How to Use the pass Statement in Python
- Understanding the pass Statement
- Using pass in Functions
- Using pass in Classes
- Using pass in Control Flow Structures
- Conclusion
- FAQ
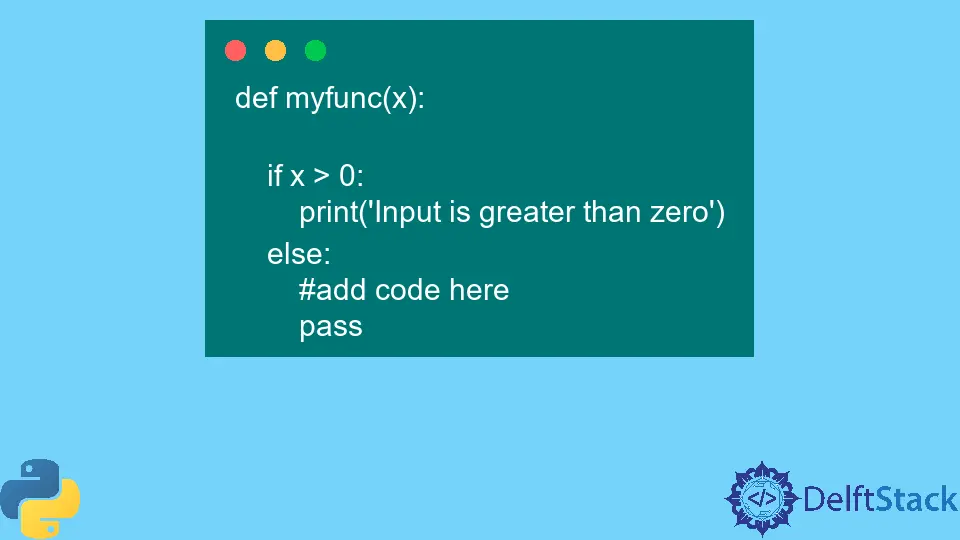
In the world of Python programming, the pass
statement is a simple yet powerful tool that often goes unnoticed by beginners. It’s a placeholder that allows you to write syntactically correct code while you plan out your logic. Whether you’re defining a function, a class, or a control flow structure, the pass
statement ensures that your code runs without errors, even when you haven’t implemented any functionality yet.
This tutorial will delve into the various ways you can use the pass
statement in Python, complete with practical examples to solidify your understanding. By the end, you’ll see how this seemingly trivial statement can enhance your coding workflow.
Understanding the pass Statement
The pass
statement in Python serves as a no-operation command. It does nothing when executed and is often used as a placeholder in your code. This can be particularly useful during the development phase, where you might want to outline the structure of your code without filling in all the details immediately. By using pass
, you can maintain the flow of your program while keeping it syntactically correct.
For example, if you are defining a function but haven’t decided on its implementation yet, you can use pass
to avoid syntax errors. Here’s a simple illustration:
def my_function():
pass
In this example, my_function
is defined but contains no executable code. The pass
statement allows you to define the function without causing an error. This is particularly useful when you’re sketching out your code structure and want to keep your options open for further development.
Using pass in Functions
One of the most common scenarios for using the pass
statement is within function definitions. When you’re in the early stages of writing a program, you may want to outline several functions without implementing their logic right away. This way, you can focus on the overall design of your program.
Consider the following example where we define multiple functions that are yet to be implemented:
def function_one():
pass
def function_two():
pass
def function_three():
pass
In this case, function_one
, function_two
, and function_three
are placeholders. This approach allows you to maintain a clean code structure while you think through the details of each function. Later, you can fill in the logic for each function as your project evolves. This method keeps your code organized and avoids the need to comment out incomplete functions, making it easier to read and maintain.
Using pass in Classes
Another common application of the pass
statement is within class definitions. Just like functions, you may want to define classes without implementing their methods immediately. This is particularly useful when you’re designing a complex system where the class structure is crucial.
Here’s an example of how you might use pass
in a class definition:
class MyClass:
pass
In this example, MyClass
is defined but has no attributes or methods. This allows you to establish a class structure as part of your program’s blueprint. You can later add attributes and methods as needed. This is especially beneficial in object-oriented programming, where class hierarchies can be complex and require careful planning.
Using pass in Control Flow Structures
The pass
statement is also applicable in control flow structures like loops and conditionals. When you want to create a loop or conditional statement but haven’t decided on the specific actions to take, pass
can help you maintain the structure without causing syntax errors.
For instance, consider the following example with an if
statement:
if condition:
pass
else:
print("Condition not met.")
Output:
Condition not met.
In this case, if condition
evaluates to True
, nothing happens because of the pass
statement. However, if it evaluates to False
, the program prints “Condition not met.” This allows you to outline your control flow while leaving some branches unimplemented for the time being. It keeps your code functional and allows for future expansion.
Conclusion
The pass
statement is a valuable tool in Python programming that allows you to write clean, organized code without committing to specific implementations. Whether you’re defining functions, classes, or control flow structures, pass
enables you to maintain syntactical correctness while planning your code. By using pass
, you can focus on the architecture of your program and fill in the details later, which is especially beneficial during the initial stages of development. Embracing this simple statement can enhance your coding efficiency and make your programming process smoother.
FAQ
-
What does the pass statement do in Python?
Thepass
statement serves as a placeholder in your code, allowing you to write syntactically correct code without implementing any functionality. -
Can I use pass in loops?
Yes, you can usepass
in loops to indicate that no action should be taken for certain iterations while maintaining the loop structure.
-
Is the pass statement necessary?
While it’s not strictly necessary, it helps maintain code organization and prevents syntax errors when you want to leave sections of your code unimplemented. -
Can I use pass in class methods?
Absolutely. You can usepass
in class methods to define the method structure without implementing any functionality immediately. -
How does pass improve my coding workflow?
Thepass
statement allows you to outline your code structure, keeping your code clean and organized while you plan the details of your implementation.