How to Remove Rows With NA in One Column in R
-
Remove Rows With
NA
in One Column Usingis.na()
in R -
Remove Rows With
NA
in One Column Usingcomplete.cases()
in R -
Remove Rows With
NA
in One Column Usingdrop_na()
in R -
Remove Rows With
NA
in One Column Usingna.omit()
in R -
Remove Rows With
NA
in One Column Usingsubset()
in R -
Remove Rows With
NA
in One Column Usingfilter()
in R - Conclusion
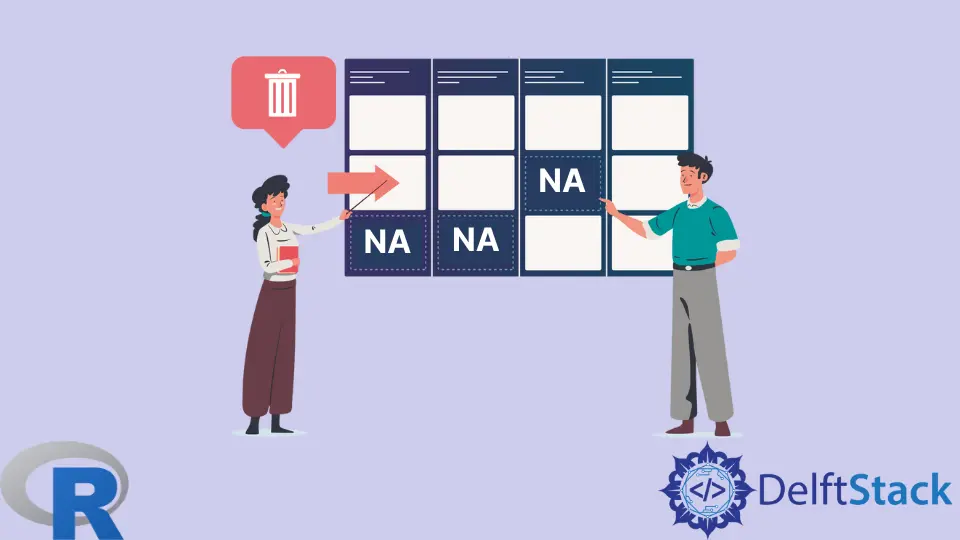
Handling missing values is a common challenge in data analysis, and one frequent task is removing rows with NA
values in a specific column. In R, there are several methods to achieve this, ranging from base R functions to specialized packages like tidyr
and dplyr
.
In this article, we’ll explore various approaches to removing rows with NA
values and provide detailed examples to guide you through the process.
Remove Rows With NA
in One Column Using is.na()
in R
One approach to deal with missing values involves using the is.na()
function.
The is.na()
function in R is designed to detect missing values (NA
) within a data frame. It returns a logical vector of the same length as the input vector, with TRUE
values indicating the presence of NA
.
Leveraging this logical vector, we can efficiently filter and remove the corresponding rows from our data frame.
The basic syntax for using is.na()
to remove rows with NA
values in a specific column is as follows:
# Assuming your data frame is 'df' and the column is 'column_name'
df <- df[!is.na(df$column_name),
]
Breaking down the syntax:
df
: Replace this with the name of your data frame.column_name
: Specify the column where you want to remove rows withNA
values.
Let’s illustrate the process with a practical example using a data frame named Delftstack
. This data frame contains columns for Name
, LastName
, Id
, and Designation
, and we aim to remove rows with NA
values in the Id
column.
Delftstack <- data.frame(
Name = c("Jack", "John", "Mike", "Michelle", "Jhonny"),
LastName = c("Danials", "Cena", "Chandler", "McCool", "Nitro"),
Id = c(101, 102, NA, 104, NA),
Designation = c("CEO", "Project Manager", "Senior Dev", "Junior Dev", "Intern")
)
print("The dataframe before removing the rows:-")
print(Delftstack)
Delftstack <- Delftstack[!is.na(Delftstack$Id),
]
print("The dataframe after removing the rows:-")
print(Delftstack)
In this example, we first create the data frame Delftstack
with four columns. The goal is to remove rows with missing values, specifically in the Id
column.
