources, enhancing the versatility of your application.
Method 3: Using Image Caching for Performance
When working with dynamic images, especially those fetched from the web, performance can become an issue. To improve performance and reduce loading times, you can implement image caching. React Native provides a built-in way to handle caching using the Image
component.
Here’s how to implement image caching:
- Use a library like
react-native-fast-image
for advanced caching capabilities. - Manage the image source through state as before.
Here’s a sample code snippet:
import React, { useState } from 'react';
import { View, Button } from 'react-native';
import FastImage from 'react-native-fast-image';
const App = () => {
const [imageURL, setImageURL] = useState('https://example.com/image1.jpg');
const changeImage = () => {
setImageURL('https://example.com/image2.jpg');
};
return (
<View>
<FastImage
source={{
uri: imageURL,
priority: FastImage.priority.normal,
}}
style={{ width: 200, height: 200 }}
/>
<Button title="Change Image" onPress={changeImage} />
</View>
);
};
export default App;
In this code, we use the FastImage
component to load images. The source
prop includes the URI of the image and a priority level to optimize loading. When the button is pressed, the image changes, and thanks to caching, it loads faster.
Using a caching library can significantly enhance the user experience by reducing load times and providing a smoother transition between images.
Conclusion
Incorporating dynamic images into your React Native application can significantly enhance its interactivity and user experience. Whether you choose to use local images, fetch them from the internet, or implement caching for performance, understanding these methods will empower you to create more engaging applications. As you experiment with these techniques, you’ll find that dynamically managing images opens up a world of possibilities for your mobile app.
FAQ
-
How do I display dynamic images in React Native?
You can display dynamic images by managing the image source using state and theImage
component. -
Can I fetch images from an API in React Native?
Yes, you can fetch images from an API by using their URLs as the source for theImage
component. -
What is the advantage of using image caching?
Image caching improves performance by reducing load times and providing a smoother user experience. -
Can I use local images in my React Native app?
Yes, you can use local images by placing them in your project directory and referencing them with therequire
function. -
What libraries can help with image loading in React Native?
Libraries likereact-native-fast-image
can help with advanced image loading and caching capabilities.
Aminul Is an Expert Technical Writer and Full-Stack Developer. He has hands-on working experience on numerous Developer Platforms and SAAS startups. He is highly skilled in numerous Programming languages and Frameworks. He can write professional technical articles like Reviews, Programming, Documentation, SOP, User manual, Whitepaper, etc.
LinkedInRelated Article - React Native
- How to Align Text Vertically in React-Native
- How to Set Font Weight in React Native
- How to Align Text in React-Native
- How to Animate SVG in React Native
- How to Set the Border Color in React-Native
- React Native Foreach Loop