How to Fix ValueError: Cannot Convert Float NaN to Integer in Python
-
Use the
fillna()
Method to FixValueError: cannot convert float NaN to integer
in Python -
Use the
dropna()
Method to FixValueError: cannot convert float NaN to integer
in Python -
Use the
numpy.nan_to_num()
Function to FixValueError: cannot convert float NaN to integer
in Python
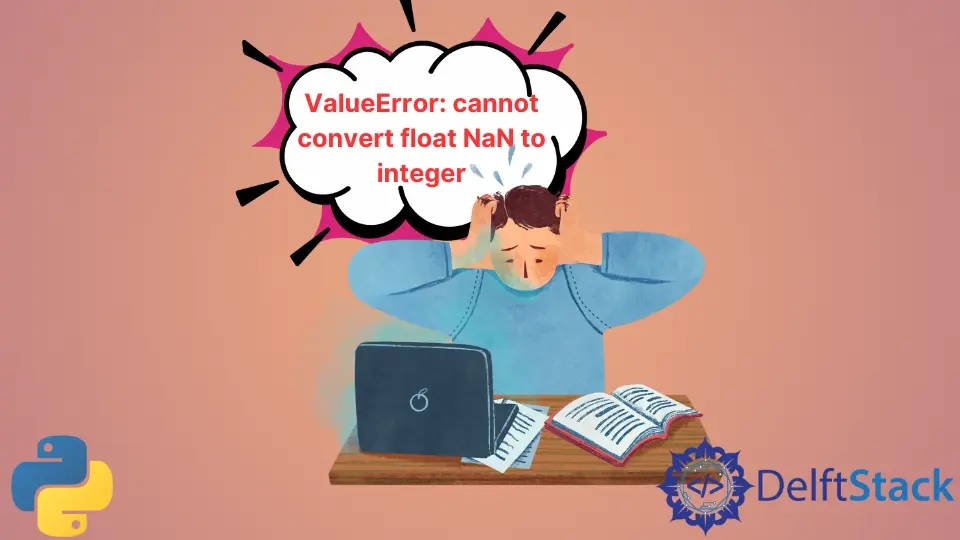
The ValueError
is raised when you give a valid argument to a function, but it is an invalid value. For example, you will get the ValueError
when you provide a negative number to the sqrt()
function of a math
module.
In Python, NaN
means Not a Number
, which indicates the missing entries in the dataset. It is a special float value that cannot be converted to other types than float.
The ValueError: cannot convert float NaN to integer
occurs when you try to convert a NaN
value to an integer type.
This tutorial will teach you to fix ValueError: cannot convert float NaN to integer
in Python.
Use the fillna()
Method to Fix ValueError: cannot convert float NaN to integer
in Python
We have used the following dataset to convert the price data to an integer type.
Here is an example of ValueError: cannot convert float NaN to integer
in Python.
# import pandas
import pandas
# read csv file
df = pandas.read_csv("itemprice.csv")
# convert to an integer type
df["price"] = df["price"].astype(int)
Output:
pandas.errors.IntCastingNaNError: Cannot convert non-finite values (NA or inf) to integer
There are two NaN
values in the dataset. You can solve this problem by replacing the NaN
values with 0
.
The fillna()
method replaces the NaN
values with a given value in Python. It checks NaN
values in the column and fills them with a specified value.
df_new = df.fillna(0)
print(df_new)
Output:
items price
0 A 540.0
1 B 370.0
2 C 0.0
3 D 220.0
4 E 0.0
Now, convert the float type to an integer type.
df_new["price"] = df_new["price"].astype(int)
Check the data type to verify.
print(df_new["price"].dtype)
Output:
int32
Use the dropna()
Method to Fix ValueError: cannot convert float NaN to integer
in Python
The dropna()
method removes all NULL
values in the DataFrame object.
The following example removes the rows that contain NULL
values in the dataset.
df_new = df.dropna()
print(df_new)
Output:
items price
0 A 540.0
1 B 370.0
3 D 220.0
Now, you can easily convert the data to an integer type.
df_new["price"] = df_new["price"].astype(int)
print(df_new["price"].dtype)
Output:
int32
Use the numpy.nan_to_num()
Function to Fix ValueError: cannot convert float NaN to integer
in Python
You can also solve this error using the numpy.nan_to_num()
function. It changes the NaN
value to 0.0
, which can be converted to an integer.
import numpy
dt = numpy.nan
print(dt)
Output:
nan
Use the numpy.nan_to_num()
function to replace the NaN
value with 0.0
.
dt = numpy.nan_to_num(dt)
print(dt)
Output:
0.0
Then convert it to an integer type.
dt = dt.astype(int)
print(dt.dtype)
Output:
int32
The ValueError: cannot convert float NaN to integer
is raised when you try to convert a NaN
value to an integer type. You can fix this problem by filling the NaN
values with a specified value or dropping the rows containing NaN
values.
Now you know how to solve ValueError: cannot convert float NaN to integer
in Python. We hope you find these solutions helpful.
Related Article - Python ValueError
Related Article - Python Error
- Can Only Concatenate List (Not Int) to List in Python
- How to Fix Value Error Need More Than One Value to Unpack in Python
- How to Fix ValueError Arrays Must All Be the Same Length in Python
- Invalid Syntax in Python
- How to Fix the TypeError: Object of Type 'Int64' Is Not JSON Serializable
- How to Fix the TypeError: 'float' Object Cannot Be Interpreted as an Integer in Python