Before any modifications, we print the original data frame to visually inspect its structure. Following that, the is.na()
method is applied to the Id
column to filter out rows containing NA
values.
The resulting data frame, now free of the targeted rows with missing data, is stored back in the variable Delftstack
.
A subsequent print
statement displays the modified data frame, illustrating the removal of rows with NA
values in the Id
column. This example effectively demonstrates how the is.na()
function can be seamlessly integrated into the R workflow to handle missing values in a specific column within a data frame.
Code Output:
Remove Rows With NA
in One Column Using complete.cases()
in R
When dealing with missing values in R, the complete.cases()
method provides another effective approach to remove rows with NA
values in a specific column.
The complete.cases()
function in R is designed to identify complete cases within a data frame, effectively filtering out rows containing any NA
values. When applied to a specific column, it allows us to focus on eliminating rows where the designated column has missing data.
The syntax for using complete.cases()
to remove rows with NA
values in a specific column is as follows:
# Assuming your data frame is 'df' and the column is 'column_name'
df <- df[complete.cases(df$column_name),
]
Where:
df
: Replace this with the name of your data frame.column_name
: Specify the column where you want to remove rows withNA
values.
Let’s illustrate the process with a practical example using the same data frame named Delftstack
. This time, we aim to remove rows with NA
values in the Id
column using the complete.cases()
method.
Delftstack <- data.frame(
Name = c("Jack", "John", "Mike", "Michelle", "Jhonny"),
LastName = c("Danials", "Cena", "Chandler", "McCool", "Nitro"),
Id = c(101, 102, NA, 104, NA),
Designation = c("CEO", "Project Manager", "Senior Dev", "Junior Dev", "Intern")
)
print("The dataframe before removing the rows:-")
print(Delftstack)
Delftstack <- Delftstack[complete.cases(Delftstack$Id),
]
print("The dataframe after removing the rows:-")
print(Delftstack)
In this example, we begin by creating the same data frame, Delftstack
. The goal is to remove rows with missing values, specifically in the Id
column, using the complete.cases()
method.
Before any modifications, we print the original data frame to visualize its structure. The complete.cases()
method is then applied to the Id
column, effectively filtering out rows containing NA
values. The resulting data frame, now without the targeted rows with missing data, is stored back in the variable Delftstack
.
A subsequent print
statement showcases the modified data frame, highlighting the removal of rows with NA
values in the Id
column. This example demonstrates how the complete.cases()
method provides an alternative and equally effective solution for handling missing values in a specific column within a data frame.
Code Output:
In this output, we can observe the successful removal of rows with NA
values in the specified column using the complete.cases()
method, resulting in a clean and refined data frame.
Remove Rows With NA
in One Column Using drop_na()
in R
In R, the drop_na()
function from the tidyr
package provides a convenient method to remove rows with NA
values in a specific column.
The drop_na()
function is part of the tidyr
package in R and is designed to drop rows containing NA
values. When applied to a specific column, it allows us to focus on removing rows where the designated column has missing data.
The basic syntax is as follows:
# Assuming your data frame is 'df' and the column is 'column_name'
library(tidyr)
df <- df %>%
drop_na(column_name)
Where:
df
: Replace this with the name of your data frame.column_name
: Specify the column where you want to remove rows withNA
values.
Before using the drop_na()
function, you need to ensure that the tidyr
package is installed and loaded. If you haven’t installed it yet, you can do so using the following command:
install.packages("tidyr")
Let’s illustrate the process with a practical example using a data frame named Delftstack
. In this case, we want to remove rows with NA
values in the Id
column using the drop_na()
method.
