Axios GET Header in React Native
- What is Axios?
- Installing Axios in React Native
- Sending a GET Request with Custom Headers
- Handling Errors in Axios GET Requests
- Conclusion
- FAQ
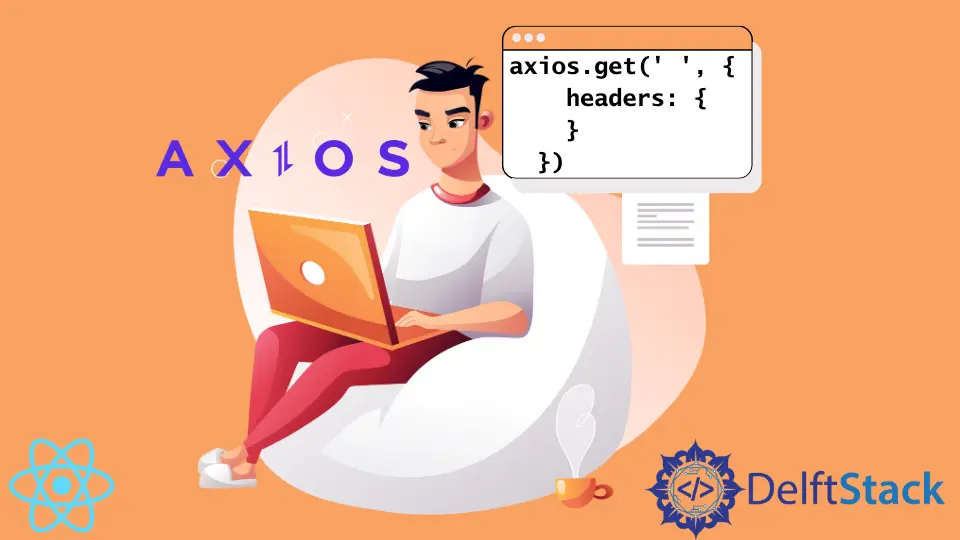
In the world of mobile app development, React Native has emerged as a powerful framework for building cross-platform applications. One of the most common tasks developers face is fetching data from APIs. Axios, a popular promise-based HTTP client, simplifies this process significantly.
In this tutorial, we will explore how to use Axios to send GET requests in React Native, specifically focusing on how to include headers in those requests. Whether you’re looking to authenticate your requests or pass custom data, understanding how to manipulate headers can elevate your app’s functionality. Let’s dive into the details!
What is Axios?
Axios is an HTTP client for JavaScript that allows you to make requests to servers. Its promise-based nature makes it easy to work with asynchronous code, which is essential in a mobile environment. By default, Axios sends requests with a set of standard headers, but you may need to customize these headers based on your API’s requirements. This customization is particularly important when dealing with authentication tokens, content types, or any other specific data your API expects.
Installing Axios in React Native
Before we start sending GET requests with headers, we need to install Axios in our React Native project. You can do this using npm or yarn. Open your terminal and navigate to your project directory, then run:
npm install axios
or
yarn add axios
Once installed, you can import Axios into your components and start using it to make requests. This simple step sets the stage for all the powerful functionalities that Axios brings to the table.
Sending a GET Request with Custom Headers
Now that we have Axios ready to go, let’s see how we can send a GET request with custom headers. In this example, we will use Axios to fetch data from a public API that requires an authorization token in the headers.
import React, { useEffect } from 'react';
import axios from 'axios';
const App = () => {
useEffect(() => {
const fetchData = async () => {
try {
const response = await axios.get('https://api.example.com/data', {
headers: {
Authorization: 'Bearer your_token_here'
}
});
console.log(response.data);
} catch (error) {
console.error('Error fetching data:', error);
}
};
fetchData();
}, []);
return null;
};
export default App;
In this code snippet, we define a functional component called App
. Inside the useEffect
hook, we make an asynchronous GET request to a hypothetical API endpoint. The headers object includes an Authorization
field with a bearer token. This token is crucial for authenticating the request. If the request is successful, the response data is logged to the console. If there’s an error, it is caught and logged as well.
Output:
{ data: {...} }
By including custom headers, you can tailor your requests to meet the specific needs of the API you are working with. This is particularly useful in scenarios where APIs require tokens or other forms of authentication.
Handling Errors in Axios GET Requests
Error handling is a vital aspect of any API interaction. Axios provides a straightforward way to manage errors that may arise during the request process. In the previous example, we used a try-catch block to handle potential errors. Let’s expand on that to provide a more user-friendly experience.
import React, { useEffect, useState } from 'react';
import axios from 'axios';
import { View, Text } from 'react-native';
const App = () => {
const [data, setData] = useState(null);
const [error, setError] = useState(null);
useEffect(() => {
const fetchData = async () => {
try {
const response = await axios.get('https://api.example.com/data', {
headers: {
Authorization: 'Bearer your_token_here'
}
});
setData(response.data);
} catch (err) {
setError(err.message);
}
};
fetchData();
}, []);
return (
<View>
{error ? (
<Text>Error: {error}</Text>
) : (
<Text>Data: {JSON.stringify(data)}</Text>
)}
</View>
);
};
export default App;
In this enhanced example, we introduce state variables to manage both the fetched data and any errors that occur. If an error happens, we update the error
state, which is then displayed to the user. This not only helps in debugging but also enhances the user experience by providing feedback on what went wrong.
Output:
Error: [error message]
This approach ensures that your application remains robust and user-friendly, even when external API calls fail.
Conclusion
Axios is an invaluable tool for React Native developers looking to streamline their API interactions. By understanding how to send GET requests with custom headers, you can effectively manage authentication and tailor your requests to meet specific API requirements. Moreover, implementing error handling ensures that your users receive clear feedback when something goes wrong. As you continue to build your application, mastering Axios will undoubtedly enhance your development experience and the overall performance of your app.
FAQ
-
How do I install Axios in my React Native project?
You can install Axios by runningnpm install axios
oryarn add axios
in your project directory. -
Can I send multiple headers with Axios?
Yes, you can include multiple headers in the headers object when making a request. -
What should I do if my GET request fails?
Implement error handling using try-catch blocks to manage any errors that arise during the request. -
Is Axios compatible with other JavaScript frameworks?
Yes, Axios can be used with any JavaScript framework, including Angular, Vue, and Node.js. -
How can I test my API requests in React Native?
You can use tools like Postman to test your API endpoints before implementing them in your React Native application.
Aminul Is an Expert Technical Writer and Full-Stack Developer. He has hands-on working experience on numerous Developer Platforms and SAAS startups. He is highly skilled in numerous Programming languages and Frameworks. He can write professional technical articles like Reviews, Programming, Documentation, SOP, User manual, Whitepaper, etc.
LinkedIn