How to Fix Valueerror: Expected 2d Array, Got 1d Array Instead
-
NumPy
Array in Python -
Create a
NumPy
Array in Python -
Cause of the
ValueError Expected 2D array, got 1D array instead
Error in Python -
Use Double Square Brackets With the Data to Fix
ValueError: Expected 2D Array, got 1D Array instead
in Python -
Reshape the Array Using
reshape()
to FixValueError: Expected 2D Array, got 1D Array instead
in Python - Conclusion
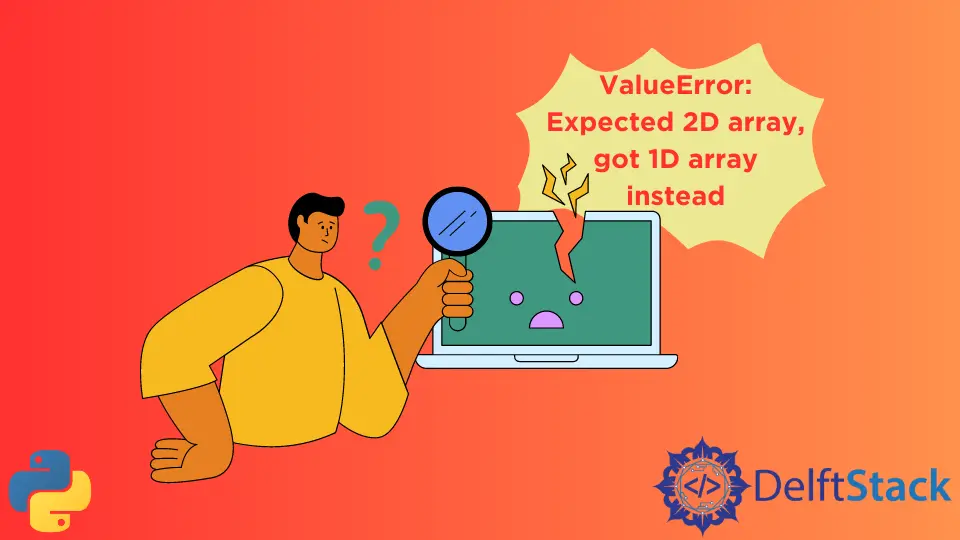
As we know, every programming language encounters many errors, some at runtime and others at compile time. Python sometimes encounters array errors when using the NumPy
library.
The ValueError: Expected 2D array, got 1D array instead
error occurs when we pass a 1-dimensional array instead of a 2-dimensional array in NumPy
.
NumPy
Array in Python
NumPy
is an open-source library that deals with arrays and mathematical operations. In Python, we have lists that provide us with the purpose of arrays, but the NumPy
creators claim that they prove 50x
faster arrays than lists.
It is one of the core purposes of using the NumPy
array.
Create a NumPy
Array in Python
The syntax of the NumPy
arrays is straightforward. We must import the NumPy
library to your program and use as accordingly.
Example Code:
import numpy as np
array1 = np.array([2, 4, 6])
print(array1)
In this code, we use the NumPy
library in Python to create an array named array1
containing the elements [2, 4, 6]
. By importing NumPy
as np
, we leverage its array creation functionality to quickly generate the array.
The print(array1)
statement is employed to display the contents of the array.
Output:
[2 4 6]
The output simply shows the array elements, providing a clear representation of the numeric values within the array: [2 4 6]
.
Cause of the ValueError Expected 2D array, got 1D array instead
Error in Python
This error occurs when we pass a 1-dimensional array in a function. However, the function requires a 2-dimensional array, so instead of giving a 2D array, we passed an Array with a Single Dimension.
It mostly happens using a machine learning algorithm in the predict()
method. Now, let’s take a look at the following scenario.
Example Code:
import numpy as np
from sklearn import svm
X = np.array([[2, 1], [4, 5], [2.6, 3.5], [6, 6], [0.8, 1], [7, 10]])
y = [1, 0, 1, 0, 1, 0]
classifier = svm.SVC(kernel="linear", C=1.0)
classifier.fit(X, y)
print(classifier.predict([0.7, 1.10]))
In this code, we utilize both the NumPy
and scikit-learn
libraries in Python for machine learning tasks. We create a two-dimensional NumPy
array named X
to represent input features and a corresponding list y
for target labels.
Subsequently, we instantiate a Support Vector Machine (SVM) classifier using scikit-learn
’s svm.SVC
with a linear kernel and regularization parameter C
set to 1.0
. The classifier is trained on the provided data using the fit()
method.
Finally, we make a prediction using the predict()
method with the input [0.7, 1.10]
.
Output:
ValueError: Expected 2D array, got 1D array instead:
array=[0.7 1.1].
Reshape your data either using array.reshape(-1, 1) if your data has a single feature or array.reshape(1, -1) if it contains a single sample.
The output is a ValueError
accompanied by a message stating, "Expected 2D array, got 1D array instead"
. This error occurs during the execution of the print(classifier.predict([0.7, 1.10]))
line.
The predict()
method of the SVM classifier expects a 2D array as input, but a 1D array is provided instead. The input [0.7, 1.10]
is a one-dimensional array, and the SVM model requires a two-dimensional array to make predictions.
In order to address this, the input should be reshaped into a 2D array. Let’s discuss it in the next section.
Use Double Square Brackets With the Data to Fix ValueError: Expected 2D Array, got 1D Array instead
in Python
Below we have resolved the error in the previous example. The simplest way to fix the error is to convert a dimensional array to a 2-dimensional array.
We can enclose [0.7,1.10]
in another square bracket to convert it to a 2D array when passing it to the predict()
method.
Example Code:
import numpy as np
from sklearn import svm
X = np.array([[2, 1], [4, 5], [2.6, 3.5], [6, 6], [0.8, 1], [7, 10]])
y = [1, 0, 1, 0, 1, 0]
classifier = svm.SVC(kernel="linear", C=1.0)
classifier.fit(X, y)
print(classifier.predict([[0.7, 1.10]]))
In this code, the NumPy
array X
represents input features, and the list y
contains corresponding target labels. We instantiate the SVM classifier with a linear kernel and a regularization parameter named C
set to 1.0
.
The classifier is trained on the provided data using the fit()
method. We then make a prediction using the predict()
method with the input [[0.7, 1.10]]
, which is a two-dimensional array representing a single data point.
Output:
[1]
The output of the prediction is [1]
, indicating the predicted class label.
Reshape the Array Using reshape()
to Fix ValueError: Expected 2D Array, got 1D Array instead
in Python
Another way to convert a 1D array to 2D is to reshape the array using the reshape()
method. We can reshape an array in Python using the reshape()
method.
The number of elements in each dimension determines the shape of an array. We can add or remove array dimensions using reshaping.
In the following code, we can see the dimensions of the NumPy
array before and after using the reshape()
method.
Example Code:
import numpy as np
from sklearn import svm
X = np.array([[2, 1], [4, 5], [2.6, 3.5], [6, 6], [0.8, 1], [7, 10]])
y = [1, 0, 1, 0, 1, 0]
classifier = svm.SVC(kernel="linear", C=1.0)
classifier.fit(X, y)
test = np.array([0.7, 1.10])
print("Dimension before:", test.ndim)
test = test.reshape(1, -1)
print("Dimension now:", test.ndim)
print("Classifier Result:", classifier.predict(test))
In this code, the training data, represented by the NumPy
array X
and corresponding target labels y
, is used to instantiate and train the SVM classifier and regularization parameter named C
set to 1.0
.
To test the classifier, we create a new array, test
, with values [0.7, 1.10]
. Before making predictions, we check the dimension of this array using the ndim
attribute and then reshape it into a two-dimensional array with the reshape()
method.
Finally, we feed the reshaped data into the trained SVM model to obtain a prediction.
Output:
Dimension before: 1
Dimension now: 2
Classifier Result: [1]
The output indicates the transformation of the input data for an SVM classifier.
Initially, the data has a dimension of 1
. After reshaping it to meet the classifier’s requirement of a 2D array, the dimension becomes 2
.
The final result of the classifier’s prediction for the reshaped data is [1]
.
Conclusion
In conclusion, encountering the ValueError: Expected 2D array, got 1D array instead
error in Python, particularly when using the NumPy
library, is a common issue. This error arises when a 1-dimensional array is passed instead of the required 2-dimensional array.
To resolve this issue, one can either use double square brackets with the data or reshape the array using the reshape()
method. Addressing this error is crucial, especially in scenarios like machine learning algorithms, where it often occurs in the predict()
method.
By understanding and applying these solutions, we can ensure proper handling of arrays in Python, preventing this specific error.
I am Fariba Laiq from Pakistan. An android app developer, technical content writer, and coding instructor. Writing has always been one of my passions. I love to learn, implement and convey my knowledge to others.
LinkedInRelated Article - Python Error
- Can Only Concatenate List (Not Int) to List in Python
- How to Fix Value Error Need More Than One Value to Unpack in Python
- How to Fix ValueError Arrays Must All Be the Same Length in Python
- Invalid Syntax in Python
- How to Fix the TypeError: Object of Type 'Int64' Is Not JSON Serializable
- How to Fix the TypeError: 'float' Object Cannot Be Interpreted as an Integer in Python