Delftstack <- data.frame(
Name = c("Alice", "Bob", "Charlie", "David", "Eva"),
LastName = c("Anderson", "Brown", "Clark", "Davis", "Evans"),
Id = c(201, NA, 203, NA, 205),
Designation = c("Manager", "Developer", "Analyst", "Intern", "CEO")
)
print("The dataframe before removing the rows:-")
print(Delftstack)
library(tidyr)
Delftstack <- Delftstack %>%
drop_na(Id)
print("The dataframe after removing the rows:-")
print(Delftstack)
Similar to the previous examples, we start by creating the same data frame, Delftstack
. The objective is to remove rows with missing values, specifically in the Id
column, using the drop_na()
method from the tidyr
package.
Before any modifications, we print the original data frame to visualize its structure. The drop_na()
method is then applied to the Id
column, effectively removing rows containing NA
values.
The resulting data frame, now without the targeted rows with missing data, is stored back in the variable Delftstack
.
A subsequent print
statement showcases the modified data frame, emphasizing the removal of rows with NA
values in the specified column. This example demonstrates how the drop_na()
function, as part of the tidyr
package, offers a concise and powerful solution for handling missing values in a specific column within a data frame.
Code Output:
In the output, we can observe the successful removal of rows with NA
values using the drop_na()
method, resulting in a refined data frame without missing values in the specified column.
Remove Rows With NA
in One Column Using na.omit()
in R
In R, the na.omit()
function is another valuable tool for removing rows with NA
values, and it provides a straightforward way to handle missing data in a specific column.
The na.omit()
function in R is designed to omit missing values (NA
) from a data frame. When applied to a data frame, it removes any row containing at least one NA
value.
To use it for a specific column, you can subset the data frame based on the absence of NA
values in that particular column:
# Assuming your data frame is 'df' and the column is 'column_name'
df <- na.omit(
df[!is.na(df$column_name),
]
)
Breaking down the above code:
df
: Replace this with the name of your data frame.column_name
: Specify the column where you want to remove rows withNA
values.
Let’s illustrate the process with a practical example using a data frame named Delftstack
. In this case, we want to remove rows with NA
values in the Id
column using the na.omit()
method.
Delftstack <- data.frame(
Name = c("Grace", "Henry", "Ivy", "Jack", "Kate"),
LastName = c("Graham", "Harrison", "Irwin", "Johnson", "Keller"),
Id = c(301, 302, NA, 304, NA),
Designation = c("Analyst", "Manager", "Developer", "CEO", "Intern")
)
print("The dataframe before removing the rows:-")
print(Delftstack)
Delftstack <- na.omit(
Delftstack[!is.na(Delftstack$Id),
]
)
print("The dataframe after removing the rows:-")
print(Delftstack)
In this example, we create the data frame Delftstack
. Then, we print the original data frame to visualize its structure.
The na.omit()
method is then applied to the Id
column, effectively removing rows containing NA
values. The resulting data frame, now without the targeted rows with missing data, is stored back in the variable Delftstack
.
A subsequent print
statement showcases the modified data frame, emphasizing the removal of rows with NA
values. This example demonstrates how the na.omit()
function provides a concise and effective solution for handling missing values in a specific column within a data frame.
Code Output:
In the output, we observe the successful removal of rows with NA
values using the na.omit()
method. The resulting data frame is clean and does not contain any missing values in the specified column.
Remove Rows With NA
in One Column Using subset()
in R
In R, the subset()
function offers a flexible way to remove rows with NA
values from a specific column within a data frame.
The subset()
function in R allows us to filter rows based on specified conditions. To remove rows with NA
values in a particular column, we can use the following syntax:
# Assuming your data frame is 'df' and the column is 'column_name'
df <- subset(df, !is.na(column_name))
Breaking down the syntax:
df
: Replace this with the name of your data frame.column_name
: Specify the column where you want to remove rows withNA
values.
Let’s illustrate the process with a practical example using the same data frame named Delftstack
. In this scenario, we aim to remove rows with NA
values in the Id
column using the subset()
method.
