How to Fix ZeroDivisionError: Float Division by Zero in Python
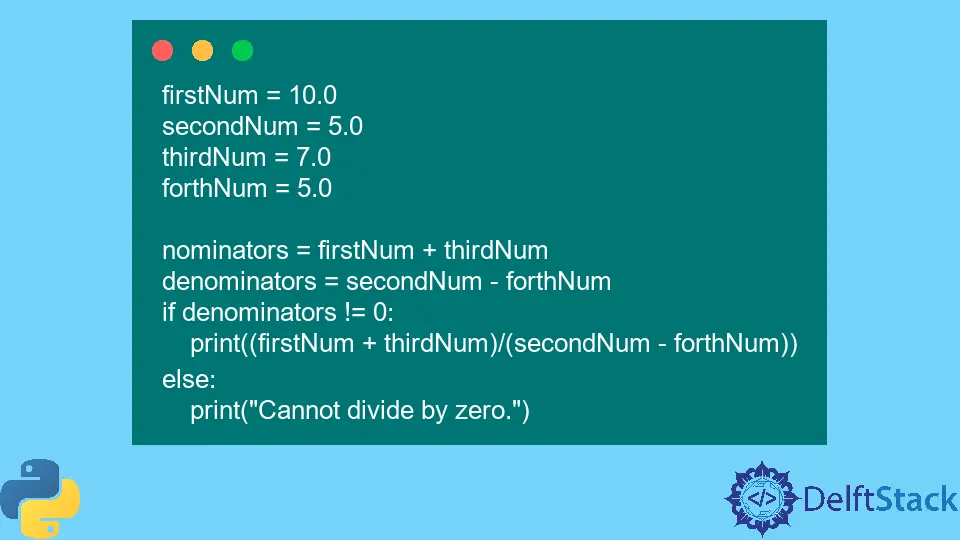
We will introduce why ZeroDivisionError
occurs and how we can easily resolve it with examples in Python.
ZeroDivisionError: Float Division by Zero
in Python
While working on mathematical equations or code that includes mathematical expressions based on results, it is a common error. In Python, a ZeroDivisionError
occurs when attempting to divide a number by zero.
In mathematics, it is impossible to divide any number by zero. Whenever there is a situation through our code, dividing a number by zero will throw an exception.
Let’s write a program to throw this exception using Python, as shown below.
firstNum = 10
secondNum = 5
thirdNum = 7
forthNum = 5
print((firstNum + thirdNum) / (secondNum - forthNum))
Output:
As we can see from the above example, the subtraction of the denominators resulted in 0
, which we got the error ZeroDivisionError
. Let’s test another example with float numbers instead, as shown below.
firstNum = 10.0
secondNum = 5.0
thirdNum = 7.0
forthNum = 5.0
print((firstNum + thirdNum) / (secondNum - forthNum))
We will get the following error message now.
As we can see from the above examples, whenever our result in the denominator is zero, it will crash our application and could also lose important data during an important program run.
It is important to deal with this kind of error properly, to protect our program from crashing and losing any important information.
There are two ways to resolve this issue, which we will discuss in detail. The first solution is to use an if-else
statement.
We will always ensure that to execute division, the difference of denominators is always bigger than zero. If the difference between both denominators equals zero, it will just output the message saying that we cannot divide by zero.
The code of the solution is shown below.
firstNum = 10.0
secondNum = 5.0
thirdNum = 7.0
forthNum = 5.0
nominators = firstNum + thirdNum
denominators = secondNum - forthNum
if denominators != 0:
print((firstNum + thirdNum) / (secondNum - forthNum))
else:
print("Cannot divide by zero.")
When we run this code, it will give the following output.
As we can see from the above example, instead of throwing an error, our code outputs the phrase we wanted it to output if the result of denominators results in 0
. Our second solution uses the try
and except
methods, as shown below.
firstNum = 10.0
secondNum = 5.0
thirdNum = 7.0
forthNum = 5.0
try:
print((firstNum + thirdNum) / (secondNum - forthNum))
except:
print("Cannot divide by zero.")
The code will try to run the expression; if it runs successfully, it will display the result; otherwise, it will display the phrase saying that we cannot divide by zero. The output of the following code is shown below.
It is very important to note that these solutions only handle the error when we know the source of the error is because the denominator is zero, but if you are not sure if the denominator could be zero, it is better to check it before doing the operation.
In conclusion, the ZeroDivisionError: float division by zero
is a common exception in Python that occurs when attempting to divide a float by zero.
This exception can be handled using an if
statement and a try-except
block. To avoid crashing or producing incorrect results, we must handle this exception in our code.
Rana is a computer science graduate passionate about helping people to build and diagnose scalable web application problems and problems developers face across the full-stack.
LinkedInRelated Article - Python Error
- Can Only Concatenate List (Not Int) to List in Python
- How to Fix Value Error Need More Than One Value to Unpack in Python
- How to Fix ValueError Arrays Must All Be the Same Length in Python
- Invalid Syntax in Python
- How to Fix the TypeError: Object of Type 'Int64' Is Not JSON Serializable
- How to Fix the TypeError: 'float' Object Cannot Be Interpreted as an Integer in Python