Python에서 del 키워드 사용
Muhammad Maisam Abbas
2023년1월30일
Python
Python Keyword
-
Python에서
del
문을 사용하여 변수 삭제 -
Python에서
del
문을 사용하여 목록 삭제 -
Python에서
del
문을 사용하여 사전 요소 삭제 -
Python에서
del
문을 사용하여 사용자 정의 클래스 개체 삭제
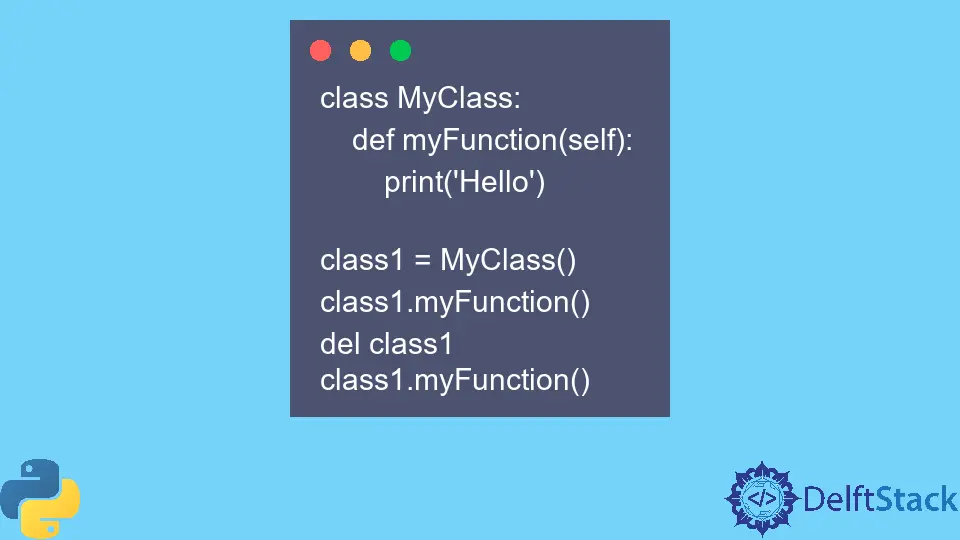
이 튜토리얼에서는 파이썬에서 del
문의 사용법에 대해 설명합니다.
del
문은 개체를 삭제하는 데 사용됩니다. Python의 객체 지향 특성 때문에 데이터를 보유 할 수있는 모든 것이 객체입니다. 따라서del
문을 사용하여 변수, 클래스 개체, 목록 등을 삭제할 수 있습니다.
del
문의 구문은 다음과 같습니다.
del object_name
로컬 및 글로벌 네임 스페이스 모두에서object_name
을 제거하여 작동합니다.
Python에서del
문을 사용하여 변수 삭제
variable = "This is a variable"
print(variable)
del variable
print(variable)
출력:
This is a variable
---------------------------------------------------------------------------
NameError Traceback (most recent call last)
<ipython-input-1-f9a4f1b9bb9c> in <module>()
2 print(variable)
3 del variable
----> 4 print(variable)
NameError: name 'variable' is not defined
위의 프로그램은variable
의 값을 표시 한 다음NameError
를 제공했습니다. 이는del
문을 사용한 후variable
이 네임 스페이스에서 제거 되었기 때문입니다.
Python에서del
문을 사용하여 목록 삭제
List = ["One", "Two", "Three"]
print(List)
del List
print(List)
출력:
['One', 'Two', 'Three']
---------------------------------------------------------------------------
NameError Traceback (most recent call last)
<ipython-input-2-edd546e00a8e> in <module>()
2 print(List)
3 del List
----> 4 print(List)
NameError: name 'List' is not defined
이전 예와 유사하게List
이름이 네임 스페이스에서 제거되었습니다.
del
문을 사용하여 목록을 분할 할 수도 있습니다.
List = ["One", "Two", "Three"]
print(List)
del List[1]
print(List)
출력:
['One', 'Two', 'Three']
['One', 'Three']
인덱스가 1 인 목록 요소를 삭제합니다.
Python에서del
문을 사용하여 사전 요소 삭제
dictionary = {"key1": "value1", "key2": "value2", "key3": "value3"}
print(dictionary)
del dictionary["key2"]
print(dictionary)
출력:
{'key1': 'value1', 'key2': 'value2', 'key3': 'value3'}
{'key1': 'value1', 'key3': 'value3'}
Python에서del
문을 사용하여 사용자 정의 클래스 개체 삭제
class MyClass:
def myFunction(self):
print("Hello")
class1 = MyClass()
class1.myFunction()
del class1
class1.myFunction()
출력:
Hello
---------------------------------------------------------------------------
NameError Traceback (most recent call last)
<ipython-input-23-26401eda690e> in <module>()
6 class1.myFunction()
7 del class1
----> 8 class1.myFunction()
NameError: name 'class1' is not defined
튜토리얼이 마음에 드시나요? DelftStack을 구독하세요 YouTube에서 저희가 더 많은 고품질 비디오 가이드를 제작할 수 있도록 지원해주세요. 구독하다
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn