How to Get the Number of Columns in R
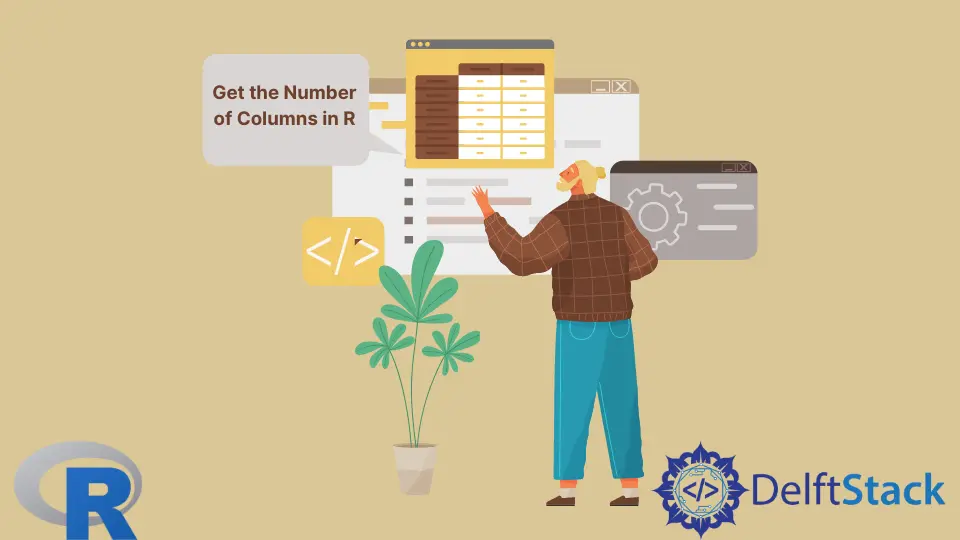
In the world of data analysis, understanding the structure of your data is crucial. When working with data frames in R, knowing how to get the number of columns can help you manage your data effectively.
This tutorial will guide you through various methods to retrieve the number of columns in R, making it easier to manipulate and analyze your datasets. Whether you’re a beginner or an experienced R user, this guide will provide you with the information you need to enhance your data analysis skills. Let’s dive into the different ways to get the number of columns in R!
Using the ncol()
Function
The simplest way to get the number of columns in a data frame is by using the ncol()
function. This built-in R function directly returns the number of columns present in a data frame or matrix.
Here’s how you can use it:
data_frame <- data.frame(Name = c("Alice", "Bob", "Charlie"), Age = c(25, 30, 35), City = c("New York", "Los Angeles", "Chicago"))
num_columns <- ncol(data_frame)
num_columns
Output:
3
The ncol()
function takes your data frame as an argument and returns the total number of columns. In the above example, we created a simple data frame with three columns: Name, Age, and City. By calling ncol(data_frame)
, we received an output of 3, indicating that there are three columns in our data frame.
Using the dim()
Function
Another way to find out the number of columns is by using the dim()
function. This function returns the dimensions of the data frame in a vector format, where the first element is the number of rows and the second element is the number of columns.
Here’s how to implement it:
data_frame <- data.frame(Name = c("Alice", "Bob", "Charlie"), Age = c(25, 30, 35), City = c("New York", "Los Angeles", "Chicago"))
dimensions <- dim(data_frame)
num_columns <- dimensions[2]
num_columns
Output:
3
In this example, we again created a data frame similar to the previous one. When we called dim(data_frame)
, it returned a vector containing the dimensions of the data frame. By accessing the second element of this vector (dimensions[2]
), we obtained the number of columns, which is 3. This method is particularly useful if you also want to know the number of rows in the data frame.
Using the colnames()
Function
If you prefer to work with column names, the colnames()
function can also be used to determine the number of columns in a data frame. By obtaining the column names and measuring their length, you can easily find out how many columns are present.
Here’s how you can do this:
data_frame <- data.frame(Name = c("Alice", "Bob", "Charlie"), Age = c(25, 30, 35), City = c("New York", "Los Angeles", "Chicago"))
column_names <- colnames(data_frame)
num_columns <- length(column_names)
num_columns
Output:
3
In this case, we again created a data frame with three columns. The colnames(data_frame)
function retrieves the names of the columns, and we then used length()
to count how many names were returned. The output, once more, is 3, confirming the number of columns in our data frame. This method is useful if you also want to inspect the actual names of the columns.
Conclusion
Understanding how to get the number of columns in R is a fundamental skill for anyone working with data frames. Whether you choose to use the ncol()
, dim()
, or colnames()
functions, each method provides a straightforward way to access this information. By mastering these techniques, you can enhance your data manipulation capabilities, making your analysis more efficient and effective. Keep practicing, and soon you’ll find yourself navigating R like a pro!
FAQ
-
How do I get the number of rows in R?
You can use the nrow() function to get the number of rows in a data frame. -
Can I find the number of columns in a matrix using similar methods?
Yes, the same functions like ncol(), dim(), and colnames() can be used to find the number of columns in a matrix. -
Is it possible to get the number of columns for a list in R?
No, lists do not have a fixed number of columns like data frames or matrices. You can use length() to get the number of elements in a list.
-
What if my data frame has missing values?
The methods mentioned will still return the number of columns regardless of missing values. -
Can I apply these methods to large datasets?
Absolutely! These functions are efficient and work well with large datasets in R.
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook