How to Write JSON to a File in Python
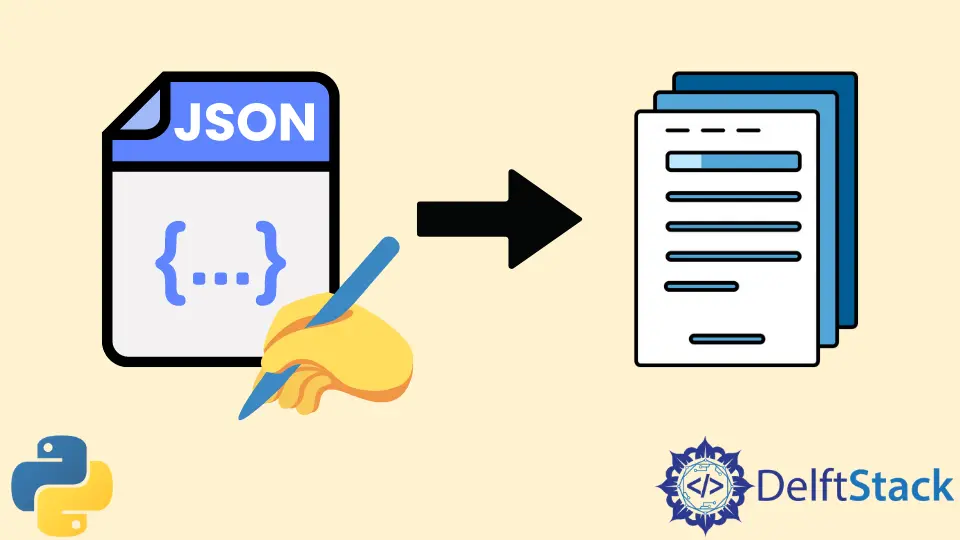
JSON is a lightweight and human-readable file format that is heavily used in the industry. JSON stands for JavaScript Object Notation
. As the name suggests, JSON is greatly popular among web developers and is heavily used in web applications to send and retrieve data from the server or other applications.
Python comes with many in-built packages or modules and has many external modules available over the internet for our use. Interestingly, it also has an in-built module, json
, to handle JSON data.
In Python, the JSON can be represented in two ways. First, as strings. A JSON string looks like this.
jsonString = '{ "name": "DelftStack", "email": "DelftStack@domain.com", "age": 20, "country": "Netherlands", "city": "Delft"}'
When representing JSON as a string, make sure that you only use double quotation (""
) to wrap keys and string values. JSON doesn’t support the single quotation and throws an error if the single quotation is used.
The second way is by using the Python object or popularly known as the Python dictionary. It has a similar syntax to that of a JSON.
The representation of the JSON in the form of a python dictionary would look like this.
jsonObject = {
"name": "DelftStack",
"email": "DelftStack@domain.com",
"age": 20,
"country": "Netherlands",
"city": "Delft",
}
Again, make sure you only use double quotation.
Write JSON to a File With the json
Module in Python
Suppose you have a variable that stores a JSON in the form of a string. So to write it in a JSON file, you can use the following code.
import json
fileName = "my-data.json"
jsonString = '{ "name": "DelftStack", "email": "DelftStack@domain.com", "age": 20, "country": "Netherlands", "city": "Delft"}'
jsonString = json.loads(jsonString)
file = open(fileName, "w")
json.dump(jsonString, file)
file.close()
First, we have imported the json
module. Then we have stored the JSON file name and the JSON string itself in two variables. Then we are creating and opening a new file with the name that we chose in the write
mode.
Then, we use the loads
function from the json
module to convert the JSON string to a python dictionary to write it to a file. The function accepts a valid JSON string and converts it to a Python dictionary.
Note that if an invalid string is provided to this function, it throws a
json.decoder.JSONDecodeError
error. So, make sure you pass in a correct string or maybe use atry-except-finally
block for error handling.
Next, we use the dump()
method that json
module provides us. This method accepts a python dictionary and a file descriptor as its parameters and writes the dictionary’s data to the file.
The dump()
method works if and only if the file is not opened in a binary format, that is, "wb"
and "rb"
won’t work and lead to a TypeError
.
Lastly, we close the file, and the program exits. A JSON file by the name you defined in the program will be created in the current directory.
If a file with the same name and same extension exists in the working directory, that file’s content will be overwritten.
Now, suppose you wish to write a Python object or a Python dictionary to a JSON file. In that case, refer to the following code snippet.
import json
fileName = "my-data.json"
jsonObject = {
"name": "DelftStack",
"email": "DelftStack@domain.com",
"age": 20,
"country": "Netherlands",
"city": "Delft",
}
file = open(fileName, "w")
json.dump(jsonObject, file)
file.close()
In this case, we already have the python object or dictionary, and the dump()
, as explained above, accepts a dictionary as a parameter and writes it to the file descriptor. So, we open a file in write mode, write the data to the file using the dump()
method, and close the file.