The switch Statement in Python
-
Implement the
switch
Statement Using the Dictionary -
Implement the
switch
Statement Using theif...elif...else
orif ... else
Statements
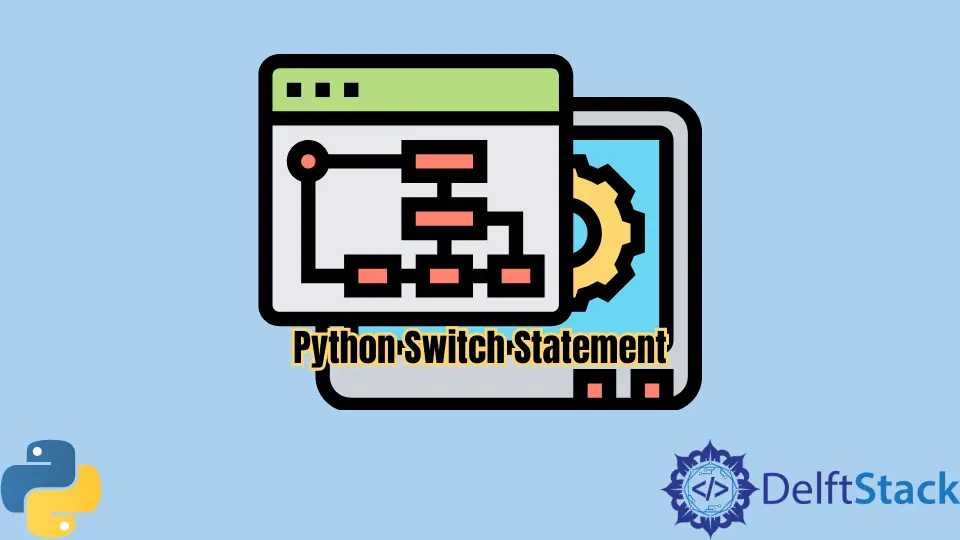
This tutorial will demonstrate various methods to implement the switch
statement functionality in Python. In many programming languages, the switch
statement is used to control the program flow or, in other words, which code blocks to execute based on the value of variable or expression.
Unlike the programming languages like C, C++, C#, and JAVA, the Python language does not provide the switch statement. But we can use the following methods in place of the switch statement in Python.
Implement the switch
Statement Using the Dictionary
The dictionary data type in Python is used to store the collection of data as key:value
pairs. It is a changeable or mutable data type and does not allow duplicate values.
Like in the switch statement, we decide which code to run based on the value of the variable. We can use the key:value
pairs of the dictionary, where the key
will be the value of the variable, and the value
will be the name of the function. We can execute the corresponding function based on the key
value.
Suppose we have a variable x
which value decides the code’s execution, we can save the key:value
pair in the dictionary like 0:function1
, 1:function2
, etc., where 0
and 1
is the value of the dictionary. To prevent the code from running into an error, we can use the dict.get(key, default)
method and pass the default
value for the function name, if some unknown value of x
is provided.
The below example code demonstrates how to use the dictionary data type to implement the switch
statement functionality in Python:
def function1():
print("function 1 was executed")
def function2():
print("function 2 was executed")
def function3():
print("function 3 was executed")
switch = {1: function1, 2: function2, 3: function3}
x = 1
switch.get(x, function1)()
x = 2
switch.get(x, function1)()
Output:
function 1 was executed
function 2 was executed
In case we want to perform some actions on the value of another variable a
based on the value of variable x
, we can do so as shown in the below example code:
switch = {1: lambda a: a + 1, 2: lambda a: a + 2, 3: lambda a: a + 3}
x = 2
a = 10
print(switch[x](a))
x = 1
a = 4
print(switch[x](a))
Output:
12
5
Implement the switch
Statement Using the if...elif...else
or if ... else
Statements
The if...elif...else
statement is used if we need to handle more than two cases, and the if ... else
statement is used to handle two possibilities or cases.
We can use if ... else
if we have two possible codes for the value of x
instead of the switch
statement. And we can use if...elif...else
statement if we have more than two codes or functions to execute for the multiple values of x
.
The below example code demonstrates how to implement the switch
statement functionality using the if ... else
statement in Python:
def function1():
print("function 1 was executed")
def function2():
print("function 2 was executed")
x = 3
if x == 1:
function1()
else:
function2()
Output:
function 2 was executed
We can implement the switch
statement using the if...elif...else
statement to handle multiple cases, as shown in the below example code:
def function1():
print("function 1 was executed")
def function2():
print("function 2 was executed")
def function3():
print("function 3 was executed")
x = 2
if x == 1:
function1()
elif x == 2:
function2()
elif x == 3:
function3()
Output:
function 2 was executed