The self Keyword in Python
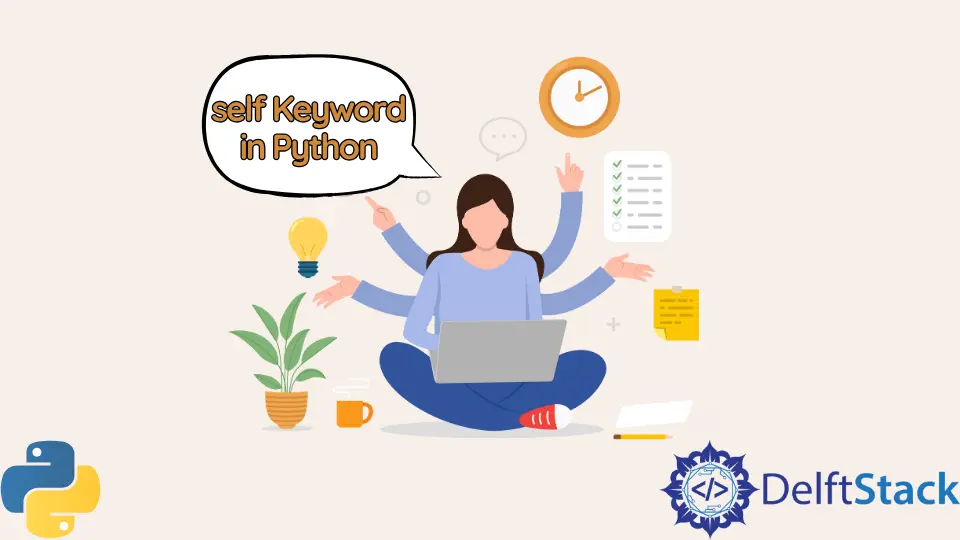
This tutorial will explain the purpose and use of the self
keyword in Python. In object-oriented programming, we have classes, and each class has various attributes and methods. Whenever an object or instance of a class is created, the constructor (__init__()
method in Python) is called to initialize the instance’s attributes.
While creating an instance of a class, we need to differentiate or specify the instance attributes from the arguments and other variables. And that’s where we need the self
keyword to specify that we are passing the value to the instance attributes and not to the local variable or argument with the same name.
Suppose, for a class car
we pass argument color
to the __init__()
method to initialize the color
attribute of the car
class, we will specify the color
attribute of the instance as self.color
, which shows that the class car
has an attribute color
and we assign the value to that attribute.
Similarly, we can also call the class’s instance methods using the self
keyword like self.start()
method of the car
class. So in short we can say that the self
keyword allows the user to specify and access the attributes and methods of an instance of the class.
Use and Example Codes of the self
Keyword in Python
Now, let us look into how the self
keyword is used in Python to specify an instance’s methods and attributes through the example code.
The first example code given below demonstrates how the self
keyword is used in the __init__()
method to initialize the attributes of the instance being initialized. We do not need to declare or create variables before initializing or assigning values to them so that we can initialize the instance attributes in the following way:
class car:
def __init__(self, model, color):
self.model = model
self.color = color
mycar = car("model XYZ", "red")
print(mycar.model)
print(mycar.color)
Output:
model XYZ
red
As can be seen above, the __init__()
method is called automatically on creating a new instance of the class, and its attributes get initialized. The self
needs to be the method’s parameter to access the instances’ methods and attributes.
The below example code demonstrates how to use the self
keyword to call the instance/class methods within other class methods in Python.
class car:
def __init__(self, model, color):
self.model = model
self.color = color
self.start()
def start(self):
print(self.model + ", has started!")
mycar = car("model XYZ", "red")
Output:
model XYZ, has started!
As in the above example code, we can call class methods using the self
keyword and access the attributes in any class method by passing the instance of the class as self
parameter.