How to Calculate Variance in Python
-
Use the
variance()
Function of the Statistics Module to Calculate Variance in Python -
Use the
var()
Function of the NumPy Library to Calculate Variance in Python -
Use the
sum()
Function and List Comprehensions to Calculate Variance in Python
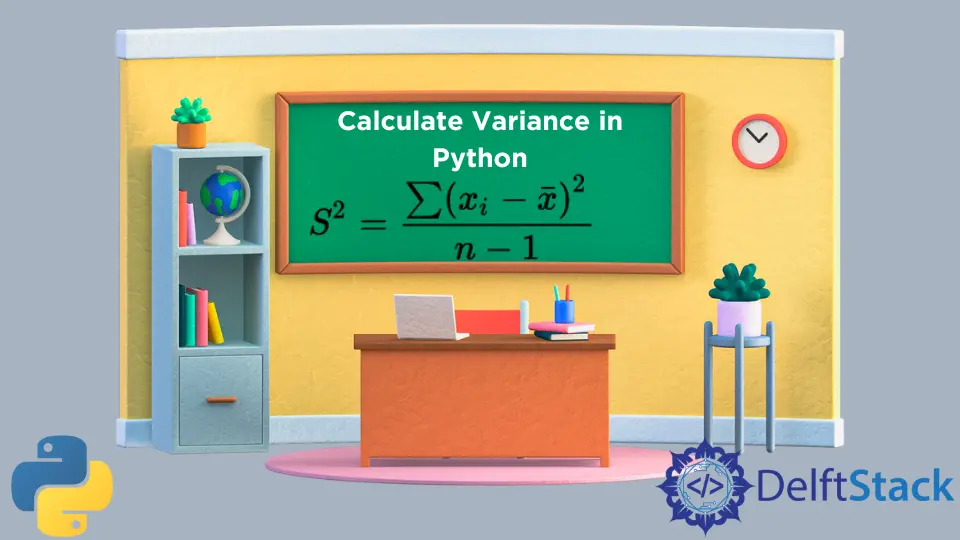
Variance is the measure of dispersion that considers all the data points spread across the data set. There are two measures of dispersion, variance and standard deviation (square root of variance).
In Python, several modules and libraries can help calculate the variance of a dataset or data points. This tutorial will discuss finding the variance in Python with examples provided to understand the methods better.
Use the variance()
Function of the Statistics Module to Calculate Variance in Python
The variance()
function is one of the functions of the Statistics module of Python. This module is used to provide functions to perform statistical operations like mean, median, standard deviation, etc., on numeric data.
The variance()
function of the Statistics module helps a user calculate the variance of the dataset or given data points.
import statistics
list = [12, 14, 10, 6, 23, 31]
print("List : " + str(list))
var = statistics.variance(list)
print("Variance of the given list: " + str(var))
Output:
List : [12, 14, 10, 6, 23, 31]
Variance of the given list: 86
In the above example, the str()
function converted the whole list and its standard deviation into a string because it can only be concatenated with a string.
Use the var()
Function of the NumPy Library to Calculate Variance in Python
The var()
function of the NumPy library can also calculate the variance of the elements in a given array list.
import numpy as np
arr = [12, 43, 24, 17, 32]
print("Array : ", arr)
print("Variance of array : ", np.var(arr))
Output:
Array : [12, 43, 24, 17, 32]
Variance of array : 121.04
Use the sum()
Function and List Comprehensions to Calculate Variance in Python
The sum()
function sums up all the elements of an iterable like a list, a tuple, etc.
On the other hand, list comprehension is a method that creates a list from the elements present in an already existing list.
The sum()
function and the list comprehension can help calculate the variance of a list.
list = [12, 43, 24, 17, 32]
average = sum(list) / len(list)
var = sum((x - average) ** 2 for x in list) / len(list)
print(var)
Output:
121.04
In the above example, the Math module is imported as it provides the sqrt()
function used to compute the square root of a given value.
Also, note that the function len()
is used. This function helped provide the length of the given list or the number of elements in the list.
The program above is based on the mathematical formula of variance.
Lakshay Kapoor is a final year B.Tech Computer Science student at Amity University Noida. He is familiar with programming languages and their real-world applications (Python/R/C++). Deeply interested in the area of Data Sciences and Machine Learning.
LinkedIn