How to Download File in React Native
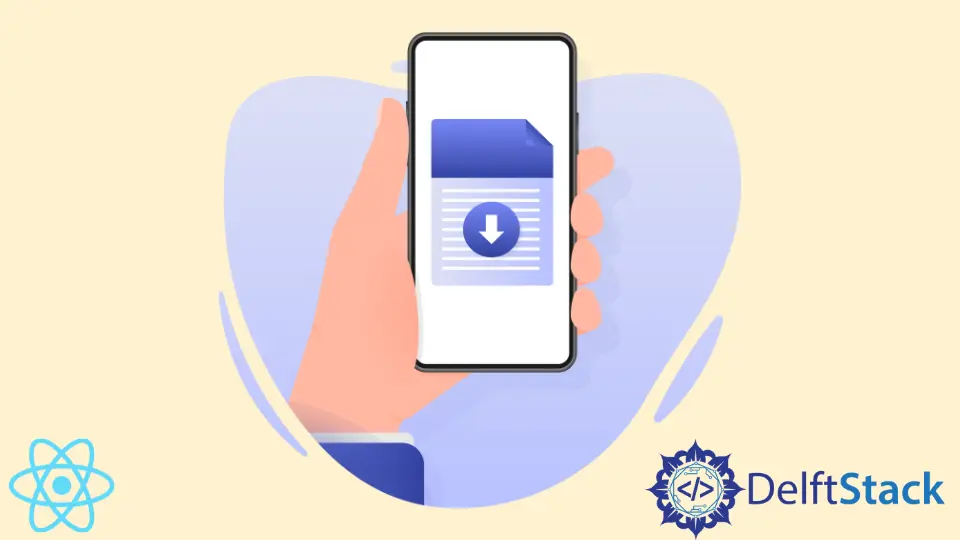
This article will show how we can download a file using a React-Native app. Also, we will make the topic easier by using the necessary examples and explanations.
Download File in React Native
If you are working with the Expo
, you must follow the steps below.
-
Install the
expo-file-system
by the commandnpm i expo-file-system
. -
Install the
expo-linking
by the commandnpm i expo-linking
.
Let’s have an example of how to download a file from a URL using the React-Native app.
Our example below demonstrates how we can download a pdf file using our React-Native app. The code snippet for our example will be as follows.
// importing necessary packages
import * as FileSystem from 'expo-file-system';
import * as Linking from 'expo-linking';
import * as React from 'react';
import {Alert, StyleSheet, Text, View} from 'react-native';
export default class App extends React.Component {
constructor(props) {
super(props);
}
componentDidMount() {
let URL = 'http://www.soundczech.cz/temp/lorem-ipsum.pdf';
let LocalPath = FileSystem.documentDirectory + 'lorem-ipsum.pdf';
FileSystem.downloadAsync(URL, LocalPath)
.then(({uri}) => Linking.openURL(uri));
}
render() {
return (<View style = {styles.container}>
<Text style = {styles.paragraph}>Downloading your
file...</Text>
</View>);
}
}
// Providing some styles to the UI components
const styles = StyleSheet.create({
container: {
flex: 1,
justifyContent: 'center',
paddingTop: 30,
padding: 8,
},
paragraph: {
margin: 24,
fontSize: 20,
textAlign: 'center',
},
});
We already commanded the purpose of all necessary lines regarding the steps shared above in the example. Now we will describe some important parts of the example code shared above.
The line let URL = 'http://www.soundczech.cz/temp/lorem-ipsum.pdf';
provides the file URL and the line let LocalPath = FileSystem.documentDirectory + "lorem-ipsum.pdf";
provides the file location on Local storage. The block of code below downloads the file and opens it.
FileSystem
.downloadAsync(URL, LocalPath) // A function to download and open the file
.then(({uri}) => Linking.openURL(uri)); // Linking the URL
}
When you run the app, you’ll get the below output.
Output:
Note that the code shared above is created in React-Native, and we used the Expo-CLI
to run the app. Also, you need the latest version of Node.js.
If you don’t have Expo-CLI
in your environment, install it first.
Aminul Is an Expert Technical Writer and Full-Stack Developer. He has hands-on working experience on numerous Developer Platforms and SAAS startups. He is highly skilled in numerous Programming languages and Frameworks. He can write professional technical articles like Reviews, Programming, Documentation, SOP, User manual, Whitepaper, etc.
LinkedIn