How to Fix ValueError: Unsupported Pickle Protocol: 3 in Python
- Pickling and Unpickling in Python
-
Cause of the
ValueError: unsupported pickle protocol: 3
in Python -
Fix the
ValueError: unsupported pickle protocol: 3
in Python
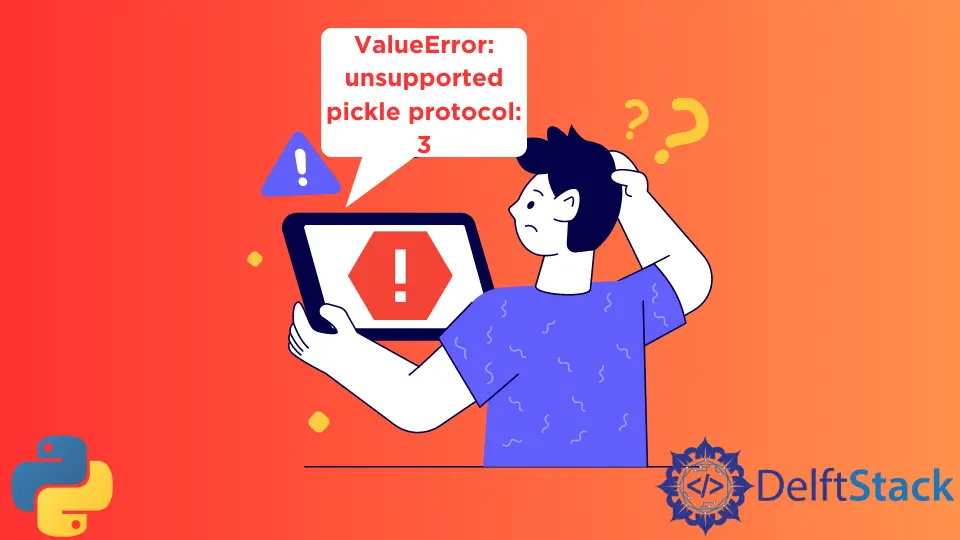
Every programming language encounters many errors. Some occur at the compile time, some at run time.
This article will discuss the ValueError: unsupported pickle protocol: 3
.
Pickling and Unpickling in Python
Pickling
is a method to turn a Python object (list
, dict
, etc.) into a character stream. This character stream is supposed to include all the data required to recreate the object in another Python script using a reverse process called Unpickling
.
The pickle
module does pickling and unpickling in Python.
In the following code, we have pickled and unpickled a list. We have created a list of names and opened the file using the wb
mode.
The w
will create a file if it is not already present, and the b
will write the data(list) into the file in a byte stream. We invoked the dump()
method specifying the list to be dumped and the file name in which to write the data in the form of bytes.
Similarly, we unpickled the data by first reading it from the file in rb
mode. Using the load()
method, we read the bytes from the file and converted them back to the list object.
Example Code:
# Python 3.x
import pickle
my_list = ["Jhon", "Alia", "Sam", "Chris"]
with open("my_file.txt", "wb") as f:
pickle.dump(my_list, f)
my_file = open("my_file.txt", "rb")
data = pickle.load(my_file)
print(data)
Output (Run on Anaconda’s Jupyter Notebook):
Cause of the ValueError: unsupported pickle protocol: 3
in Python
Sometimes in the process of unpickling, we face the ValueError: unsupported pickle protocol: 3
. This is due to the incompatible pickle protocols used during pickling and unpickling the data.
Python 3 introduced and used protocol 3 by default to pickle and unpickle the data if we do not specify the protocol. If we pickle and unpickle the data using different protocols, we will face this error, as shown in the code below.
Python 2 used protocol 0 by default, and Python 3 used protocol 3. So this error is mostly faced when we pickle and unpickle the data in different Python versions without correctly specifying the protocol version.
Example Code:
Python 3:
# Python 3.x
import pickle
my_list = ["Jhon", "Alia", "Sam", "Chris"]
with open("my_file.txt", "wb") as f:
pickle.dump(my_list, f)
Python 2:
# Python 2.x
import pickle
my_file = open("my_file.txt", "rb")
data = pickle.load(my_file)
print(data)
Output:
Fix the ValueError: unsupported pickle protocol: 3
in Python
To solve this error, we must specify the pickle protocol less than 3
when we dump the data using Python 3 to load this data in Python 2. Because Python 2 does not support protocols greater than 2.
Example Code:
# Python 3.x
import pickle
my_list = ["Jhon", "Alia", "Sam", "Chris"]
with open("my_file.txt", "wb") as f:
pickle.dump(my_list, f, protocol=2)
Example Code:
# Python 2.x
import pickle
my_file = open("my_file.txt", "rb")
data = pickle.load(my_file)
print(data)
Output:
Here, u
before strings represents Unicode.
I am Fariba Laiq from Pakistan. An android app developer, technical content writer, and coding instructor. Writing has always been one of my passions. I love to learn, implement and convey my knowledge to others.
LinkedInRelated Article - Python Error
- Can Only Concatenate List (Not Int) to List in Python
- How to Fix Value Error Need More Than One Value to Unpack in Python
- How to Fix ValueError Arrays Must All Be the Same Length in Python
- Invalid Syntax in Python
- How to Fix the TypeError: Object of Type 'Int64' Is Not JSON Serializable
- How to Fix the TypeError: 'float' Object Cannot Be Interpreted as an Integer in Python