How to Create a Large Data Frame in R
- Use Vectors and Factors to Create a Data Frame in R
- Use List to Create a Data Frame in R
- Use Matrix to Create a Data Frame in R
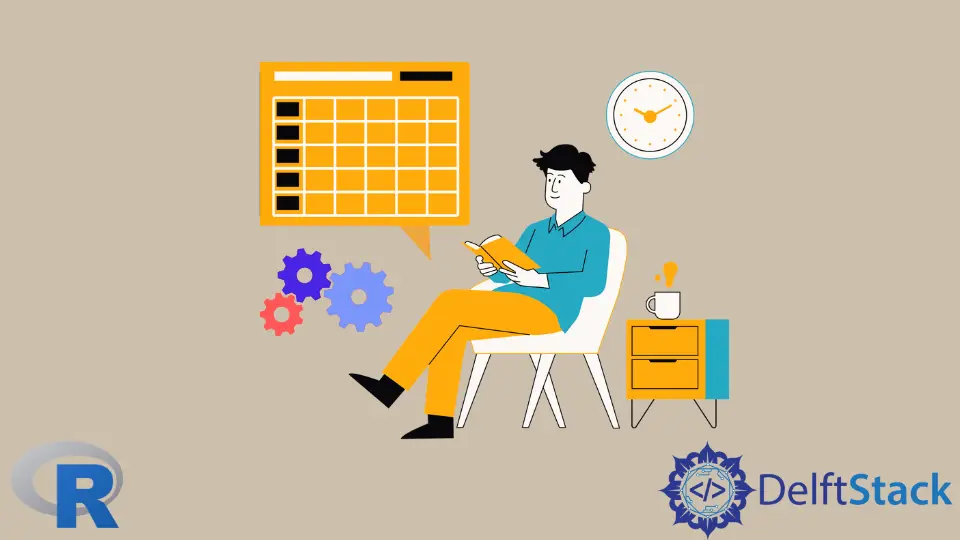
This article will explain several methods of creating a large data frame in R.
Use Vectors and Factors to Create a Data Frame in R
A data frame is the most powerful built-in data structure of the R language, and it resembles the tabular matrix, where each column has the same length, and they must have names. Underneath, though, it has more list
like features, as each column of the data frame is treated as if it was an element of the list
data structure. On the plus side, we can construct a data frame using the mix of vectors and factors as demonstrated in the following code snippet. We use the data.frame
function to build a data frame object from vectors and factors.
v1 <- c(1.1, 1.2, 1.3, 2.1, 2.2, 2.3)
v2 <- c(11, 12, 13, 21, 22, 23)
v3 <- c(1, 2, 3, 1, 2, 3)
wday <- factor(c("Wed", "Thu", "Mon", "Wed", "Thu", "Fri"))
df2 <- data.frame(v1, v2, v3, wday)
Output:
v1 v2 v3 wday
1 1.1 11 1 Wed
2 1.2 12 2 Thu
3 1.3 13 3 Mon
4 2.1 21 1 Wed
5 2.2 22 2 Thu
6 2.3 23 3 Fri
Use List to Create a Data Frame in R
Alternatively, a data frame object can be created using the list object. Although, this method requires a different function called as.data.frame
that converts a single list object to a data frame. Note that we construct the list object with the list
function in the following example and chain that call as the argument of the as.data.frame
. Since the data frame must have the column labels, they are usually chosen automatically if the user does not specify them. If the unwanted names are chosen during the conversion, one can always call the colnames
function and assign a vector of column names to it.
v1 <- c(1.1, 1.2, 1.3, 2.1, 2.2, 2.3)
v2 <- c(11, 12, 13, 21, 22, 23)
v3 <- c(1, 2, 3, 1, 2, 3)
wday <- factor(c("Wed", "Thu", "Mon", "Wed", "Thu", "Fri"))
df1 <- as.data.frame(list(v1, v2, v3, wday))
colnames(df1) <- c("v1", "v2", "v3", "wday")
df1
Output:
v1 v2 v3 wday
1 1.1 11 1 Wed
2 1.2 12 2 Thu
3 1.3 13 3 Mon
4 2.1 21 1 Wed
5 2.2 22 2 Thu
6 2.3 23 3 Fri
Use Matrix to Create a Data Frame in R
Sometimes, there needs to be a huge data frame allocation that is impractical to initialize with the vectors or lists. Fortunately, the matrix
function provides an easy and fast way to construct large data frames by specifying the row/column numbers. Optionally, one can initialize the values in the data frame using the vector passed as the first argument. Otherwise, the elements will have NA
values.
m1 <- matrix(1:1000, ncol = 10, nrow = 100)
df1 <- as.data.frame(m1)
df1
Output:
V1 V2 V3 V4 V5 V6 V7 V8 V9 V10
1 1 101 201 301 401 501 601 701 801 901
2 2 102 202 302 402 502 602 702 802 902
....
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook