How to Center Image in React Native
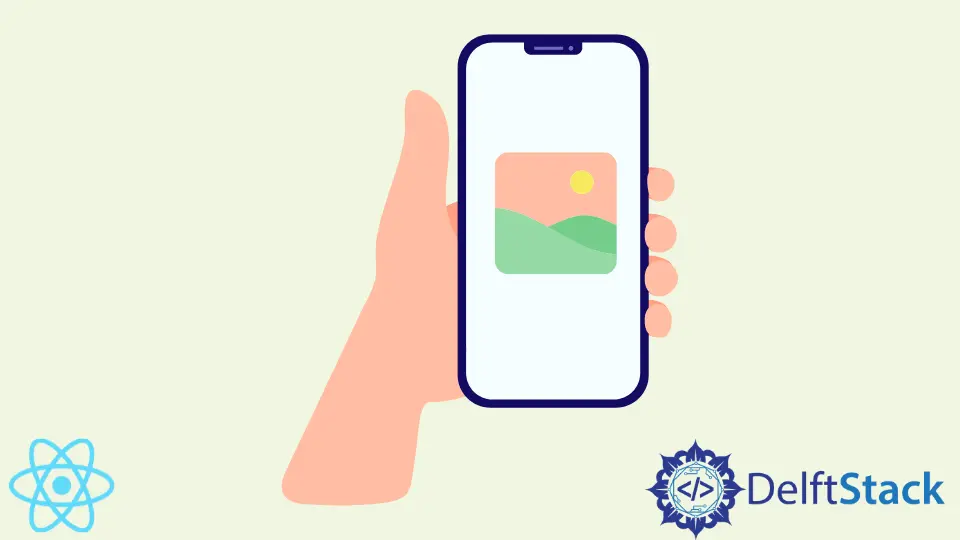
In React-Native, it is very easy to implement images in an app. After implementing, we need to provide the perfect alignment to our image to look better.
In this article, we will see how we can center an image item of a React-Native app. We will look at an example and explanation to make the topic easier.
Center Image in React Native
Sometimes we need to centralize the image item for various purposes. For example, if you are designing a preloader for an app, you need to provide a preloader image on the preloader window, which should be aligned in the center.
In our example below, we’ll illustrate how we can place an image item in the center of a React-Native app. The code snippet for our example will be as follows.
// importing necessary packages
import {StatusBar} from 'expo-status-bar';
import {Image, StyleSheet, Text, View} from 'react-native';
export default function App() {
return ( // Including the image
<View style={styles.container}>
<Text>Simple Image</Text>
<Image
style={styles.img}
source={{
uri: 'https://www.delftstack.com/assets/img/logo.png',
}
}
/>
<StatusBar style="auto" / > <
/View>
);
}
/ / Providing style to the app
const styles = StyleSheet.create({
container: {
flex: 1,
backgroundColor: '#fff',
alignItems: 'center',
justifyContent: 'center',
},
img: {
width: 200,
height: 200,
resizeMode: 'contain',
},
});
We already commanded the purpose of all necessary lines regarding the steps shared above in the example.
<Image style={styles.img} source={{ uri: 'https://www.delftstack.com/assets/img/logo.png', }} />
After including the image by the above code line, we set the below properties on the style sheet.
For the containers
:
Properties | Description |
---|---|
flex: 1, |
Define the container as flex. |
backgroundColor: '#fff', |
Set the background color two white. |
alignItems: 'center', |
Set horizontal align to center. |
justifyContent: 'center', |
Set vertical-align to center. |
For the image
:
Properties | Description |
---|---|
width: 200, |
Set the image width to 200. |
height: 200, |
Set the image height to 200. |
resizeMode:'contain', |
Don’t crop the image. |
You will get the output below on your screen when you run the app.
Output:
Note that the code shared above is created in React-Native. We used the Expo-CLI
to run the app, and also you need the latest version of Node.js.
If you don’t have Expo-CLI
in your environment, install it first.
Aminul Is an Expert Technical Writer and Full-Stack Developer. He has hands-on working experience on numerous Developer Platforms and SAAS startups. He is highly skilled in numerous Programming languages and Frameworks. He can write professional technical articles like Reviews, Programming, Documentation, SOP, User manual, Whitepaper, etc.
LinkedInRelated Article - React Native
- How to Align Text Vertically in React-Native
- How to Set Font Weight in React Native
- How to Align Text in React-Native
- How to Animate SVG in React Native
- How to Set the Border Color in React-Native
- React Native Foreach Loop