Delftstack <- data.frame(
Name = c("Oliver", "Penelope", "Quincy", "Riley", "Sophia"),
LastName = c("Owens", "Parker", "Quinn", "Reed", "Smith"),
Id = c(401, NA, 403, NA, 405),
Designation = c("Developer", "Manager", "Analyst", "CEO", "Intern")
)
print("The dataframe before removing the rows:-")
print(Delftstack)
Delftstack <- subset(Delftstack, !is.na(Id))
print("The dataframe after removing the rows:-")
print(Delftstack)
In this example, we start by creating the same data frame, Delftstack
, and printing the original data frame to visualize its structure.
The subset()
method is then applied to the Id
column, effectively removing rows containing NA
values. The resulting data frame, now without the targeted rows with missing data, is stored back in the variable Delftstack
.
A subsequent print
statement showcases the modified data frame, emphasizing the removal of rows with NA
values. This example illustrates how the subset()
function provides a straightforward and versatile solution for handling missing values in a specific column within a data frame.
Code Output:
In the output, we can observe the successful removal of rows with NA
values in the Id
column using the subset()
method. The resulting data frame is clean and does not contain any missing values in the specified column.
Remove Rows With NA
in One Column Using filter()
in R
In R, the filter()
function, part of the dplyr
package, provides a powerful and expressive way to remove rows with NA
values from a specific column within a data frame.
The filter()
function allows us to conditionally select rows based on specified criteria. To remove rows with NA
values in a particular column, we can use the following syntax:
# Assuming your data frame is 'df' and the column is 'column_name'
library(dplyr)
df <- df %>%
filter(!is.na(column_name))
Where:
df
: Replace this with the name of your data frame.column_name
: Specify the column where you want to remove rows withNA
values.
Before using the filter()
function, ensure that the dplyr
package is installed. If you haven’t installed it yet, you can do so with the following command:
install.packages("dplyr")
Now, let’s see the process with a practical example using the same data frame named Delftstack
. In this scenario, our goal is to remove rows with NA
values in the Id
column using the filter()
method.
Delftstack <- data.frame(
Name = c("Taylor", "Ursula", "Victor", "Wendy", "Xander"),
LastName = c("Thomas", "Underwood", "Vance", "Williams", "Xu"),
Id = c(501, NA, 503, NA, 505),
Designation = c("Analyst", "Manager", "Developer", "CEO", "Intern")
)
print("The dataframe before removing the rows:-")
print(Delftstack)
library(dplyr)
Delftstack <- Delftstack %>%
filter(!is.na(Id))
print("The dataframe after removing the rows:-")
print(Delftstack)
In this example, we first print the original data frame Delftstack
to visualize its structure. The filter()
method is then applied to the Id
column, effectively removing rows containing NA
values.
The resulting data frame, now without the targeted rows with missing data, is stored back in the variable Delftstack
.
A subsequent print
statement showcases the modified data frame, emphasizing the removal of rows with NA
values. This example demonstrates how the filter()
function, as part of the dplyr
package, provides a concise and expressive solution for handling missing values in a specific column within a data frame.
Code Output:
In the output, we can observe the successful removal of rows with NA
values in the column using the filter()
method. The resulting data frame is clean and does not contain any missing values in the specified column.
Conclusion
This article explored various methods for removing rows with NA
values from a specific column in R, offering flexibility to cater to different preferences and workflows.
We discussed the application of is.na()
, complete.cases()
, drop_na()
from the tidyr
package, na.omit()
, subset()
, and filter()
from the dplyr
package. Each method showcased its unique syntax and functionality, providing users with options based on their coding style and preferences.
Whether using base R functions or leveraging packages like tidyr
and dplyr
, the presented examples demonstrated effective ways to handle missing values and ensure clean and reliable datasets for further analysis. Choose the method that aligns best with your coding practices and data manipulation needs.
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook