How to Write List to CSV in Python
-
Write List to CSV in Python Using the
csv.writer()
Method - Write List to CSV in Python Using the Quotes Method
-
Write List to CSV in Python Using the
pandas
Method -
Write List to CSV in Python Using the
NumPy
Method
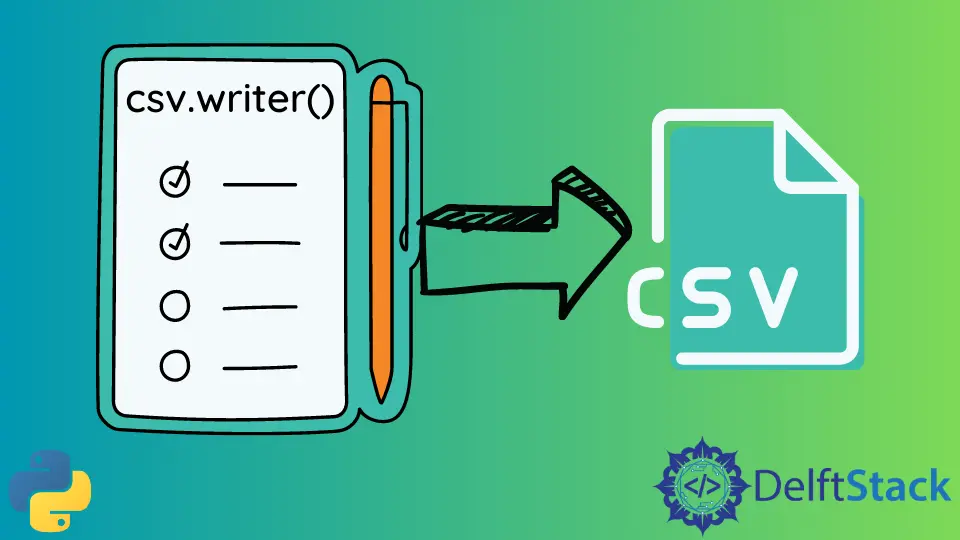
A CSV file can store the data in a tabular format. This data is a simple text; every row of data is called the record, and each one can be separated with commas.
This article will discuss the different methods to write a list to CSV in Python.
Write List to CSV in Python Using the csv.writer()
Method
Let’s first import the csv
module:
import csv
Suppose we take the following entries to write into a CSV file.
RollNo, Name, Subject
1, ABC, Economics
2, TUV, Arts
3, XYZ, Python
Now, the complete example code is as follows:
import csv
with open("students.csv", "w", newline="") as student_file:
writer = csv.writer(student_file)
writer.writerow(["RollNo", "Name", "Subject"])
writer.writerow([1, "ABC", "Economics"])
writer.writerow([2, "TUV", "Arts"])
writer.writerow([3, "XYZ", "Python"])
If you run the above codes, the students.csv
file will be created, row-wise, in the current directory with the entries that are present in the code. The csv.writer()
function will create the writer()
object, and the writer.writerow()
command will insert the entries row-wise into the CSV file.
Write List to CSV in Python Using the Quotes Method
In this method, we will see how we can write values in CSV file with quotes. The complete example code is as follows:
import csv
list = [["RN", "Name", "GRADES"], [1, "ABC", "A"], [2, "TUV", "B"], [3, "XYZ", "C"]]
with open("studentgrades.csv", "w", newline="") as file:
writer = csv.writer(file, quoting=csv.QUOTE_ALL, delimiter=";")
writer.writerows(list)
The studentgrades.csv
file will be created in the current directory. The csv.QUOTE_ALL()
function places double quotes on all the entries, using a delimiter ;
for separation.
Output:
RN;"Name";"GRADES"
1;"ABC";"A"
2;"TUV";"B"
3;"XYZ";"C"
Write List to CSV in Python Using the pandas
Method
This method uses the Pandas
library, which holds a complete DataFrame. If you do not have this library installed on your PC, you can use an online tool called Google Colab.
The complete example code is as follows:
import pandas as pd
name = ["ABC", "TUV", "XYZ", "PQR"]
degree = ["BBA", "MBA", "BSC", "MSC"]
score = [98, 90, 88, 95]
dict = {"name": name, "degree": degree, "score": score}
df = pd.DataFrame(dict)
df.to_csv("test.csv")
Output:
name degree score
0 ABC BBA 98
1 TUV MBA 90
2 XYZ BSC 88
3 PQR MSC 95
This method writes the Python list into a CSV file as a DataFrame.
Write List to CSV in Python Using the NumPy
Method
This method uses the NumPy
library to save a list to a CSV file in Python. The complete example code is as follows:
import numpy as np
list_rows = [
["ABC", "COE", "2", "9.0"],
["TUV", "COE", "2", "9.1"],
["XYZ", "IT", "2", "9.3"],
["PQR", "SE", "1", "9.5"],
]
np.savetxt("numpy_test.csv", list_rows, delimiter=",", fmt="% s")
The savetxt()
function of the NumPy
library will write a CSV file from the Python list.
Output:
ABC COE 2 9
TUV COE 2 9.1
XYZ IT 2 9.3
PQR SE 1 9.5
Related Article - Python CSV
- How to Import Multiple CSV Files Into Pandas and Concatenate Into One DataFrame
- How to Split CSV Into Multiple Files in Python
- How to Compare Two CSV Files and Print Differences Using Python
- How to Convert XLSX to CSV File in Python
- How to Write List to CSV Columns in Python
- How to Write to CSV Line by Line in Python
Related Article - Python List
- How to Convert a Dictionary to a List in Python
- How to Remove All the Occurrences of an Element From a List in Python
- How to Remove Duplicates From List in Python
- How to Get the Average of a List in Python
- What Is the Difference Between List Methods Append and Extend
- How to Convert a List to String in Python