How to Align Elements in React Native
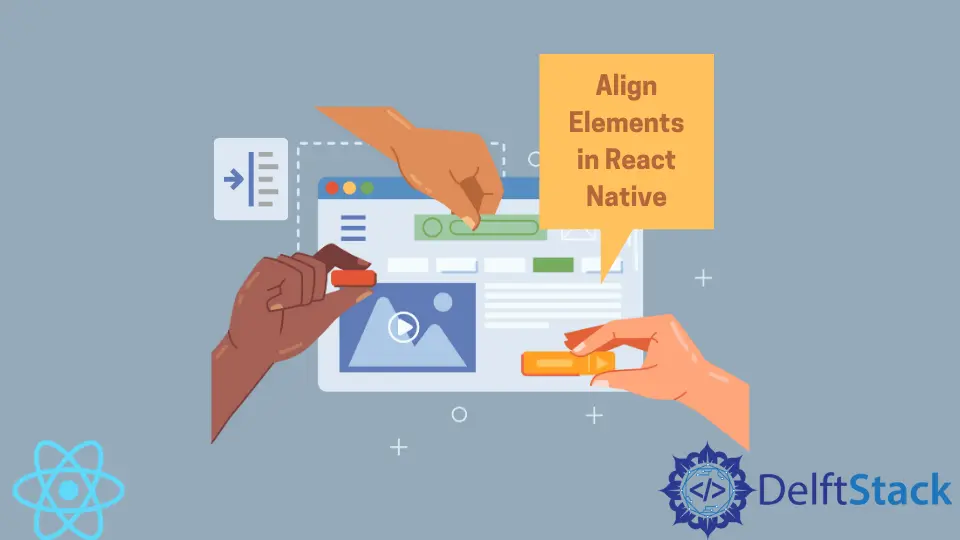
In this article, we will see how we can align React-Native elements and make them easier to understand by using necessary examples and explanations.
Align Elements in React Native
Two kinds of alignment are available for aligning items: Horizontal and Vertical. There are three properties for Horizontal alignment: Top, Center, and button, and there are three properties for Vertical alignment: Left, Center, and Right.
Using the flex
, we can easily align items using the syntax below.
Syntax:
container: {flex: 1
justifyContent: 'center', // For aligning vertically
alignItems: 'center', // For aligning horizontally
}
Use flex
to Align the React-Native Elements
// Importing necessary packages
import {StatusBar} from 'expo-status-bar';
import {Image, StyleSheet, Text, View, ViewComponent, ViewPagerAndroidBase} from 'react-native';
// Our main function
export default function App() {
let img = './assets/image1.jpg'; // Declaring a variable that hold the image
// location
return (
<View style = {styles.container}><Text>This is a body aligned center<
/Text>
<View style={styles.container01}>
<Text>I am Left</Text>
<Image style = {styles.img} source =
{
require(img)
} />
<StatusBar style="auto" />
</View>
<View style={styles.container02}>
<Text>I am Center</Text>
<Image style = {styles.img} source =
{
require(img)
} />
<StatusBar style="auto" />
</View>
<View style={styles.container03}>
<Text>I am Right</Text>
<Image style = {styles.img} source = {
require(img)
} />
<StatusBar style="auto" /></View>
</View>);
}
const styles = StyleSheet.create({
// Providing some styles to the UI
container: {
flex: 1,
backgroundColor: '#fff',
alignItems: 'center',
justifyContent: 'center',
margin: 50,
},
container01: {
flex: 1,
alignSelf: 'flex-start',
},
container02: {
flex: 1,
alignSelf: 'center',
},
container03: {
flex: 1,
alignSelf: 'flex-end',
},
img: {
width: 100,
height: 100,
},
});
We already commanded the purpose of all necessary lines regarding the steps shared above in the example. In the example, the container01
, container02
, and container03
is the child element of container
.
When you run the app, you’ll get the below output on your screen.
Output:
Note that the code shared above is created in React-Native
, and we used Expo-CLI
to run the app.
Aminul Is an Expert Technical Writer and Full-Stack Developer. He has hands-on working experience on numerous Developer Platforms and SAAS startups. He is highly skilled in numerous Programming languages and Frameworks. He can write professional technical articles like Reviews, Programming, Documentation, SOP, User manual, Whitepaper, etc.
LinkedInRelated Article - React Native
- How to Align Text Vertically in React-Native
- How to Set Font Weight in React Native
- How to Align Text in React-Native
- How to Animate SVG in React Native
- How to Set the Border Color in React-Native
- React Native Foreach Loop