How to Use del Keyword in Python
-
Delete Variables With the
del
Statement in Python -
Delete Lists With the
del
Statement in Python -
Delete Dictionary Element With the
del
Statement in Python -
Delete User-Defined Class Objects With the
del
Statement in Python
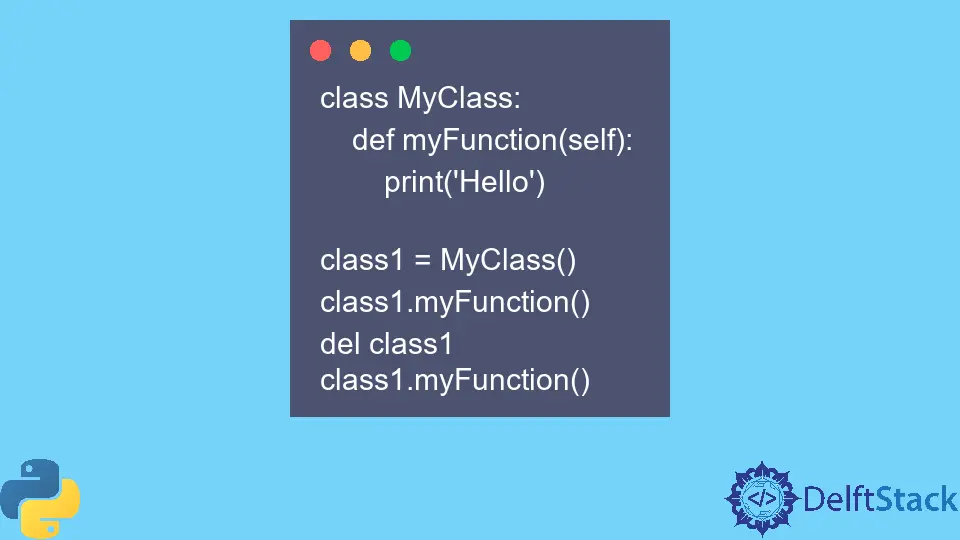
In this tutorial, we will discuss the usage of the del
statement in Python.
The del
statement is used to delete objects. Because of the object-oriented nature of Python, everything that can hold data is an object. So, the del
statement can be used to delete variables, class-objects, lists, etc.
The syntax of the del
statement is
del object_name
It works by removing the object_name
from both local and global namespace.
Delete Variables With the del
Statement in Python
variable = "This is a variable"
print(variable)
del variable
print(variable)
Output:
This is a variable
---------------------------------------------------------------------------
NameError Traceback (most recent call last)
<ipython-input-1-f9a4f1b9bb9c> in <module>()
2 print(variable)
3 del variable
----> 4 print(variable)
NameError: name 'variable' is not defined
The above program displayed the value of variable
and then gave us NameError
. This is because variable
has been removed from the namespace after using the del
statement.
Delete Lists With the del
Statement in Python
List = ["One", "Two", "Three"]
print(List)
del List
print(List)
Output:
['One', 'Two', 'Three']
---------------------------------------------------------------------------
NameError Traceback (most recent call last)
<ipython-input-2-edd546e00a8e> in <module>()
2 print(List)
3 del List
----> 4 print(List)
NameError: name 'List' is not defined
Similar to the previous example, the name List
has been removed from the namespace.
We can also slice a list using the del
statement.
List = ["One", "Two", "Three"]
print(List)
del List[1]
print(List)
Output:
['One', 'Two', 'Three']
['One', 'Three']
It deletes the list element with the index as 1.
Delete Dictionary Element With the del
Statement in Python
dictionary = {"key1": "value1", "key2": "value2", "key3": "value3"}
print(dictionary)
del dictionary["key2"]
print(dictionary)
Output:
{'key1': 'value1', 'key2': 'value2', 'key3': 'value3'}
{'key1': 'value1', 'key3': 'value3'}
Delete User-Defined Class Objects With the del
Statement in Python
class MyClass:
def myFunction(self):
print("Hello")
class1 = MyClass()
class1.myFunction()
del class1
class1.myFunction()
Output:
Hello
---------------------------------------------------------------------------
NameError Traceback (most recent call last)
<ipython-input-23-26401eda690e> in <module>()
6 class1.myFunction()
7 del class1
----> 8 class1.myFunction()
NameError: name 'class1' is not defined
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn