How to Create Empty Data Frame in R
-
Create an Empty Data Frame in R Using the
data.frame()
Function - Create an Empty Data Frame in R by Creating a Matrix and Converting to a Data Frame
- Create an Empty Data Frame in R by Initializing a Set of Vectors
-
Create an Empty Data Frame in R Using the
structure()
Function -
Create an Empty Data Frame in R Using the
tibble()
Function From thetibble
Package - Conclusion
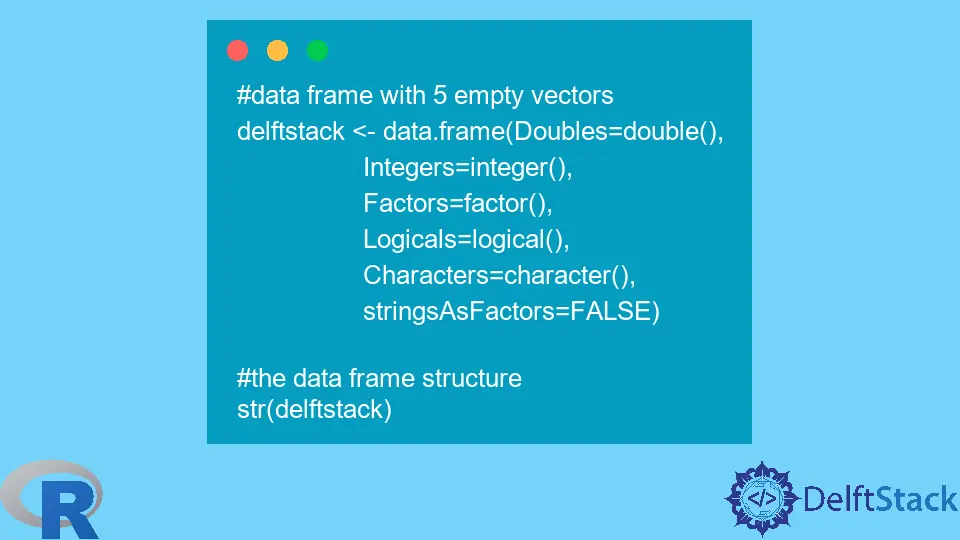
In R, a data frame is a fundamental data structure used for storing and manipulating tabular data.
It is a two-dimensional object with rows and columns, similar to a spreadsheet or a database table. Sometimes, you may need to create an empty data frame as a starting point for your data analysis or to populate it later with data.
In this article, we will explore how to create an empty data frame in R.
Create an Empty Data Frame in R Using the data.frame()
Function
One common method to create an empty data frame in R is by using the data.frame()
function.
The data.frame()
function in R is a versatile tool for creating and manipulating data frames. It takes arguments that define the structure of the data frame, including the column names and initial values.
To create an empty data frame, we can use this function with the appropriate parameters. The basic syntax of the data.frame()
function for creating an empty data frame is as follows:
empty_df <- data.frame()
This simple call initializes an empty data frame, setting the lengths of both columns and rows to zero. If you wish to include specific columns, you can provide them as arguments within the function call.
Example 1: Creating an Empty Data Frame Using data.frame()
Now, let’s explore a complete working example of creating an empty data frame using the data.frame()
function:
empty_df <- data.frame()
print("Empty Data Frame:")
print(empty_df)
In the provided code, we start by invoking the data.frame()
function without any arguments. This results in the creation of an empty data frame stored in the variable empty_df
.
The subsequent print()
statements are used to display a clear message and the content of the empty data frame.
Code Output:
In this output, you can see the confirmation message and the representation of the empty data frame with zero columns and zero rows.
This demonstrates a basic yet essential technique for initializing an empty data frame in R using the data.frame()
function.
Example 2: Creating an Empty Data Frame With Specified Column Names
Now, let’s create an empty data frame with two columns:
# Create an empty data frame with specified column names
empty_df <- data.frame(ID = integer(), Name = character())
print("Empty Data Frame:")
print(empty_df)
Here, we create an empty data frame named empty_df
with two columns - ID
of integer type and Name
of character type. The integer()
and character()
functions are used to define empty vectors for each column, indicating their respective data types.
Code Output:
The resulting empty_df
data frame is effectively empty, ready for further data input or manipulation. The flexibility of the data.frame()
function allows for the concise and customizable creation of empty data frames in R.
Create an Empty Data Frame in R by Creating a Matrix and Converting to a Data Frame
Another approach to generating an empty data frame in R involves creating an empty matrix and subsequently converting it into a data frame. This method allows for more flexibility as you can easily add columns or customize the matrix structure before conversion.
To create an empty data frame using this method, we first initiate an empty matrix with zero columns and rows. We then use the data.frame()
function to convert the empty matrix into a data frame.
empty_matrix <- matrix(ncol = 0, nrow = 0)
empty_df <- data.frame(empty_matrix)
Here, the matrix(ncol = 0, nrow = 0)
statement initializes an empty matrix with zero columns and rows. The data.frame(empty_matrix)
then converts this matrix into an empty data frame.
This process allows for the creation of an empty data frame with the option to define specific columns in the matrix before conversion.
Example 1: Creating an Empty Data Frame by Creating a Matrix
Now, let’s see a working example of creating an empty data frame by creating an empty matrix and converting it:
# Create an empty matrix
demo_matrix <- matrix(ncol = 0, nrow = 0)
# Convert the matrix to a data frame
delftstack <- data.frame(demo_matrix)
# Display the data frame
print("Empty Data Frame:")
print(delftstack)
In this example, we begin by initializing an empty matrix named demo_matrix
with zero columns and zero rows. Subsequently, we use the data.frame()
function to convert this empty matrix into a data frame stored in the variable delftstack
.
The print()
statements are employed to provide informative messages and display the content of the resulting data frame.
Code Output:
The output illustrates the success of the process, confirming the creation of an empty data frame through the generation of an empty matrix and subsequent conversion using the data.frame()
function in R.
Example 2: Creating an Empty Data Frame Without Specifying Column Names
Additionally, an empty data frame can be created without specifying column names by passing empty vectors as arguments:
# Declare an empty data frame with 4 columns and null entries
delftstack <- data.frame(
matrix(vector(), 0, 4, dimnames = list(c(), c("C1", "C2", "C3", "C4"))),
stringsAsFactors = FALSE
)
# Display the empty data frame
print("Empty Data Frame:")
print(delftstack)
In this code, an empty data frame named delftstack
is created with four columns. The matrix(vector(), 0, 4, dimnames = list(c(), c("C1", "C2", "C3", "C4")))
statement initializes an empty matrix with four columns and null entries.
The data.frame()
function is then used to convert this matrix to a data frame, and the resulting structure is displayed.
Code Output:
This output confirms the successful creation of an empty data frame with four columns using the method of generating an empty matrix and converting it. The column names are also specified in this example.
Create an Empty Data Frame in R by Initializing a Set of Vectors
An alternative method for crafting an empty data frame in R involves defining the data frame structure by initializing a set of empty vectors. This approach allows explicit specification of the column types and serves as a useful foundation for subsequent data populations.
To create an empty data frame using this method, we declare the data frame and assign empty vectors to each desired column. It’s crucial to specify the class types for each vector, and setting stringsAsFactors = FALSE
is often preferred to treat character vectors as strings.
Here’s how we can do it:
# Create an empty data frame with specified vectors
empty_df <- data.frame(
Doubles = double(), Integers = integer(), Factors = factor(), Logicals = logical(),
Characters = character(), stringsAsFactors = FALSE
)
This approach allows for explicit control over the structure of the data frame, defining the column names and data types.
Example: Creating an Empty Data Frame by Initializing a Set of Vectors
Now, let’s see an example of creating an empty data frame by initializing a set of vectors:
# Create an empty data frame with specified vectors
delftstack <- data.frame(
Doubles = double(), Integers = integer(), Factors = factor(), Logicals = logical(),
Characters = character(), stringsAsFactors = FALSE
)
# Display the structure of the data frame
print("Empty Data Frame Structure:")
str(delftstack)
In this example, we use the data.frame()
function to create an empty data frame named delftstack
.
We initialize vectors of different types (double
, integer
, factor
, logical
, and character
) within the function call, defining the structure of the data frame explicitly. The stringsAsFactors = FALSE
argument ensures that character vectors are treated as strings.
