R의 한 열에서 NA가 있는 행 제거
-
R에서
is.na()
메서드를 사용하여 한 열에서NA
가 있는 행 제거 -
R에서
complete.cases()
메서드를 사용하여 한 열에서NA
가 있는 행 제거 -
R에서 Tidyr 라이브러리
drop_na()
메서드를 사용하여 한 열에서NA
가 있는 행 제거
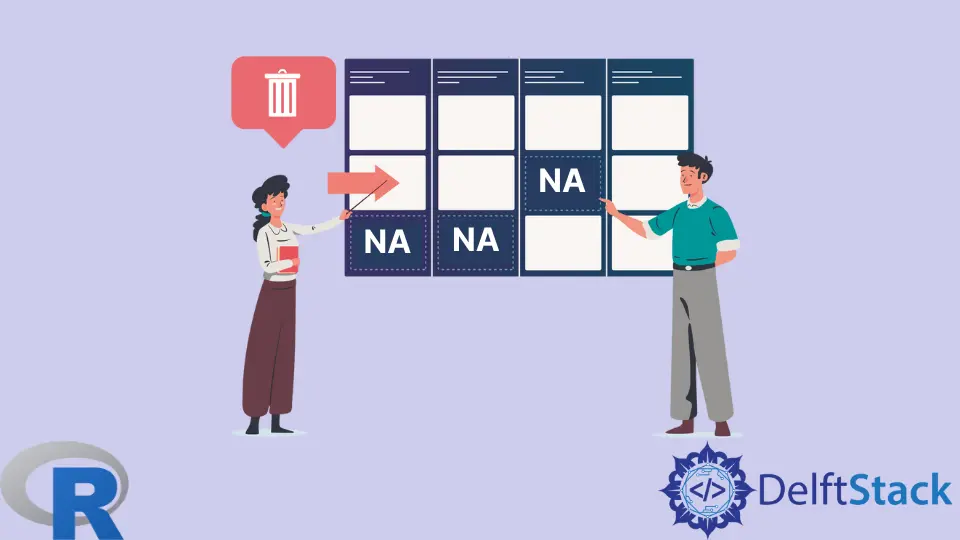
데이터 프레임의 열은 NA
키워드로 표시되는 빈 값을 가질 수 있습니다. 이 튜토리얼은 R의 한 열에서 NA
값을 포함하는 행을 제거하는 방법을 보여줍니다.
R에서 is.na()
메서드를 사용하여 한 열에서 NA
가 있는 행 제거
메소드 is.na()
는 데이터 프레임에서 NA
값을 찾고 NA
값의 행을 제거합니다. 프로세스는 다음과 같습니다.
- 먼저 데이터 프레임을 생성합니다.
- 삭제할
NA
값과 행을 기반으로 열을 선택합니다. dataframe$columnname
매개 변수를 우회하는is.na()
메서드를 사용하여 부정을 생성합니다.- 메서드는
NA
값이 포함된 경우 지정된 열을 확인하여 행을 제거합니다.
위의 단계에 따라 예를 들어 보겠습니다.
예:
Delftstack = data.frame(Name=c('Jack', 'John', 'Mike', 'Michelle', 'Jhonny'),
LastName=c('Danials', 'Cena', 'Chandler', 'McCool', 'Nitro'),
Id=c(101, 102, NA, 104, NA),
Designation=c('CEO', 'Project Manager', 'Senior Dev', 'Junior Dev', 'Intern'))
print('The dataframe before removing the rows:-')
print(Delftstack)
print('The dataframe after removing the rows:-')
Delftstack[!is.na(Delftstack$Id),]
위의 코드는 Id
열의 NA
값을 기반으로 행을 삭제합니다.
출력:
[1] "The dataframe before removing the rows:-"
Name LastName Id Designation
1 Jack Danials 101 CEO
2 John Cena 102 Project Manager
3 Mike Chandler NA Senior Dev
4 Michelle McCool 104 Junior Dev
5 Jhonny Nitro NA Intern
[1] "The dataframe after removing the rows:-"
Name LastName Id Designation
1 Jack Danials 101 CEO
2 John Cena 102 Project Manager
4 Michelle McCool 104 Junior Dev
R에서 complete.cases()
메서드를 사용하여 한 열에서 NA
가 있는 행 제거
complete.cases()
메서드는 is.na()
메서드와 유사하게 작동합니다. complete.cases
메서드는 데이터 프레임에서 NA
값을 찾고 이 값을 포함하는 행을 제거합니다.
프로세스는 위에서 설명한 단계와 유사하지만 complete.cases()
에 부정을 사용하지 않는다는 차이점만 있습니다.
예:
Delftstack = data.frame(Name=c('Jack', 'John', 'Mike', 'Michelle', 'Jhonny'),
LastName=c('Danials', 'Cena', 'Chandler', 'McCool', 'Nitro'),
Id=c(101, 102, NA, 104, NA),
Designation=c('CEO', 'Project Manager', 'Senior Dev', 'Junior Dev', 'Intern'))
print('The dataframe before removing the rows:-')
print(Delftstack)
print('The dataframe after removing the rows:-')
Delftstack[complete.cases(Delftstack$Id),]
위의 코드는 Id
열의 NA
값을 기반으로 행을 삭제합니다.
출력:
[1] "The dataframe before removing the rows:-"
Name LastName Id Designation
1 Jack Danials 101 CEO
2 John Cena 102 Project Manager
3 Mike Chandler NA Senior Dev
4 Michelle McCool 104 Junior Dev
5 Jhonny Nitro NA Intern
[1] "The dataframe after removing the rows:-"
Name LastName Id Designation
1 Jack Danials 101 CEO
2 John Cena 102 Project Manager
4 Michelle McCool 104 Junior Dev
R에서 Tidyr 라이브러리 drop_na()
메서드를 사용하여 한 열에서 NA
가 있는 행 제거
tidyr 라이브러리의 drop_na()
는 NA
값 열을 기반으로 행을 삭제합니다. 먼저 tidyr 라이브러리가 아직 설치되지 않은 경우 설치해야 합니다.
다음 코드를 실행하여 패키지를 설치합니다.
install.packages('tidyverse')
코드 출력은 위의 방법과 유사하지만 프로세스는 약간 다릅니다. dataframe %>% drop_na(column)
구문을 사용하여 행을 삭제합니다.
예:
library(tidyr)
Delftstack = data.frame(Name=c('Jack', 'John', 'Mike', 'Michelle', 'Jhonny'),
LastName=c('Danials', 'Cena', 'Chandler', 'McCool', 'Nitro'),
Id=c(101, 102, NA, 104, NA),
Designation=c('CEO', 'Project Manager', 'Senior Dev', 'Junior Dev', 'Intern'))
print('The dataframe before removing the rows:-')
print(Delftstack)
print('The dataframe after removing the rows:-')
Delftstack %>% drop_na(Id)
위의 코드는 위의 방법과 유사하게 작동합니다.
출력:
[1] "The dataframe before removing the rows:-"
Name LastName Id Designation
1 Jack Danials 101 CEO
2 John Cena 102 Project Manager
3 Mike Chandler NA Senior Dev
4 Michelle McCool 104 Junior Dev
5 Jhonny Nitro NA Intern
[1] "The dataframe after removing the rows:-"
Name LastName Id Designation
1 Jack Danials 101 CEO
2 John Cena 102 Project Manager
4 Michelle McCool 104 Junior Dev
na.omit()
, filter()
등과 같은 메소드도 있으며, 이는 모든 열에서 발견된 NA
값을 기반으로 행을 제거하는 데 사용됩니다. 하나의 열이 아닌 여러 열을 기준으로 값을 제거합니다.
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook