The with Statement in Python
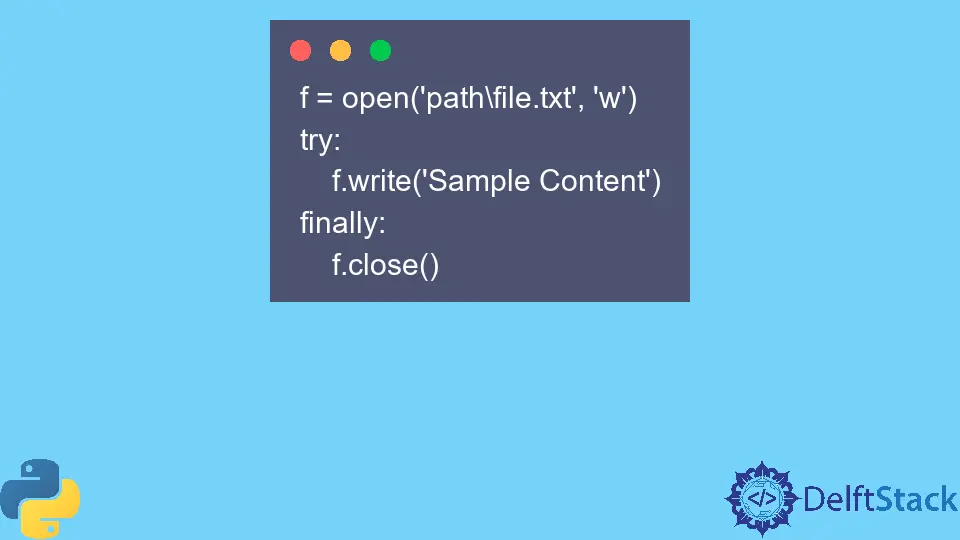
Python provides a variety of features and options to improve our existing code. One such feature is the with
keyword.
This tutorial will demonstrate the function of the with
statement and its applications in Python.
Use the with
Statement in Python
This statement is essentially used to help handle exceptions and clear up resources as they are used. It ensures that the code is executed properly and the resources are cleaned up afterward.
Mostly, you will find the use of the with
statement in file handling. Let us discuss an example.
f = open("path\file.txt", "w")
f.write("Sample Content")
f.close()
In the above example, we have opened a file using the open()
function and wrote some content using the write()
method. Then, the close()
function closed the file object and cleared up the resources.
Let’s see how the above code looks with the with
keyword.
with open("path\file.txt") as f:
f.write("Sample Content")
In the code, we can see that, using the with
statement, we were able to eliminate the use of the close()
function.
Another instance where the with
statement can be helpful is in encapsulating the try
and finally
blocks.
For example,
f = open("path\file.txt", "w")
try:
f.write("Sample Content")
finally:
f.close()
The try
block takes a code like opening a file, and the code in the finally
block is executed regardless if the code in the try
block raises an exception. In the above example, the code in the finally
block will execute and run the close()
function, even if the write()
function raises an exception.
We have seen how we can achieve this using the with
statement in the earlier example.
We can also use the with
statement with user-defined objects, and such objects are known as context managers. They have an __enter__
and __exit__
method defined.
The __enter__
method executes while creating an object of this class using the with
statement. The __exit__
method executes when the code in the with
block finishes.
class writer_class(object):
def __init__(self, filename):
self.filename = filename
def __enter__(self):
self.f = open(self.filename, "w")
return self.f
def __exit__(self, exception, value):
self.f.close()
with writer_class("file.txt") as f:
f.write("DelftStack")
The objects of the above class in Python execute the __enter__
method when created, which is used to open the file. The close()
function is put inside the __exit__
method to close the file object when the code has been executed.
Like classes, we can use the contextlib
library to create context manager methods. These methods can be made using the @contextmanager
decorator, which is put before the defined function.
For example,
from contextlib import contextmanager
@contextmanager
def new_open(file):
try:
f = open(file, "w")
yield f
finally:
f.close()
with new_open("sample.txt") as f:
f.write("DelftStack")
In the example code, we can see the use of the @contextmanager
decorator that allows us to create such methods. In the defined function, we put the complete code for creating the file object and closing the created object using the try
and finally
blocks.
Conclusion
To conclude, we discussed the with
statement in Python and its uses. We first discussed the basics of this statement and how it improves code readability and efficiency by cleaning up the resources.
We focused on its most common application with file handling to demonstrate its use. We also discussed context manager and its methods and how we can use the with
statement with them.
Manav is a IT Professional who has a lot of experience as a core developer in many live projects. He is an avid learner who enjoys learning new things and sharing his findings whenever possible.
LinkedIn