The subsequent print()
statement displays a message indicating that we are displaying the structure of the empty data frame. The str()
function reveals the detailed structure, including the variable names and their respective data types.
Code Output:
The output demonstrates the successful creation of an empty data frame with the specified structure. Each column is represented with its respective data type, indicating that the data frame is ready to be populated with data of the defined types.
Create an Empty Data Frame in R Using the structure()
Function
Another method for generating an empty data frame in R involves using the structure()
function.
The structure()
function in R is commonly used to set the class and attributes of an object. In the context of creating an empty data frame, we can leverage this function to specify the structure of the data frame directly.
The basic syntax of the structure()
function for creating an empty data frame is as follows:
# Create an empty data frame with specified structure
empty_df <- structure(list(), class = "data.frame")
Here, we use the list()
function to create an empty list, and then structure()
is employed to assign the “data.frame” class to this list, effectively creating an empty data frame.
Example: Creating an Empty Data Frame Using structure()
Now, let’s explore a working example of creating an empty data frame using the structure()
function:
# Create an empty data frame using structure()
empty_df <- structure(list(), class = "data.frame")
# Display the empty data frame
print("Empty Data Frame:")
print(empty_df)
In this example, the structure()
function is utilized to create an empty data frame named empty_df
. The list()
function inside structure()
generates an empty list, and by specifying the class as "data.frame"
, we essentially convert the list into a data frame.
The subsequent print()
statements are used to provide a message indicating that we are displaying an empty data frame and to display the content of the data frame.
Code Output:
The output confirms the successful creation of an empty data frame using the structure()
function. The data frame has zero columns and zero rows, aligning with the definition of an empty structure.
This method offers a concise way to initialize an empty data frame in R by explicitly specifying its class and structure using the structure()
function.
Create an Empty Data Frame in R Using the tibble()
Function From the tibble
Package
In R, the tibble
package provides an alternative to the base R data frame with the tibble()
function. Tibbles are enhanced data frames that offer some advantages over traditional data frames, such as improved printing and subsetting.
The tibble()
function, similar to data.frame()
, allows for the initialization of a data frame with enhanced features. The basic syntax of the tibble()
function for creating an empty data frame is as follows:
# Create an empty data frame using tibble()
empty_df <- tibble()
The tibble()
function is invoked without any arguments, resulting in the creation of an empty data frame.
Before using the tibble()
function, you need to install the tibble
package if you haven’t already:
# Install and load the tibble package
install.packages("tibble")
Example: Creating an Empty Data Frame Using tibble()
Now that the tibble
package is installed, we can use the tibble()
function to create an empty data frame.
# Load the tibble package
library(tibble)
# Create an empty data frame using tibble()
empty_df <- tibble()
# Display the empty data frame
print("Empty Data Frame:")
print(empty_df)
In this example, we first load the tibble
package using the library(tibble)
statement. Then, the tibble()
function is called to create an empty data frame named empty_df
.
Unlike base R functions, the tibble()
function is designed for modern data frames and comes with additional features.
The subsequent print()
statements are used to provide a message indicating that we are displaying an empty data frame and to display the content of the data frame.
Code Output:
The output confirms the successful creation of an empty data frame using the tibble()
function. The output format includes the term tibble
, indicating that it is a tibble data frame.
The dimensions are specified as 0 rows by 0 columns, aligning with the definition of an empty structure. This method offers a modern and concise way to initialize an empty data frame in R using the tibble()
function from the tibble
package.
Conclusion
Creating an empty data frame in R can be accomplished through various methods, each offering suitability for different use cases. We explored several approaches, including using the data.frame()
function, creating an empty matrix and converting it, initializing a set of vectors, using the structure()
function, and leveraging the tibble()
function from the tibble
package.
The data.frame()
function provides a straightforward way to create an empty data frame, while generating an empty matrix and converting it allows for additional customization. Initializing a set of vectors offers explicit control over column types, and the structure()
function provides a concise means of specifying the class and creating an empty data frame.
The tibble()
function from the tibble
package adds modern features to data frames and offers an alternative method for creating empty structures. Ultimately, the selection of a method depends on your preferences, desired level of control, and any additional features required.
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook