How to Delete Multiple Columns in R
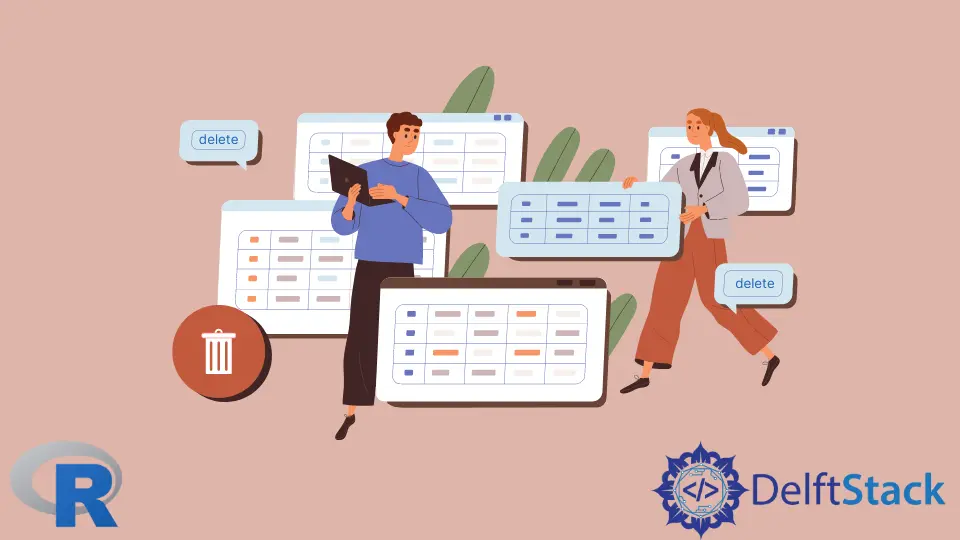
Multiple columns can be simultaneously deleted from a data frame in R.
This tutorial demonstrates how to delete multiple columns in R.
Delete Multiple Columns in R
There are two methods to delete multiple columns from a data frame in R. These methods are demonstrated below.
Delete Multiple Columns Using Base R
We can delete multiple columns from the data frame in R by assigning Null
values to columns. The syntax to delete multiple columns in R using Base R is:
DataFrame[ , c('column1', 'column2',………..,'column_n)] <- list(NULL)
Where DataFrame
is the given data frame and in the list
, we make the columns Null
. Let’s try an example:
#create a data frame
Delftstack <- data.frame(Name=c('Jack', 'John', 'Mike', 'Michelle', 'Jhonny'),
LastName=c('Danials', 'Cena', 'Chandler', 'McCool', 'Nitro'),
Id=c(101, 102, 103, 104, 105),
Designation=c('CEO', 'Project Manager', 'Senior Dev', 'Junior Dev', 'Intern'))
#View the data frame before deleting the columns
print('The DataFrame Before Deletion:')
Delftstack
#delete columns Name and LastName from a data frame
Delftstack[ , c('Name', 'LastName')] <- list(NULL)
#view data frame after deleting the columns
print('The DataFrame After Deletion:')
Delftstack
The code above will delete the columns given as the arguments. See output:
[1] "The DataFrame Before Deletion:"
Name LastName Id Designation
1 Jack Danials 101 CEO
2 John Cena 102 Project Manager
3 Mike Chandler 103 Senior Dev
4 Michelle McCool 104 Junior Dev
5 Jhonny Nitro 105 Intern
[1] "The DataFrame After Deletion:"
Id Designation
1 101 CEO
2 102 Project Manager
3 103 Senior Dev
4 104 Junior Dev
5 105 Intern
We can also use a range of columns to be deleted with this method, the range can be shown with the :
operator, and we can pass it as a parameter instead of column names. See example:
#create a data frame
Delftstack <- data.frame(Name=c('Jack', 'John', 'Mike', 'Michelle', 'Jhonny'),
LastName=c('Danials', 'Cena', 'Chandler', 'McCool', 'Nitro'),
Id=c(101, 102, 103, 104, 105),
Designation=c('CEO', 'Project Manager', 'Senior Dev', 'Junior Dev', 'Intern'))
#View the data frame before deleting the columns
print('The DataFrame Before Deletion:')
Delftstack
#delete columns Name and LastName from data frame
Delftstack[, 1:2] <- list(NULL)
#view data frame after deleting the columns
print('The DataFrame After Deletion:')
Delftstack
The code above will have a similar output as the example above. See the result after deletion:
[1] "The DataFrame After Deletion:"
Id Designation
1 101 CEO
2 102 Project Manager
3 103 Senior Dev
4 104 Junior Dev
5 105 Intern
Delete Multiple Columns Using the dplyr
Package in R
We can also use the dplyr
package to delete multiple columns from a data frame. We can extract the columns using the select()
method.
We can also use the one_of
method to create a new data frame with the deleted columns from the given data frame.
The syntax for this method is:
dataframe_new <- data frame %>% select(- one_of(columns to be removed))
First, install and load the dplyr
package, and then we can use the above method to delete multiple columns from a data frame. See example:
install.packages("dplyr")
library("dplyr")
#create a data frame
Delftstack <- data.frame(Name=c('Jack', 'John', 'Mike', 'Michelle', 'Jhonny'),
LastName=c('Danials', 'Cena', 'Chandler', 'McCool', 'Nitro'),
Id=c(101, 102, 103, 104, 105),
Designation=c('CEO', 'Project Manager', 'Senior Dev', 'Junior Dev', 'Intern'))
#View the data frame before deleting the columns
print('The DataFrame Before Deletion:')
Delftstack
# Columns to be removed
RemoveColumns <- c("Name", "LastName")
#delete columns Name and LastName from a data frame
DelftstackNew <- Delftstack %>% select(- one_of(RemoveColumns))
#view data frame after deleting the columns
print('The DataFrame After Deletion:')
DelftstackNew
The code above will create a new data frame from the previous one with the deleted columns. See output:
[1] "The DataFrame Before Deletion:"
Name LastName Id Designation
1 Jack Danials 101 CEO
2 John Cena 102 Project Manager
3 Mike Chandler 103 Senior Dev
4 Michelle McCool 104 Junior Dev
5 Jhonny Nitro 105 Intern
[1] "The DataFrame After Deletion:"
Id Designation
1 101 CEO
2 102 Project Manager
3 103 Senior Dev
4 104 Junior Dev
5 105 Intern
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook